commit message
This commit is contained in:
commit
3fbc2483b3
|
@ -0,0 +1,47 @@
|
|||
<?php
|
||||
|
||||
ini_set('display_errors', 'on');
|
||||
include('./consql.php');
|
||||
include_once('config.php');
|
||||
$StatServer = "StatServer_20";
|
||||
|
||||
$queryP = "SELECT date, numplayers FROM ".$StatServer." ORDER BY id DESC LIMIT 80";
|
||||
$arrPlay = $con->query($queryP);
|
||||
|
||||
?>
|
||||
|
||||
<!doctype html>
|
||||
<head>
|
||||
<!--<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.0.3/jquery.min.js"></script>-->
|
||||
<script src="https://code.jquery.com/jquery-3.3.1.js"></script>
|
||||
<script src="//cdnjs.cloudflare.com/ajax/libs/raphael/2.1.2/raphael-min.js"></script>
|
||||
<script src="morris/morris.js"></script>
|
||||
<!--link rel="stylesheet" href="lib/example.css"-->
|
||||
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/prettify/r224/prettify.min.css">
|
||||
<link rel="stylesheet" href="morris/morris.css">
|
||||
</head>
|
||||
<body>
|
||||
|
||||
|
||||
<h3>☠ Players on <?php echo $namemap ;?> (-ToX-)</h3>
|
||||
<div id="graph32"></div>
|
||||
|
||||
<script>
|
||||
var day_data = [<?php while($row = mysqli_fetch_assoc($arrPlay)){ echo "{'Date': '".$row["date"]."', 'Players': ".$row["numplayers"]."}, "; } ?> ];
|
||||
|
||||
Morris.Line({
|
||||
element: 'graph32',
|
||||
data: day_data,
|
||||
xkey: 'Date',
|
||||
ykeys: ['Players'],
|
||||
labels: ['Players on <?php echo $namemap ;?>'],
|
||||
yLabelFormat: function(y){return y != Math.round(y)?'':y;},
|
||||
grid: false,
|
||||
//ymin: 0,
|
||||
//parseTime: false,
|
||||
hideHover:'auto'
|
||||
});
|
||||
</script>
|
||||
|
||||
</body>
|
||||
</html>
|
|
@ -0,0 +1,26 @@
|
|||
# Hi Survivor !!
|
||||
|
||||
|
||||
*Little Stat for Dayz Standalone server.*
|
||||
|
||||
|
||||
- Rquire Omega (to check mod only)
|
||||
|
||||
- Requie mysql database (to store status for graph)
|
||||
|
||||
- You need to set your `ip` , `port` , `query` and `omega server port mod` in `config.php`
|
||||
|
||||
|
||||
STILL in Devlopment ...
|
||||
|
||||
|
||||
|
||||
-----
|
||||
|
||||
|
||||
|
||||
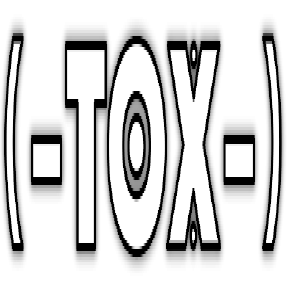
|
||||
|
||||
Website: [dayz.echosystem.fr](https://dayz.echosystem.fr)
|
||||
|
||||
Author: Erreur32
|
|
@ -0,0 +1,139 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
use xPaw\SourceQuery\Exception\InvalidPacketException;
|
||||
use xPaw\SourceQuery\Exception\SocketException;
|
||||
|
||||
/**
|
||||
* Base socket interface
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*
|
||||
* @uses xPaw\SourceQuery\Exception\InvalidPacketException
|
||||
* @uses xPaw\SourceQuery\Exception\SocketException
|
||||
*/
|
||||
abstract class BaseSocket
|
||||
{
|
||||
/** @var resource */
|
||||
public $Socket;
|
||||
public int $Engine;
|
||||
|
||||
public string $Address;
|
||||
public int $Port;
|
||||
public int $Timeout;
|
||||
|
||||
public function __destruct( )
|
||||
{
|
||||
$this->Close( );
|
||||
}
|
||||
|
||||
abstract public function Close( ) : void;
|
||||
abstract public function Open( string $Address, int $Port, int $Timeout, int $Engine ) : void;
|
||||
abstract public function Write( int $Header, string $String = '' ) : bool;
|
||||
abstract public function Read( int $Length = 1400 ) : Buffer;
|
||||
|
||||
protected function ReadInternal( Buffer $Buffer, int $Length, callable $SherlockFunction ) : Buffer
|
||||
{
|
||||
if( $Buffer->Remaining( ) === 0 )
|
||||
{
|
||||
throw new InvalidPacketException( 'Failed to read any data from socket', InvalidPacketException::BUFFER_EMPTY );
|
||||
}
|
||||
|
||||
$Header = $Buffer->GetLong( );
|
||||
|
||||
if( $Header === -1 ) // Single packet
|
||||
{
|
||||
// We don't have to do anything
|
||||
}
|
||||
else if( $Header === -2 ) // Split packet
|
||||
{
|
||||
$Packets = [];
|
||||
$IsCompressed = false;
|
||||
$ReadMore = false;
|
||||
$PacketChecksum = null;
|
||||
|
||||
do
|
||||
{
|
||||
$RequestID = $Buffer->GetLong( );
|
||||
|
||||
switch( $this->Engine )
|
||||
{
|
||||
case SourceQuery::GOLDSOURCE:
|
||||
{
|
||||
$PacketCountAndNumber = $Buffer->GetByte( );
|
||||
$PacketCount = $PacketCountAndNumber & 0xF;
|
||||
$PacketNumber = $PacketCountAndNumber >> 4;
|
||||
|
||||
break;
|
||||
}
|
||||
case SourceQuery::SOURCE:
|
||||
{
|
||||
$IsCompressed = ( $RequestID & 0x80000000 ) !== 0;
|
||||
$PacketCount = $Buffer->GetByte( );
|
||||
$PacketNumber = $Buffer->GetByte( ) + 1;
|
||||
|
||||
if( $IsCompressed )
|
||||
{
|
||||
$Buffer->GetLong( ); // Split size
|
||||
|
||||
$PacketChecksum = $Buffer->GetUnsignedLong( );
|
||||
}
|
||||
else
|
||||
{
|
||||
$Buffer->GetShort( ); // Split size
|
||||
}
|
||||
|
||||
break;
|
||||
}
|
||||
default:
|
||||
{
|
||||
throw new SocketException( 'Unknown engine.', SocketException::INVALID_ENGINE );
|
||||
}
|
||||
}
|
||||
|
||||
$Packets[ $PacketNumber ] = $Buffer->Get( );
|
||||
|
||||
$ReadMore = $PacketCount > sizeof( $Packets );
|
||||
}
|
||||
while( $ReadMore && $SherlockFunction( $Buffer, $Length ) );
|
||||
|
||||
$Data = Implode( $Packets );
|
||||
|
||||
// TODO: Test this
|
||||
if( $IsCompressed )
|
||||
{
|
||||
// Let's make sure this function exists, it's not included in PHP by default
|
||||
if( !Function_Exists( 'bzdecompress' ) )
|
||||
{
|
||||
throw new \RuntimeException( 'Received compressed packet, PHP doesn\'t have Bzip2 library installed, can\'t decompress.' );
|
||||
}
|
||||
|
||||
$Data = bzdecompress( $Data );
|
||||
|
||||
if( !is_string( $Data ) || CRC32( $Data ) !== $PacketChecksum )
|
||||
{
|
||||
throw new InvalidPacketException( 'CRC32 checksum mismatch of uncompressed packet data.', InvalidPacketException::CHECKSUM_MISMATCH );
|
||||
}
|
||||
}
|
||||
|
||||
$Buffer->Set( SubStr( $Data, 4 ) );
|
||||
}
|
||||
else
|
||||
{
|
||||
throw new InvalidPacketException( 'Socket read: Raw packet header mismatch. (0x' . DecHex( $Header ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
|
||||
return $Buffer;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,187 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
/**
|
||||
* Class Buffer
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*/
|
||||
class Buffer
|
||||
{
|
||||
/**
|
||||
* Buffer
|
||||
*
|
||||
* @var string
|
||||
*/
|
||||
private $Buffer;
|
||||
|
||||
/**
|
||||
* Buffer length
|
||||
*
|
||||
* @var int
|
||||
*/
|
||||
private $Length;
|
||||
|
||||
/**
|
||||
* Current position in buffer
|
||||
*
|
||||
* @var int
|
||||
*/
|
||||
private $Position;
|
||||
|
||||
/**
|
||||
* Sets buffer
|
||||
*
|
||||
* @param string $Buffer Buffer
|
||||
*/
|
||||
public function Set( $Buffer )
|
||||
{
|
||||
$this->Buffer = $Buffer;
|
||||
$this->Length = StrLen( $Buffer );
|
||||
$this->Position = 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Resets buffer
|
||||
*/
|
||||
public function Reset( )
|
||||
{
|
||||
$this->Buffer = "";
|
||||
$this->Length = 0;
|
||||
$this->Position = 0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get remaining bytes
|
||||
*
|
||||
* @return int Remaining bytes in buffer
|
||||
*/
|
||||
public function Remaining( )
|
||||
{
|
||||
return $this->Length - $this->Position;
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets data from buffer
|
||||
*
|
||||
* @param int $Length Bytes to read
|
||||
*
|
||||
* @return string
|
||||
*/
|
||||
public function Get( $Length = -1 )
|
||||
{
|
||||
if( $Length === 0 )
|
||||
{
|
||||
return '';
|
||||
}
|
||||
|
||||
$Remaining = $this->Remaining( );
|
||||
|
||||
if( $Length === -1 )
|
||||
{
|
||||
$Length = $Remaining;
|
||||
}
|
||||
else if( $Length > $Remaining )
|
||||
{
|
||||
return '';
|
||||
}
|
||||
|
||||
$Data = SubStr( $this->Buffer, $this->Position, $Length );
|
||||
|
||||
$this->Position += $Length;
|
||||
|
||||
return $Data;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get byte from buffer
|
||||
*
|
||||
* @return int
|
||||
*/
|
||||
public function GetByte( )
|
||||
{
|
||||
return Ord( $this->Get( 1 ) );
|
||||
}
|
||||
|
||||
/**
|
||||
* Get short from buffer
|
||||
*
|
||||
* @return int
|
||||
*/
|
||||
public function GetShort( )
|
||||
{
|
||||
$Data = UnPack( 'v', $this->Get( 2 ) );
|
||||
|
||||
return $Data[ 1 ];
|
||||
}
|
||||
|
||||
/**
|
||||
* Get long from buffer
|
||||
*
|
||||
* @return int
|
||||
*/
|
||||
public function GetLong( )
|
||||
{
|
||||
$Data = UnPack( 'l', $this->Get( 4 ) );
|
||||
|
||||
return $Data[ 1 ];
|
||||
}
|
||||
|
||||
/**
|
||||
* Get float from buffer
|
||||
*
|
||||
* @return float
|
||||
*/
|
||||
public function GetFloat( )
|
||||
{
|
||||
$Data = UnPack( 'f', $this->Get( 4 ) );
|
||||
|
||||
return $Data[ 1 ];
|
||||
}
|
||||
|
||||
/**
|
||||
* Get unsigned long from buffer
|
||||
*
|
||||
* @return int
|
||||
*/
|
||||
public function GetUnsignedLong( )
|
||||
{
|
||||
$Data = UnPack( 'V', $this->Get( 4 ) );
|
||||
|
||||
return $Data[ 1 ];
|
||||
}
|
||||
|
||||
/**
|
||||
* Read one string from buffer ending with null byte
|
||||
*
|
||||
* @return string
|
||||
*/
|
||||
public function GetString( )
|
||||
{
|
||||
$ZeroBytePosition = StrPos( $this->Buffer, "\0", $this->Position );
|
||||
|
||||
if( $ZeroBytePosition === false )
|
||||
{
|
||||
$String = "";
|
||||
}
|
||||
else
|
||||
{
|
||||
$String = $this->Get( $ZeroBytePosition - $this->Position );
|
||||
|
||||
$this->Position++;
|
||||
}
|
||||
|
||||
return $String;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,19 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
class AuthenticationException extends SourceQueryException
|
||||
{
|
||||
const BAD_PASSWORD = 1;
|
||||
const BANNED = 2;
|
||||
}
|
|
@ -0,0 +1,18 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
class InvalidArgumentException extends SourceQueryException
|
||||
{
|
||||
const TIMEOUT_NOT_INTEGER = 1;
|
||||
}
|
|
@ -0,0 +1,21 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
class InvalidPacketException extends SourceQueryException
|
||||
{
|
||||
const PACKET_HEADER_MISMATCH = 1;
|
||||
const BUFFER_EMPTY = 2;
|
||||
const BUFFER_NOT_EMPTY = 3;
|
||||
const CHECKSUM_MISMATCH = 4;
|
||||
}
|
|
@ -0,0 +1,18 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
class SocketException extends SourceQueryException
|
||||
{
|
||||
const COULD_NOT_CREATE_SOCKET = 1;
|
||||
}
|
|
@ -0,0 +1,18 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
abstract class SourceQueryException extends \Exception
|
||||
{
|
||||
// Base exception class
|
||||
}
|
|
@ -0,0 +1,18 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery\Exception;
|
||||
|
||||
class TimeoutException extends SourceQueryException
|
||||
{
|
||||
const TIMEOUT_CONNECT = 1;
|
||||
}
|
|
@ -0,0 +1,150 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
use xPaw\SourceQuery\Exception\AuthenticationException;
|
||||
|
||||
/**
|
||||
* Class GoldSourceRcon
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*
|
||||
* @uses xPaw\SourceQuery\Exception\AuthenticationException
|
||||
*/
|
||||
class GoldSourceRcon
|
||||
{
|
||||
/**
|
||||
* Points to buffer class
|
||||
*
|
||||
* @var Buffer
|
||||
*/
|
||||
private $Buffer;
|
||||
|
||||
/**
|
||||
* Points to socket class
|
||||
*
|
||||
* @var Socket
|
||||
*/
|
||||
private $Socket;
|
||||
|
||||
private $RconPassword;
|
||||
private $RconRequestId;
|
||||
private $RconChallenge;
|
||||
|
||||
public function __construct( $Buffer, $Socket )
|
||||
{
|
||||
$this->Buffer = $Buffer;
|
||||
$this->Socket = $Socket;
|
||||
}
|
||||
|
||||
public function Close( )
|
||||
{
|
||||
$this->RconChallenge = 0;
|
||||
$this->RconRequestId = 0;
|
||||
$this->RconPassword = 0;
|
||||
}
|
||||
|
||||
public function Open( )
|
||||
{
|
||||
//
|
||||
}
|
||||
|
||||
public function Write( $Header, $String = '' )
|
||||
{
|
||||
$Command = Pack( 'cccca*', 0xFF, 0xFF, 0xFF, 0xFF, $String );
|
||||
$Length = StrLen( $Command );
|
||||
|
||||
return $Length === FWrite( $this->Socket->Socket, $Command, $Length );
|
||||
}
|
||||
|
||||
/**
|
||||
* @param int $Length
|
||||
* @throws AuthenticationException
|
||||
* @return bool
|
||||
*/
|
||||
public function Read( $Length = 1400 )
|
||||
{
|
||||
// GoldSource RCON has same structure as Query
|
||||
$this->Socket->Read( );
|
||||
|
||||
if( $this->Buffer->GetByte( ) !== SourceQuery::S2A_RCON )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$Buffer = $this->Buffer->Get( );
|
||||
$Trimmed = Trim( $Buffer );
|
||||
|
||||
if( $Trimmed === 'Bad rcon_password.' )
|
||||
{
|
||||
throw new AuthenticationException( $Trimmed, AuthenticationException::BAD_PASSWORD );
|
||||
}
|
||||
else if( $Trimmed === 'You have been banned from this server.' )
|
||||
{
|
||||
throw new AuthenticationException( $Trimmed, AuthenticationException::BANNED );
|
||||
}
|
||||
|
||||
$ReadMore = false;
|
||||
|
||||
// There is no indentifier of the end, so we just need to continue reading
|
||||
// TODO: Needs to be looked again, it causes timeouts
|
||||
do
|
||||
{
|
||||
$this->Socket->Read( );
|
||||
|
||||
$ReadMore = $this->Buffer->Remaining( ) > 0 && $this->Buffer->GetByte( ) === SourceQuery::S2A_RCON;
|
||||
|
||||
if( $ReadMore )
|
||||
{
|
||||
$Packet = $this->Buffer->Get( );
|
||||
$Buffer .= SubStr( $Packet, 0, -2 );
|
||||
|
||||
// Let's assume if this packet is not long enough, there are no more after this one
|
||||
$ReadMore = StrLen( $Packet ) > 1000; // use 1300?
|
||||
}
|
||||
}
|
||||
while( $ReadMore );
|
||||
|
||||
$this->Buffer->Set( Trim( $Buffer ) );
|
||||
}
|
||||
|
||||
public function Command( $Command )
|
||||
{
|
||||
if( !$this->RconChallenge )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$this->Write( 0, 'rcon ' . $this->RconChallenge . ' "' . $this->RconPassword . '" ' . $Command . "\0" );
|
||||
$this->Read( );
|
||||
|
||||
return $this->Buffer->Get( );
|
||||
}
|
||||
|
||||
public function Authorize( $Password )
|
||||
{
|
||||
$this->RconPassword = $Password;
|
||||
|
||||
$this->Write( 0, 'challenge rcon' );
|
||||
$this->Socket->Read( );
|
||||
|
||||
if( $this->Buffer->Get( 14 ) !== 'challenge rcon' )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$this->RconChallenge = Trim( $this->Buffer->Get( ) );
|
||||
|
||||
return true;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,193 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
use xPaw\SourceQuery\Exception\InvalidPacketException;
|
||||
use xPaw\SourceQuery\Exception\SocketException;
|
||||
|
||||
/**
|
||||
* Class Socket
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*
|
||||
* @uses xPaw\SourceQuery\Exception\InvalidPacketException
|
||||
* @uses xPaw\SourceQuery\Exception\SocketException
|
||||
*/
|
||||
class Socket
|
||||
{
|
||||
public $Socket;
|
||||
public $Engine;
|
||||
|
||||
public $Ip;
|
||||
public $Port;
|
||||
public $Timeout;
|
||||
|
||||
/**
|
||||
* Points to buffer class
|
||||
*
|
||||
* @var Buffer
|
||||
*/
|
||||
private $Buffer;
|
||||
|
||||
public function __construct( $Buffer )
|
||||
{
|
||||
$this->Buffer = $Buffer;
|
||||
}
|
||||
|
||||
public function Close( )
|
||||
{
|
||||
if( $this->Socket )
|
||||
{
|
||||
FClose( $this->Socket );
|
||||
|
||||
$this->Socket = null;
|
||||
}
|
||||
}
|
||||
|
||||
public function Open( $Ip, $Port, $Timeout, $Engine )
|
||||
{
|
||||
$this->Timeout = $Timeout;
|
||||
$this->Engine = $Engine;
|
||||
$this->Port = $Port;
|
||||
$this->Ip = $Ip;
|
||||
|
||||
$this->Socket = @FSockOpen( 'udp://' . $Ip, $Port, $ErrNo, $ErrStr, $Timeout );
|
||||
|
||||
if( $ErrNo || $this->Socket === false )
|
||||
{
|
||||
throw new SocketException( 'Could not create socket: ' . $ErrStr, SocketException::COULD_NOT_CREATE_SOCKET );
|
||||
}
|
||||
|
||||
Stream_Set_Timeout( $this->Socket, $Timeout );
|
||||
Stream_Set_Blocking( $this->Socket, true );
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
public function Write( $Header, $String = '' )
|
||||
{
|
||||
$Command = Pack( 'ccccca*', 0xFF, 0xFF, 0xFF, 0xFF, $Header, $String );
|
||||
$Length = StrLen( $Command );
|
||||
|
||||
return $Length === FWrite( $this->Socket, $Command, $Length );
|
||||
}
|
||||
|
||||
public function Read( $Length = 1400 )
|
||||
{
|
||||
$this->ReadBuffer( FRead( $this->Socket, $Length ), $Length );
|
||||
}
|
||||
|
||||
protected function ReadBuffer( $Buffer, $Length )
|
||||
{
|
||||
$this->Buffer->Set( $Buffer );
|
||||
|
||||
if( $this->Buffer->Remaining( ) === 0 )
|
||||
{
|
||||
// TODO: Should we throw an exception here?
|
||||
return;
|
||||
}
|
||||
|
||||
$Header = $this->Buffer->GetLong( );
|
||||
|
||||
if( $Header === -1 ) // Single packet
|
||||
{
|
||||
// We don't have to do anything
|
||||
}
|
||||
else if( $Header === -2 ) // Split packet
|
||||
{
|
||||
$Packets = Array( );
|
||||
$IsCompressed = false;
|
||||
$ReadMore = false;
|
||||
|
||||
do
|
||||
{
|
||||
$RequestID = $this->Buffer->GetLong( );
|
||||
|
||||
switch( $this->Engine )
|
||||
{
|
||||
case SourceQuery::GOLDSOURCE:
|
||||
{
|
||||
$PacketCountAndNumber = $this->Buffer->GetByte( );
|
||||
$PacketCount = $PacketCountAndNumber & 0xF;
|
||||
$PacketNumber = $PacketCountAndNumber >> 4;
|
||||
|
||||
break;
|
||||
}
|
||||
case SourceQuery::SOURCE:
|
||||
{
|
||||
$IsCompressed = ( $RequestID & 0x80000000 ) !== 0;
|
||||
$PacketCount = $this->Buffer->GetByte( );
|
||||
$PacketNumber = $this->Buffer->GetByte( ) + 1;
|
||||
|
||||
if( $IsCompressed )
|
||||
{
|
||||
$this->Buffer->GetLong( ); // Split size
|
||||
|
||||
$PacketChecksum = $this->Buffer->GetUnsignedLong( );
|
||||
}
|
||||
else
|
||||
{
|
||||
$this->Buffer->GetShort( ); // Split size
|
||||
}
|
||||
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
$Packets[ $PacketNumber ] = $this->Buffer->Get( );
|
||||
|
||||
$ReadMore = $PacketCount > sizeof( $Packets );
|
||||
}
|
||||
while( $ReadMore && $this->Sherlock( $Length ) );
|
||||
|
||||
$Buffer = Implode( $Packets );
|
||||
|
||||
// TODO: Test this
|
||||
if( $IsCompressed )
|
||||
{
|
||||
// Let's make sure this function exists, it's not included in PHP by default
|
||||
if( !Function_Exists( 'bzdecompress' ) )
|
||||
{
|
||||
throw new \RuntimeException( 'Received compressed packet, PHP doesn\'t have Bzip2 library installed, can\'t decompress.' );
|
||||
}
|
||||
|
||||
$Buffer = bzdecompress( $Buffer );
|
||||
|
||||
if( CRC32( $Buffer ) !== $PacketChecksum )
|
||||
{
|
||||
throw new InvalidPacketException( 'CRC32 checksum mismatch of uncompressed packet data.', InvalidPacketException::CHECKSUM_MISMATCH );
|
||||
}
|
||||
}
|
||||
|
||||
$this->Buffer->Set( SubStr( $Buffer, 4 ) );
|
||||
}
|
||||
else
|
||||
{
|
||||
throw new InvalidPacketException( 'Socket read: Raw packet header mismatch. (0x' . DecHex( $Header ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
}
|
||||
|
||||
private function Sherlock( $Length )
|
||||
{
|
||||
$Data = FRead( $this->Socket, $Length );
|
||||
|
||||
if( StrLen( $Data ) < 4 )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$this->Buffer->Set( $Data );
|
||||
|
||||
return $this->Buffer->GetLong( ) === -2;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,572 @@
|
|||
<?php
|
||||
/**
|
||||
* This class provides the public interface to the PHP-Source-Query library.
|
||||
*
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
use xPaw\SourceQuery\Exception\InvalidArgumentException;
|
||||
use xPaw\SourceQuery\Exception\TimeoutException;
|
||||
use xPaw\SourceQuery\Exception\InvalidPacketException;
|
||||
|
||||
/**
|
||||
* Class SourceQuery
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*
|
||||
* @uses xPaw\SourceQuery\Exception\InvalidArgumentException
|
||||
* @uses xPaw\SourceQuery\Exception\TimeoutException
|
||||
* @uses xPaw\SourceQuery\Exception\InvalidPacketException
|
||||
*/
|
||||
class SourceQuery
|
||||
{
|
||||
/**
|
||||
* Values returned by GetChallenge()
|
||||
*
|
||||
* @todo Get rid of this? Improve? Do something else?
|
||||
*/
|
||||
const GETCHALLENGE_FAILED = 0;
|
||||
const GETCHALLENGE_ALL_CLEAR = 1;
|
||||
const GETCHALLENGE_CONTAINS_ANSWER = 2;
|
||||
|
||||
/**
|
||||
* Engines
|
||||
*/
|
||||
const GOLDSOURCE = 0;
|
||||
const SOURCE = 1;
|
||||
|
||||
/**
|
||||
* Packets sent
|
||||
*/
|
||||
const A2S_PING = 0x69;
|
||||
const A2S_INFO = 0x54;
|
||||
const A2S_PLAYER = 0x55;
|
||||
const A2S_RULES = 0x56;
|
||||
const A2S_SERVERQUERY_GETCHALLENGE = 0x57;
|
||||
|
||||
/**
|
||||
* Packets received
|
||||
*/
|
||||
const S2A_PING = 0x6A;
|
||||
const S2A_CHALLENGE = 0x41;
|
||||
const S2A_INFO = 0x49;
|
||||
const S2A_INFO_OLD = 0x6D; // Old GoldSource, HLTV uses it
|
||||
const S2A_PLAYER = 0x44;
|
||||
const S2A_RULES = 0x45;
|
||||
const S2A_RCON = 0x6C;
|
||||
|
||||
/**
|
||||
* Source rcon sent
|
||||
*/
|
||||
const SERVERDATA_EXECCOMMAND = 2;
|
||||
const SERVERDATA_AUTH = 3;
|
||||
|
||||
/**
|
||||
* Source rcon received
|
||||
*/
|
||||
const SERVERDATA_RESPONSE_VALUE = 0;
|
||||
const SERVERDATA_AUTH_RESPONSE = 2;
|
||||
|
||||
/**
|
||||
* Points to rcon class
|
||||
*
|
||||
* @var SourceRcon
|
||||
*/
|
||||
private $Rcon;
|
||||
|
||||
/**
|
||||
* Points to buffer class
|
||||
*
|
||||
* @var Buffer
|
||||
*/
|
||||
private $Buffer;
|
||||
|
||||
/**
|
||||
* Points to socket class
|
||||
*
|
||||
* @var Socket
|
||||
*/
|
||||
private $Socket;
|
||||
|
||||
/**
|
||||
* True if connection is open, false if not
|
||||
*
|
||||
* @var bool
|
||||
*/
|
||||
private $Connected;
|
||||
|
||||
/**
|
||||
* Contains challenge
|
||||
*
|
||||
* @var string
|
||||
*/
|
||||
private $Challenge;
|
||||
|
||||
/**
|
||||
* Use old method for getting challenge number
|
||||
*
|
||||
* @var bool
|
||||
*/
|
||||
private $UseOldGetChallengeMethod;
|
||||
|
||||
public function __construct( )
|
||||
{
|
||||
$this->Buffer = new Buffer( );
|
||||
$this->Socket = new Socket( $this->Buffer );
|
||||
}
|
||||
|
||||
public function __destruct( )
|
||||
{
|
||||
$this->Disconnect( );
|
||||
}
|
||||
|
||||
/**
|
||||
* Opens connection to server
|
||||
*
|
||||
* @param string $Ip Server ip
|
||||
* @param int $Port Server port
|
||||
* @param int $Timeout Timeout period
|
||||
* @param int $Engine Engine the server runs on (goldsource, source)
|
||||
*
|
||||
* @throws InvalidArgumentException
|
||||
* @throws TimeoutException
|
||||
*/
|
||||
public function Connect( $Ip, $Port, $Timeout = 3, $Engine = self::SOURCE )
|
||||
{
|
||||
$this->Disconnect( );
|
||||
|
||||
if( !is_int( $Timeout ) || $Timeout < 0 )
|
||||
{
|
||||
throw new InvalidArgumentException( 'Timeout must be an integer.', InvalidArgumentException::TIMEOUT_NOT_INTEGER );
|
||||
}
|
||||
|
||||
if( !$this->Socket->Open( $Ip, (int)$Port, $Timeout, (int)$Engine ) )
|
||||
{
|
||||
throw new TimeoutException( 'Could not connect to server.', TimeoutException::TIMEOUT_CONNECT );
|
||||
}
|
||||
|
||||
$this->Connected = true;
|
||||
}
|
||||
|
||||
/**
|
||||
* Forces GetChallenge to use old method for challenge retrieval because some games use outdated protocol (e.g Starbound)
|
||||
*
|
||||
* @param bool $Value Set to true to force old method
|
||||
*
|
||||
* @returns bool Previous value
|
||||
*/
|
||||
public function SetUseOldGetChallengeMethod( $Value )
|
||||
{
|
||||
$Previous = $this->UseOldGetChallengeMethod;
|
||||
|
||||
$this->UseOldGetChallengeMethod = $Value === true;
|
||||
|
||||
return $Previous;
|
||||
}
|
||||
|
||||
/**
|
||||
* Closes all open connections
|
||||
*/
|
||||
public function Disconnect( )
|
||||
{
|
||||
$this->Connected = false;
|
||||
$this->Challenge = 0;
|
||||
|
||||
$this->Buffer->Reset( );
|
||||
|
||||
$this->Socket->Close( );
|
||||
|
||||
if( $this->Rcon )
|
||||
{
|
||||
$this->Rcon->Close( );
|
||||
|
||||
$this->Rcon = null;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Sends ping packet to the server
|
||||
* NOTE: This may not work on some games (TF2 for example)
|
||||
*
|
||||
* @return bool True on success, false on failure
|
||||
*/
|
||||
public function Ping( )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$this->Socket->Write( self::A2S_PING );
|
||||
$this->Socket->Read( );
|
||||
|
||||
return $this->Buffer->GetByte( ) === self::S2A_PING;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get server information
|
||||
*
|
||||
* @throws InvalidPacketException
|
||||
*
|
||||
* @return bool|array Returns array with information on success, false on failure
|
||||
*/
|
||||
public function GetInfo( )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$this->Socket->Write( self::A2S_INFO, "Source Engine Query\0" );
|
||||
$this->Socket->Read( );
|
||||
|
||||
$Type = $this->Buffer->GetByte( );
|
||||
|
||||
if( $Type === 0 )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
// Old GoldSource protocol, HLTV still uses it
|
||||
if( $Type === self::S2A_INFO_OLD && $this->Socket->Engine === self::GOLDSOURCE )
|
||||
{
|
||||
/**
|
||||
* If we try to read data again, and we get the result with type S2A_INFO (0x49)
|
||||
* That means this server is running dproto,
|
||||
* Because it sends answer for both protocols
|
||||
*/
|
||||
|
||||
$Server[ 'Address' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'HostName' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'Map' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'ModDir' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'ModDesc' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'Players' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'MaxPlayers' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'Protocol' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'Dedicated' ] = Chr( $this->Buffer->GetByte( ) );
|
||||
$Server[ 'Os' ] = Chr( $this->Buffer->GetByte( ) );
|
||||
$Server[ 'Password' ] = $this->Buffer->GetByte( ) === 1;
|
||||
$Server[ 'IsMod' ] = $this->Buffer->GetByte( ) === 1;
|
||||
|
||||
if( $Server[ 'IsMod' ] )
|
||||
{
|
||||
$Mod[ 'Url' ] = $this->Buffer->GetString( );
|
||||
$Mod[ 'Download' ] = $this->Buffer->GetString( );
|
||||
$this->Buffer->Get( 1 ); // NULL byte
|
||||
$Mod[ 'Version' ] = $this->Buffer->GetLong( );
|
||||
$Mod[ 'Size' ] = $this->Buffer->GetLong( );
|
||||
$Mod[ 'ServerSide' ] = $this->Buffer->GetByte( ) === 1;
|
||||
$Mod[ 'CustomDLL' ] = $this->Buffer->GetByte( ) === 1;
|
||||
}
|
||||
|
||||
$Server[ 'Secure' ] = $this->Buffer->GetByte( ) === 1;
|
||||
$Server[ 'Bots' ] = $this->Buffer->GetByte( );
|
||||
|
||||
if( isset( $Mod ) )
|
||||
{
|
||||
$Server[ 'Mod' ] = $Mod;
|
||||
}
|
||||
|
||||
return $Server;
|
||||
}
|
||||
|
||||
if( $Type !== self::S2A_INFO )
|
||||
{
|
||||
throw new InvalidPacketException( 'GetInfo: Packet header mismatch. (0x' . DecHex( $Type ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
|
||||
$Server[ 'Protocol' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'HostName' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'Map' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'ModDir' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'ModDesc' ] = $this->Buffer->GetString( );
|
||||
$Server[ 'AppID' ] = $this->Buffer->GetShort( );
|
||||
$Server[ 'Players' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'MaxPlayers' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'Bots' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'Dedicated' ] = Chr( $this->Buffer->GetByte( ) );
|
||||
$Server[ 'Os' ] = Chr( $this->Buffer->GetByte( ) );
|
||||
$Server[ 'Password' ] = $this->Buffer->GetByte( ) === 1;
|
||||
$Server[ 'Secure' ] = $this->Buffer->GetByte( ) === 1;
|
||||
|
||||
// The Ship (they violate query protocol spec by modifying the response)
|
||||
if( $Server[ 'AppID' ] === 2400 )
|
||||
{
|
||||
$Server[ 'GameMode' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'WitnessCount' ] = $this->Buffer->GetByte( );
|
||||
$Server[ 'WitnessTime' ] = $this->Buffer->GetByte( );
|
||||
}
|
||||
|
||||
$Server[ 'Version' ] = $this->Buffer->GetString( );
|
||||
|
||||
// Extra Data Flags
|
||||
if( $this->Buffer->Remaining( ) > 0 )
|
||||
{
|
||||
$Server[ 'ExtraDataFlags' ] = $Flags = $this->Buffer->GetByte( );
|
||||
|
||||
// The server's game port
|
||||
if( $Flags & 0x80 )
|
||||
{
|
||||
$Server[ 'GamePort' ] = $this->Buffer->GetShort( );
|
||||
}
|
||||
|
||||
// The server's SteamID - does this serve any purpose?
|
||||
if( $Flags & 0x10 )
|
||||
{
|
||||
$Server[ 'ServerID' ] = $this->Buffer->GetUnsignedLong( ) | ( $this->Buffer->GetUnsignedLong( ) << 32 ); // TODO: verify this
|
||||
}
|
||||
|
||||
// The spectator port and then the spectator server name
|
||||
if( $Flags & 0x40 )
|
||||
{
|
||||
$Server[ 'SpecPort' ] = $this->Buffer->GetShort( );
|
||||
$Server[ 'SpecName' ] = $this->Buffer->GetString( );
|
||||
}
|
||||
|
||||
// The game tag data string for the server
|
||||
if( $Flags & 0x20 )
|
||||
{
|
||||
$Server[ 'GameTags' ] = $this->Buffer->GetString( );
|
||||
}
|
||||
|
||||
// GameID -- alternative to AppID?
|
||||
if( $Flags & 0x01 )
|
||||
{
|
||||
$Server[ 'GameID' ] = $this->Buffer->GetUnsignedLong( ) | ( $this->Buffer->GetUnsignedLong( ) << 32 );
|
||||
}
|
||||
|
||||
if( $this->Buffer->Remaining( ) > 0 )
|
||||
{
|
||||
throw new InvalidPacketException( 'GetInfo: unread data? ' . $this->Buffer->Remaining( ) . ' bytes remaining in the buffer. Please report it to the library developer.',
|
||||
InvalidPacketException::BUFFER_NOT_EMPTY );
|
||||
}
|
||||
}
|
||||
|
||||
return $Server;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get players on the server
|
||||
*
|
||||
* @throws InvalidPacketException
|
||||
*
|
||||
* @return bool|array Returns array with players on success, false on failure
|
||||
*/
|
||||
public function GetPlayers( )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
switch( $this->GetChallenge( self::A2S_PLAYER, self::S2A_PLAYER ) )
|
||||
{
|
||||
case self::GETCHALLENGE_FAILED:
|
||||
{
|
||||
return false;
|
||||
}
|
||||
case self::GETCHALLENGE_ALL_CLEAR:
|
||||
{
|
||||
$this->Socket->Write( self::A2S_PLAYER, $this->Challenge );
|
||||
$this->Socket->Read( 14000 ); // Moronic Arma 3 developers do not split their packets, so we have to read more data
|
||||
// This violates the protocol spec, and they probably should fix it: https://developer.valvesoftware.com/wiki/Server_queries#Protocol
|
||||
|
||||
$Type = $this->Buffer->GetByte( );
|
||||
|
||||
if( $Type === 0 )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
else if( $Type !== self::S2A_PLAYER )
|
||||
{
|
||||
throw new InvalidPacketException( 'GetPlayers: Packet header mismatch. (0x' . DecHex( $Type ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
$Players = Array( );
|
||||
$Count = $this->Buffer->GetByte( );
|
||||
|
||||
while( $Count-- > 0 && $this->Buffer->Remaining( ) > 0 )
|
||||
{
|
||||
$Player[ 'Id' ] = $this->Buffer->GetByte( ); // PlayerID, is it just always 0?
|
||||
$Player[ 'Name' ] = $this->Buffer->GetString( );
|
||||
$Player[ 'Frags' ] = $this->Buffer->GetLong( );
|
||||
$Player[ 'Time' ] = (int)$this->Buffer->GetFloat( );
|
||||
$Player[ 'TimeF' ] = GMDate( ( $Player[ 'Time' ] > 3600 ? "H:i:s" : "H:i" ), $Player[ 'Time' ] );
|
||||
|
||||
$Players[ ] = $Player;
|
||||
}
|
||||
|
||||
return $Players;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get rules (cvars) from the server
|
||||
*
|
||||
* @throws InvalidPacketException
|
||||
*
|
||||
* @return bool|array Returns array with rules on success, false on failure
|
||||
*/
|
||||
public function GetRules( )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
switch( $this->GetChallenge( self::A2S_RULES, self::S2A_RULES ) )
|
||||
{
|
||||
case self::GETCHALLENGE_FAILED:
|
||||
{
|
||||
return false;
|
||||
}
|
||||
case self::GETCHALLENGE_ALL_CLEAR:
|
||||
{
|
||||
$this->Socket->Write( self::A2S_RULES, $this->Challenge );
|
||||
$this->Socket->Read( );
|
||||
|
||||
$Type = $this->Buffer->GetByte( );
|
||||
|
||||
if( $Type === 0 )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
else if( $Type !== self::S2A_RULES )
|
||||
{
|
||||
throw new InvalidPacketException( 'GetRules: Packet header mismatch. (0x' . DecHex( $Type ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
$Rules = Array( );
|
||||
$Count = $this->Buffer->GetShort( );
|
||||
|
||||
while( $Count-- > 0 && $this->Buffer->Remaining( ) > 0 )
|
||||
{
|
||||
$Rule = $this->Buffer->GetString( );
|
||||
$Value = $this->Buffer->GetString( );
|
||||
|
||||
if( !Empty( $Rule ) )
|
||||
{
|
||||
$Rules[ $Rule ] = $Value;
|
||||
}
|
||||
}
|
||||
|
||||
return $Rules;
|
||||
}
|
||||
|
||||
/**
|
||||
* Get challenge (used for players/rules packets)
|
||||
*
|
||||
* @param $Header
|
||||
* @param $ExpectedResult
|
||||
* @throws InvalidPacketException
|
||||
* @return bool True if all went well, false if server uses old GoldSource protocol, and it already contains answer
|
||||
*/
|
||||
private function GetChallenge( $Header, $ExpectedResult )
|
||||
{
|
||||
if( $this->Challenge )
|
||||
{
|
||||
return self::GETCHALLENGE_ALL_CLEAR;
|
||||
}
|
||||
|
||||
if( $this->UseOldGetChallengeMethod )
|
||||
{
|
||||
$Header = self::A2S_SERVERQUERY_GETCHALLENGE;
|
||||
}
|
||||
|
||||
$this->Socket->Write( $Header, 0xFFFFFFFF );
|
||||
$this->Socket->Read( );
|
||||
|
||||
$Type = $this->Buffer->GetByte( );
|
||||
|
||||
switch( $Type )
|
||||
{
|
||||
case self::S2A_CHALLENGE:
|
||||
{
|
||||
$this->Challenge = $this->Buffer->Get( 4 );
|
||||
|
||||
return self::GETCHALLENGE_ALL_CLEAR;
|
||||
}
|
||||
case $ExpectedResult:
|
||||
{
|
||||
// Goldsource (HLTV)
|
||||
|
||||
return self::GETCHALLENGE_CONTAINS_ANSWER;
|
||||
}
|
||||
case 0:
|
||||
{
|
||||
return self::GETCHALLENGE_FAILED;
|
||||
}
|
||||
default:
|
||||
{
|
||||
throw new InvalidPacketException( 'GetChallenge: Packet header mismatch. (0x' . DecHex( $Type ) . ')', InvalidPacketException::PACKET_HEADER_MISMATCH );
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Sets rcon password, for future use in Rcon()
|
||||
*
|
||||
* @param string $Password Rcon Password
|
||||
*
|
||||
* @return bool True on success, false on failure
|
||||
*/
|
||||
public function SetRconPassword( $Password )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
switch( $this->Socket->Engine )
|
||||
{
|
||||
case SourceQuery::GOLDSOURCE:
|
||||
{
|
||||
$this->Rcon = new GoldSourceRcon( $this->Buffer, $this->Socket );
|
||||
|
||||
break;
|
||||
}
|
||||
case SourceQuery::SOURCE:
|
||||
{
|
||||
$this->Rcon = new SourceRcon( $this->Buffer, $this->Socket );
|
||||
|
||||
break;
|
||||
}
|
||||
}
|
||||
|
||||
$this->Rcon->Open( );
|
||||
|
||||
return $this->Rcon->Authorize( $Password );
|
||||
}
|
||||
|
||||
/**
|
||||
* Sends a command to the server for execution.
|
||||
*
|
||||
* @param string $Command Command to execute
|
||||
*
|
||||
* @return string|bool Answer from server in string, false on failure
|
||||
*/
|
||||
public function Rcon( $Command )
|
||||
{
|
||||
if( !$this->Connected )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
return $this->Rcon->Command( $Command );
|
||||
}
|
||||
}
|
|
@ -0,0 +1,209 @@
|
|||
<?php
|
||||
/**
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*
|
||||
* @internal
|
||||
*/
|
||||
|
||||
namespace xPaw\SourceQuery;
|
||||
|
||||
use xPaw\SourceQuery\Exception\AuthenticationException;
|
||||
use xPaw\SourceQuery\Exception\TimeoutException;
|
||||
use xPaw\SourceQuery\Exception\InvalidPacketException;
|
||||
|
||||
/**
|
||||
* Class SourceRcon
|
||||
*
|
||||
* @package xPaw\SourceQuery
|
||||
*
|
||||
* @uses xPaw\SourceQuery\Exception\AuthenticationException
|
||||
* @uses xPaw\SourceQuery\Exception\TimeoutException
|
||||
* @uses xPaw\SourceQuery\Exception\InvalidPacketException
|
||||
*/
|
||||
class SourceRcon
|
||||
{
|
||||
/**
|
||||
* Points to buffer class
|
||||
*
|
||||
* @var Buffer
|
||||
*/
|
||||
private $Buffer;
|
||||
|
||||
/**
|
||||
* Points to socket class
|
||||
*
|
||||
* @var Socket
|
||||
*/
|
||||
private $Socket;
|
||||
|
||||
private $RconSocket;
|
||||
private $RconRequestId;
|
||||
|
||||
public function __construct( $Buffer, $Socket )
|
||||
{
|
||||
$this->Buffer = $Buffer;
|
||||
$this->Socket = $Socket;
|
||||
}
|
||||
|
||||
public function Close( )
|
||||
{
|
||||
if( $this->RconSocket )
|
||||
{
|
||||
FClose( $this->RconSocket );
|
||||
|
||||
$this->RconSocket = null;
|
||||
}
|
||||
|
||||
$this->RconRequestId = 0;
|
||||
}
|
||||
|
||||
public function Open( )
|
||||
{
|
||||
if( !$this->RconSocket )
|
||||
{
|
||||
$this->RconSocket = @FSockOpen( $this->Socket->Ip, $this->Socket->Port, $ErrNo, $ErrStr, $this->Socket->Timeout );
|
||||
|
||||
if( $ErrNo || !$this->RconSocket )
|
||||
{
|
||||
throw new TimeoutException( 'Can\'t connect to RCON server: ' . $ErrStr, TimeoutException::TIMEOUT_CONNECT );
|
||||
}
|
||||
|
||||
Stream_Set_Timeout( $this->RconSocket, $this->Socket->Timeout );
|
||||
Stream_Set_Blocking( $this->RconSocket, true );
|
||||
}
|
||||
}
|
||||
|
||||
public function Write( $Header, $String = '' )
|
||||
{
|
||||
// Pack the packet together
|
||||
$Command = Pack( 'VV', ++$this->RconRequestId, $Header ) . $String . "\x00\x00";
|
||||
|
||||
// Prepend packet length
|
||||
$Command = Pack( 'V', StrLen( $Command ) ) . $Command;
|
||||
$Length = StrLen( $Command );
|
||||
|
||||
return $Length === FWrite( $this->RconSocket, $Command, $Length );
|
||||
}
|
||||
|
||||
public function Read( )
|
||||
{
|
||||
$this->Buffer->Set( FRead( $this->RconSocket, 4 ) );
|
||||
|
||||
if( $this->Buffer->Remaining( ) < 4 )
|
||||
{
|
||||
throw new InvalidPacketException( 'Rcon read: Failed to read any data from socket', InvalidPacketException::BUFFER_EMPTY );
|
||||
}
|
||||
|
||||
$PacketSize = $this->Buffer->GetLong( );
|
||||
|
||||
$this->Buffer->Set( FRead( $this->RconSocket, $PacketSize ) );
|
||||
|
||||
$Buffer = $this->Buffer->Get( );
|
||||
|
||||
$Remaining = $PacketSize - StrLen( $Buffer );
|
||||
|
||||
while( $Remaining > 0 )
|
||||
{
|
||||
$Buffer2 = FRead( $this->RconSocket, $Remaining );
|
||||
|
||||
$PacketSize = StrLen( $Buffer2 );
|
||||
|
||||
if( $PacketSize === 0 )
|
||||
{
|
||||
throw new InvalidPacketException( 'Read ' . strlen( $Buffer ) . ' bytes from socket, ' . $Remaining . ' remaining', InvalidPacketException::BUFFER_EMPTY );
|
||||
|
||||
break;
|
||||
}
|
||||
|
||||
$Buffer .= $Buffer2;
|
||||
$Remaining -= $PacketSize;
|
||||
}
|
||||
|
||||
$this->Buffer->Set( $Buffer );
|
||||
}
|
||||
|
||||
public function Command( $Command )
|
||||
{
|
||||
$this->Write( SourceQuery::SERVERDATA_EXECCOMMAND, $Command );
|
||||
|
||||
$this->Read( );
|
||||
|
||||
$this->Buffer->GetLong( ); // RequestID
|
||||
|
||||
$Type = $this->Buffer->GetLong( );
|
||||
|
||||
if( $Type === SourceQuery::SERVERDATA_AUTH_RESPONSE )
|
||||
{
|
||||
throw new AuthenticationException( 'Bad rcon_password.', AuthenticationException::BAD_PASSWORD );
|
||||
}
|
||||
else if( $Type !== SourceQuery::SERVERDATA_RESPONSE_VALUE )
|
||||
{
|
||||
return false;
|
||||
}
|
||||
|
||||
$Buffer = $this->Buffer->Get( );
|
||||
|
||||
// We do this stupid hack to handle split packets
|
||||
// See https://developer.valvesoftware.com/wiki/Source_RCON_Protocol#Multiple-packet_Responses
|
||||
if( StrLen( $Buffer ) >= 4000 )
|
||||
{
|
||||
do
|
||||
{
|
||||
$this->Write( SourceQuery::SERVERDATA_RESPONSE_VALUE );
|
||||
|
||||
$this->Read( );
|
||||
|
||||
$this->Buffer->GetLong( ); // RequestID
|
||||
|
||||
if( $this->Buffer->GetLong( ) !== SourceQuery::SERVERDATA_RESPONSE_VALUE )
|
||||
{
|
||||
break;
|
||||
}
|
||||
|
||||
$Buffer2 = $this->Buffer->Get( );
|
||||
|
||||
if( $Buffer2 === "\x00\x01\x00\x00\x00\x00" )
|
||||
{
|
||||
break;
|
||||
}
|
||||
|
||||
$Buffer .= $Buffer2;
|
||||
}
|
||||
while( true );
|
||||
}
|
||||
|
||||
return rtrim( $Buffer, "\0" );
|
||||
}
|
||||
|
||||
public function Authorize( $Password )
|
||||
{
|
||||
$this->Write( SourceQuery::SERVERDATA_AUTH, $Password );
|
||||
$this->Read( );
|
||||
|
||||
$RequestID = $this->Buffer->GetLong( );
|
||||
$Type = $this->Buffer->GetLong( );
|
||||
|
||||
// If we receive SERVERDATA_RESPONSE_VALUE, then we need to read again
|
||||
// More info: https://developer.valvesoftware.com/wiki/Source_RCON_Protocol#Additional_Comments
|
||||
|
||||
if( $Type === SourceQuery::SERVERDATA_RESPONSE_VALUE )
|
||||
{
|
||||
$this->Read( );
|
||||
|
||||
$RequestID = $this->Buffer->GetLong( );
|
||||
$Type = $this->Buffer->GetLong( );
|
||||
}
|
||||
|
||||
if( $RequestID === -1 || $Type !== SourceQuery::SERVERDATA_AUTH_RESPONSE )
|
||||
{
|
||||
throw new AuthenticationException( 'RCON authorization failed.', AuthenticationException::BAD_PASSWORD );
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
}
|
|
@ -0,0 +1,27 @@
|
|||
<?php
|
||||
/**
|
||||
* Library to query servers that implement Source Engine Query protocol.
|
||||
*
|
||||
* Special thanks to koraktor for his awesome Steam Condenser class,
|
||||
* I used it as a reference at some points.
|
||||
*
|
||||
* @author Pavel Djundik <sourcequery@xpaw.me>
|
||||
*
|
||||
* @link https://xpaw.me
|
||||
* @link https://github.com/xPaw/PHP-Source-Query
|
||||
*
|
||||
* @license GNU Lesser General Public License, version 2.1
|
||||
*/
|
||||
|
||||
require_once __DIR__ . '/Exception/SourceQueryException.php';
|
||||
require_once __DIR__ . '/Exception/AuthenticationException.php';
|
||||
require_once __DIR__ . '/Exception/InvalidArgumentException.php';
|
||||
require_once __DIR__ . '/Exception/SocketException.php';
|
||||
require_once __DIR__ . '/Exception/InvalidPacketException.php';
|
||||
require_once __DIR__ . '/Exception/TimeoutException.php';
|
||||
|
||||
require_once __DIR__ . '/Buffer.php';
|
||||
require_once __DIR__ . '/Socket.php';
|
||||
require_once __DIR__ . '/SourceRcon.php';
|
||||
require_once __DIR__ . '/GoldSourceRcon.php';
|
||||
require_once __DIR__ . '/SourceQuery.php';
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,59 @@
|
|||
<?php
|
||||
ini_set("allow_url_fopen", 1);
|
||||
|
||||
// map description ( need to change )
|
||||
$namemap = "Namalsk Island";
|
||||
|
||||
// Edit this ->
|
||||
|
||||
$ipserv = "" ; // IP server game
|
||||
$portserv = "" ; // Game Server Port
|
||||
$modport = "" ; // Mod port omega (+10)
|
||||
$queryport= "" ; // Queryport
|
||||
|
||||
|
||||
// Don't touch below
|
||||
|
||||
$urlserv = $ipserv.":".$portserv ;
|
||||
$json = file_get_contents('http://'.$ipserv.':'.$modport.'/');
|
||||
$objhigher=json_decode($json); //converts to an object
|
||||
$objlower = $objhigher[0]; // if the json response its multidimensional this lowers it
|
||||
$objlower=json_decode($json); //converts to an array of objects
|
||||
|
||||
|
||||
|
||||
|
||||
// librarie SQ - info serv game
|
||||
require 'SQ_/bootstrap.php';
|
||||
use xPaw\SourceQuery\SourceQuery;
|
||||
|
||||
define( 'SQ_SERVER_ADDR', "${ipserv}" ); // IP server
|
||||
define( 'SQ_SERVER_PORT', "${queryport}" ); // YOUR QUERY PORT
|
||||
define( 'SQ_TIMEOUT', 3 );
|
||||
define( 'SQ_ENGINE', SourceQuery::SOURCE );
|
||||
|
||||
$Timer = MicroTime( true );
|
||||
$Query = new SourceQuery( );
|
||||
|
||||
$Info = Array( );
|
||||
$Players = Array( );
|
||||
|
||||
try
|
||||
{
|
||||
$Query->Connect( SQ_SERVER_ADDR, SQ_SERVER_PORT, SQ_TIMEOUT, SQ_ENGINE );
|
||||
|
||||
$Info = $Query->GetInfo( );
|
||||
$Players = $Query->GetPlayers( );
|
||||
}
|
||||
catch( Exception $e )
|
||||
{
|
||||
$Exception = $e;
|
||||
}
|
||||
|
||||
$Query->Disconnect( );
|
||||
|
||||
$Timer = Number_Format( MicroTime( true ) - $Timer, 4, '.', '' );
|
||||
$InfoGT = $Info['GameTags'];
|
||||
|
||||
?>
|
||||
|
|
@ -0,0 +1,18 @@
|
|||
<?php
|
||||
$servdb = "";
|
||||
$userdb = "";
|
||||
$pdb = "";
|
||||
$dbname = "";
|
||||
|
||||
$con = new mysqli($servdb, $userdb, $pdb, $dbname);
|
||||
if ($con->connect_error) {
|
||||
die("Connection failed: " . $con->connect_error);
|
||||
}
|
||||
else
|
||||
{
|
||||
//echo ("Connect Successfully");
|
||||
}
|
||||
// $query = "SELECT date, numplayers FROM StatServer"; // select column
|
||||
// $aresult = $con->query($query);
|
||||
|
||||
?>
|
|
@ -0,0 +1,49 @@
|
|||
|
||||
<link rel="stylesheet" href="https://dayz.echosystem.fr/bootstrap.min.unminify.css" crossorigin="anonymous">
|
||||
<link href="https://dayz.echosystem.fr/main.css" rel="stylesheet">
|
||||
|
||||
|
||||
|
||||
<!-- Matomo -->
|
||||
<script type="text/javascript">
|
||||
var _paq = window._paq = window._paq || [];
|
||||
/* tracker methods like "setCustomDimension" should be called before "trackPageView" */
|
||||
_paq.push(["setDomains", ["*.dayz.echosystem.fr"]]);
|
||||
_paq.push(['trackPageView']);
|
||||
_paq.push(['enableLinkTracking']);
|
||||
(function() {
|
||||
var u="https://echosystem.fr/PiwM/";
|
||||
_paq.push(['setTrackerUrl', u+'matomo.php']);
|
||||
_paq.push(['setSiteId', '16']);
|
||||
var d=document, g=d.createElement('script'), s=d.getElementsByTagName('script')[0];
|
||||
g.type='text/javascript'; g.async=true; g.src=u+'matomo.js'; s.parentNode.insertBefore(g,s);
|
||||
})();
|
||||
</script>
|
||||
<noscript><p><img src="https://echosystem.fr/PiwM/matomo.php?idsite=16&rec=1" style="border:0;" alt="" /></p></noscript>
|
||||
<!-- End Matomo Code -->
|
||||
|
||||
<!-- Global site tag (gtag.js) - Google Analytics -->
|
||||
<script async src="https://www.googletagmanager.com/gtag/js?id=G-JT9LXGJV0C"></script>
|
||||
<script>
|
||||
window.dataLayer = window.dataLayer || [];
|
||||
function gtag(){dataLayer.push(arguments);}
|
||||
gtag('js', new Date());
|
||||
|
||||
gtag('config', 'G-JT9LXGJV0C');
|
||||
</script>
|
||||
|
||||
|
||||
|
||||
|
||||
<!-- Dark Theme -->
|
||||
<script>
|
||||
$("#darkTrigger").click(function(){
|
||||
if ($("body").hasClass("dark")){
|
||||
$("body").removeClass("dark");
|
||||
}
|
||||
else{
|
||||
$("body").addClass("dark");
|
||||
}
|
||||
});
|
||||
</script>
|
||||
|
|
@ -0,0 +1,143 @@
|
|||
(function(){/*
|
||||
|
||||
Copyright The Closure Library Authors.
|
||||
SPDX-License-Identifier: Apache-2.0
|
||||
*/
|
||||
'use strict';var l;function aa(a){var b=0;return function(){return b<a.length?{done:!1,value:a[b++]}:{done:!0}}}function n(a){var b="undefined"!=typeof Symbol&&Symbol.iterator&&a[Symbol.iterator];return b?b.call(a):{next:aa(a)}}function ba(a){if(!(a instanceof Array)){a=n(a);for(var b,c=[];!(b=a.next()).done;)c.push(b.value);a=c}return a}function ca(a,b,c){a instanceof String&&(a=String(a));for(var d=a.length,e=0;e<d;e++){var f=a[e];if(b.call(c,f,e,a))return{N:e,T:f}}return{N:-1,T:void 0}}
|
||||
var da="function"==typeof Object.defineProperties?Object.defineProperty:function(a,b,c){if(a==Array.prototype||a==Object.prototype)return a;a[b]=c.value;return a};function ea(a){a=["object"==typeof globalThis&&globalThis,a,"object"==typeof window&&window,"object"==typeof self&&self,"object"==typeof global&&global];for(var b=0;b<a.length;++b){var c=a[b];if(c&&c.Math==Math)return c}throw Error("Cannot find global object");}var fa=ea(this);
|
||||
function q(a,b){if(b)a:{var c=fa;a=a.split(".");for(var d=0;d<a.length-1;d++){var e=a[d];if(!(e in c))break a;c=c[e]}a=a[a.length-1];d=c[a];b=b(d);b!=d&&null!=b&&da(c,a,{configurable:!0,writable:!0,value:b})}}q("Array.prototype.findIndex",function(a){return a?a:function(b,c){return ca(this,b,c).N}});q("Array.prototype.find",function(a){return a?a:function(b,c){return ca(this,b,c).T}});
|
||||
function r(a,b,c){if(null==a)throw new TypeError("The 'this' value for String.prototype."+c+" must not be null or undefined");if(b instanceof RegExp)throw new TypeError("First argument to String.prototype."+c+" must not be a regular expression");return a+""}q("String.prototype.endsWith",function(a){return a?a:function(b,c){var d=r(this,b,"endsWith");void 0===c&&(c=d.length);c=Math.max(0,Math.min(c|0,d.length));for(var e=b.length;0<e&&0<c;)if(d[--c]!=b[--e])return!1;return 0>=e}});
|
||||
q("String.prototype.startsWith",function(a){return a?a:function(b,c){var d=r(this,b,"startsWith"),e=d.length,f=b.length;c=Math.max(0,Math.min(c|0,d.length));for(var g=0;g<f&&c<e;)if(d[c++]!=b[g++])return!1;return g>=f}});q("String.prototype.repeat",function(a){return a?a:function(b){var c=r(this,null,"repeat");if(0>b||1342177279<b)throw new RangeError("Invalid count value");b|=0;for(var d="";b;)if(b&1&&(d+=c),b>>>=1)c+=c;return d}});
|
||||
q("String.prototype.trimLeft",function(a){function b(){return this.replace(/^[\s\xa0]+/,"")}return a||b});q("String.prototype.trimStart",function(a){return a||String.prototype.trimLeft});
|
||||
q("Promise",function(a){function b(g){this.b=0;this.c=void 0;this.a=[];var h=this.g();try{g(h.resolve,h.reject)}catch(k){h.reject(k)}}function c(){this.a=null}function d(g){return g instanceof b?g:new b(function(h){h(g)})}if(a)return a;c.prototype.b=function(g){if(null==this.a){this.a=[];var h=this;this.c(function(){h.h()})}this.a.push(g)};var e=fa.setTimeout;c.prototype.c=function(g){e(g,0)};c.prototype.h=function(){for(;this.a&&this.a.length;){var g=this.a;this.a=[];for(var h=0;h<g.length;++h){var k=
|
||||
g[h];g[h]=null;try{k()}catch(m){this.g(m)}}}this.a=null};c.prototype.g=function(g){this.c(function(){throw g;})};b.prototype.g=function(){function g(m){return function(p){k||(k=!0,m.call(h,p))}}var h=this,k=!1;return{resolve:g(this.B),reject:g(this.h)}};b.prototype.B=function(g){if(g===this)this.h(new TypeError("A Promise cannot resolve to itself"));else if(g instanceof b)this.H(g);else{a:switch(typeof g){case "object":var h=null!=g;break a;case "function":h=!0;break a;default:h=!1}h?this.A(g):this.i(g)}};
|
||||
b.prototype.A=function(g){var h=void 0;try{h=g.then}catch(k){this.h(k);return}"function"==typeof h?this.F(h,g):this.i(g)};b.prototype.h=function(g){this.j(2,g)};b.prototype.i=function(g){this.j(1,g)};b.prototype.j=function(g,h){if(0!=this.b)throw Error("Cannot settle("+g+", "+h+"): Promise already settled in state"+this.b);this.b=g;this.c=h;this.m()};b.prototype.m=function(){if(null!=this.a){for(var g=0;g<this.a.length;++g)f.b(this.a[g]);this.a=null}};var f=new c;b.prototype.H=function(g){var h=this.g();
|
||||
g.G(h.resolve,h.reject)};b.prototype.F=function(g,h){var k=this.g();try{g.call(h,k.resolve,k.reject)}catch(m){k.reject(m)}};b.prototype.then=function(g,h){function k(A,I){return"function"==typeof A?function(wa){try{m(A(wa))}catch(xa){p(xa)}}:I}var m,p,x=new b(function(A,I){m=A;p=I});this.G(k(g,m),k(h,p));return x};b.prototype.catch=function(g){return this.then(void 0,g)};b.prototype.G=function(g,h){function k(){switch(m.b){case 1:g(m.c);break;case 2:h(m.c);break;default:throw Error("Unexpected state: "+
|
||||
m.b);}}var m=this;null==this.a?f.b(k):this.a.push(k)};b.resolve=d;b.reject=function(g){return new b(function(h,k){k(g)})};b.race=function(g){return new b(function(h,k){for(var m=n(g),p=m.next();!p.done;p=m.next())d(p.value).G(h,k)})};b.all=function(g){var h=n(g),k=h.next();return k.done?d([]):new b(function(m,p){function x(wa){return function(xa){A[wa]=xa;I--;0==I&&m(A)}}var A=[],I=0;do A.push(void 0),I++,d(k.value).G(x(A.length-1),p),k=h.next();while(!k.done)})};return b});
|
||||
q("Object.is",function(a){return a?a:function(b,c){return b===c?0!==b||1/b===1/c:b!==b&&c!==c}});q("Array.prototype.includes",function(a){return a?a:function(b,c){var d=this;d instanceof String&&(d=String(d));var e=d.length;c=c||0;for(0>c&&(c=Math.max(c+e,0));c<e;c++){var f=d[c];if(f===b||Object.is(f,b))return!0}return!1}});q("String.prototype.includes",function(a){return a?a:function(b,c){return-1!==r(this,b,"includes").indexOf(b,c||0)}});
|
||||
q("Array.prototype.copyWithin",function(a){function b(c){c=Number(c);return Infinity===c||-Infinity===c?c:c|0}return a?a:function(c,d,e){var f=this.length;c=b(c);d=b(d);e=void 0===e?f:b(e);c=0>c?Math.max(f+c,0):Math.min(c,f);d=0>d?Math.max(f+d,0):Math.min(d,f);e=0>e?Math.max(f+e,0):Math.min(e,f);if(c<d)for(;d<e;)d in this?this[c++]=this[d++]:(delete this[c++],d++);else for(e=Math.min(e,f+d-c),c+=e-d;e>d;)--e in this?this[--c]=this[e]:delete this[--c];return this}});
|
||||
q("Symbol",function(a){function b(e){if(this instanceof b)throw new TypeError("Symbol is not a constructor");return new c("jscomp_symbol_"+(e||"")+"_"+d++,e)}function c(e,f){this.a=e;da(this,"description",{configurable:!0,writable:!0,value:f})}if(a)return a;c.prototype.toString=function(){return this.a};var d=0;return b});
|
||||
q("Symbol.iterator",function(a){if(a)return a;a=Symbol("Symbol.iterator");for(var b="Array Int8Array Uint8Array Uint8ClampedArray Int16Array Uint16Array Int32Array Uint32Array Float32Array Float64Array".split(" "),c=0;c<b.length;c++){var d=fa[b[c]];"function"===typeof d&&"function"!=typeof d.prototype[a]&&da(d.prototype,a,{configurable:!0,writable:!0,value:function(){return ha(aa(this))}})}return a});q("Symbol.asyncIterator",function(a){return a?a:Symbol("Symbol.asyncIterator")});
|
||||
function ha(a){a={next:a};a[Symbol.iterator]=function(){return this};return a}function ia(a,b){a instanceof String&&(a+="");var c=0,d={next:function(){if(c<a.length){var e=c++;return{value:b(e,a[e]),done:!1}}d.next=function(){return{done:!0,value:void 0}};return d.next()}};d[Symbol.iterator]=function(){return d};return d}q("Array.prototype.entries",function(a){return a?a:function(){return ia(this,function(b,c){return[b,c]})}});
|
||||
q("Array.prototype.fill",function(a){return a?a:function(b,c,d){var e=this.length||0;0>c&&(c=Math.max(0,e+c));if(null==d||d>e)d=e;d=Number(d);0>d&&(d=Math.max(0,e+d));for(c=Number(c||0);c<d;c++)this[c]=b;return this}});q("Array.prototype.flat",function(a){return a?a:function(b){b=void 0===b?1:b;for(var c=[],d=0;d<this.length;d++){var e=this[d];Array.isArray(e)&&0<b?(e=Array.prototype.flat.call(e,b-1),c.push.apply(c,e)):c.push(e)}return c}});
|
||||
q("Array.prototype.flatMap",function(a){return a?a:function(b,c){for(var d=[],e=0;e<this.length;e++){var f=b.call(c,this[e],e,this);Array.isArray(f)?d.push.apply(d,f):d.push(f)}return d}});
|
||||
q("Array.from",function(a){return a?a:function(b,c,d){c=null!=c?c:function(h){return h};var e=[],f="undefined"!=typeof Symbol&&Symbol.iterator&&b[Symbol.iterator];if("function"==typeof f){b=f.call(b);for(var g=0;!(f=b.next()).done;)e.push(c.call(d,f.value,g++))}else for(f=b.length,g=0;g<f;g++)e.push(c.call(d,b[g],g));return e}});q("Array.prototype.keys",function(a){return a?a:function(){return ia(this,function(b){return b})}});q("Array.of",function(a){return a?a:function(b){return Array.from(arguments)}});
|
||||
q("Array.prototype.values",function(a){return a?a:function(){return ia(this,function(b,c){return c})}});var ja;if("function"==typeof Object.setPrototypeOf)ja=Object.setPrototypeOf;else{var ka;a:{var la={X:!0},ma={};try{ma.__proto__=la;ka=ma.X;break a}catch(a){}ka=!1}ja=ka?function(a,b){a.__proto__=b;if(a.__proto__!==b)throw new TypeError(a+" is not extensible");return a}:null}var na=ja;q("globalThis",function(a){return a||fa});function t(a,b){return Object.prototype.hasOwnProperty.call(a,b)}
|
||||
q("WeakMap",function(a){function b(k){this.a=(h+=Math.random()+1).toString();if(k){k=n(k);for(var m;!(m=k.next()).done;)m=m.value,this.set(m[0],m[1])}}function c(){}function d(k){var m=typeof k;return"object"===m&&null!==k||"function"===m}function e(k){if(!t(k,g)){var m=new c;da(k,g,{value:m})}}function f(k){var m=Object[k];m&&(Object[k]=function(p){if(p instanceof c)return p;Object.isExtensible(p)&&e(p);return m(p)})}if(function(){if(!a||!Object.seal)return!1;try{var k=Object.seal({}),m=Object.seal({}),
|
||||
p=new a([[k,2],[m,3]]);if(2!=p.get(k)||3!=p.get(m))return!1;p.delete(k);p.set(m,4);return!p.has(k)&&4==p.get(m)}catch(x){return!1}}())return a;var g="$jscomp_hidden_"+Math.random();f("freeze");f("preventExtensions");f("seal");var h=0;b.prototype.set=function(k,m){if(!d(k))throw Error("Invalid WeakMap key");e(k);if(!t(k,g))throw Error("WeakMap key fail: "+k);k[g][this.a]=m;return this};b.prototype.get=function(k){return d(k)&&t(k,g)?k[g][this.a]:void 0};b.prototype.has=function(k){return d(k)&&t(k,
|
||||
g)&&t(k[g],this.a)};b.prototype.delete=function(k){return d(k)&&t(k,g)&&t(k[g],this.a)?delete k[g][this.a]:!1};return b});
|
||||
q("Map",function(a){function b(){var h={};return h.s=h.next=h.head=h}function c(h,k){var m=h.a;return ha(function(){if(m){for(;m.head!=h.a;)m=m.s;for(;m.next!=m.head;)return m=m.next,{done:!1,value:k(m)};m=null}return{done:!0,value:void 0}})}function d(h,k){var m=k&&typeof k;"object"==m||"function"==m?f.has(k)?m=f.get(k):(m=""+ ++g,f.set(k,m)):m="p_"+k;var p=h.b[m];if(p&&t(h.b,m))for(h=0;h<p.length;h++){var x=p[h];if(k!==k&&x.key!==x.key||k===x.key)return{id:m,list:p,index:h,l:x}}return{id:m,list:p,
|
||||
index:-1,l:void 0}}function e(h){this.b={};this.a=b();this.size=0;if(h){h=n(h);for(var k;!(k=h.next()).done;)k=k.value,this.set(k[0],k[1])}}if(function(){if(!a||"function"!=typeof a||!a.prototype.entries||"function"!=typeof Object.seal)return!1;try{var h=Object.seal({x:4}),k=new a(n([[h,"s"]]));if("s"!=k.get(h)||1!=k.size||k.get({x:4})||k.set({x:4},"t")!=k||2!=k.size)return!1;var m=k.entries(),p=m.next();if(p.done||p.value[0]!=h||"s"!=p.value[1])return!1;p=m.next();return p.done||4!=p.value[0].x||
|
||||
"t"!=p.value[1]||!m.next().done?!1:!0}catch(x){return!1}}())return a;var f=new WeakMap;e.prototype.set=function(h,k){h=0===h?0:h;var m=d(this,h);m.list||(m.list=this.b[m.id]=[]);m.l?m.l.value=k:(m.l={next:this.a,s:this.a.s,head:this.a,key:h,value:k},m.list.push(m.l),this.a.s.next=m.l,this.a.s=m.l,this.size++);return this};e.prototype.delete=function(h){h=d(this,h);return h.l&&h.list?(h.list.splice(h.index,1),h.list.length||delete this.b[h.id],h.l.s.next=h.l.next,h.l.next.s=h.l.s,h.l.head=null,this.size--,
|
||||
!0):!1};e.prototype.clear=function(){this.b={};this.a=this.a.s=b();this.size=0};e.prototype.has=function(h){return!!d(this,h).l};e.prototype.get=function(h){return(h=d(this,h).l)&&h.value};e.prototype.entries=function(){return c(this,function(h){return[h.key,h.value]})};e.prototype.keys=function(){return c(this,function(h){return h.key})};e.prototype.values=function(){return c(this,function(h){return h.value})};e.prototype.forEach=function(h,k){for(var m=this.entries(),p;!(p=m.next()).done;)p=p.value,
|
||||
h.call(k,p[1],p[0],this)};e.prototype[Symbol.iterator]=e.prototype.entries;var g=0;return e});q("Math.acosh",function(a){return a?a:function(b){b=Number(b);return Math.log(b+Math.sqrt(b*b-1))}});q("Math.asinh",function(a){return a?a:function(b){b=Number(b);if(0===b)return b;var c=Math.log(Math.abs(b)+Math.sqrt(b*b+1));return 0>b?-c:c}});
|
||||
q("Math.log1p",function(a){return a?a:function(b){b=Number(b);if(.25>b&&-.25<b){for(var c=b,d=1,e=b,f=0,g=1;f!=e;)c*=b,g*=-1,e=(f=e)+g*c/++d;return e}return Math.log(1+b)}});q("Math.atanh",function(a){if(a)return a;var b=Math.log1p;return function(c){c=Number(c);return(b(c)-b(-c))/2}});q("Math.cbrt",function(a){return a?a:function(b){if(0===b)return b;b=Number(b);var c=Math.pow(Math.abs(b),1/3);return 0>b?-c:c}});
|
||||
q("Math.clz32",function(a){return a?a:function(b){b=Number(b)>>>0;if(0===b)return 32;var c=0;0===(b&4294901760)&&(b<<=16,c+=16);0===(b&4278190080)&&(b<<=8,c+=8);0===(b&4026531840)&&(b<<=4,c+=4);0===(b&3221225472)&&(b<<=2,c+=2);0===(b&2147483648)&&c++;return c}});q("Math.cosh",function(a){if(a)return a;var b=Math.exp;return function(c){c=Number(c);return(b(c)+b(-c))/2}});
|
||||
q("Math.expm1",function(a){return a?a:function(b){b=Number(b);if(.25>b&&-.25<b){for(var c=b,d=1,e=b,f=0;f!=e;)c*=b/++d,e=(f=e)+c;return e}return Math.exp(b)-1}});q("Math.fround",function(a){if(a)return a;if("function"!==typeof Float32Array)return function(c){return c};var b=new Float32Array(1);return function(c){b[0]=c;return b[0]}});
|
||||
q("Math.hypot",function(a){return a?a:function(b){if(2>arguments.length)return arguments.length?Math.abs(arguments[0]):0;var c,d,e;for(c=e=0;c<arguments.length;c++)e=Math.max(e,Math.abs(arguments[c]));if(1E100<e||1E-100>e){if(!e)return e;for(c=d=0;c<arguments.length;c++){var f=Number(arguments[c])/e;d+=f*f}return Math.sqrt(d)*e}for(c=d=0;c<arguments.length;c++)f=Number(arguments[c]),d+=f*f;return Math.sqrt(d)}});
|
||||
q("Math.imul",function(a){return a?a:function(b,c){b=Number(b);c=Number(c);var d=b&65535,e=c&65535;return d*e+((b>>>16&65535)*e+d*(c>>>16&65535)<<16>>>0)|0}});q("Math.log10",function(a){return a?a:function(b){return Math.log(b)/Math.LN10}});q("Math.log2",function(a){return a?a:function(b){return Math.log(b)/Math.LN2}});q("Math.sign",function(a){return a?a:function(b){b=Number(b);return 0===b||isNaN(b)?b:0<b?1:-1}});
|
||||
q("Math.sinh",function(a){if(a)return a;var b=Math.exp;return function(c){c=Number(c);return 0===c?c:(b(c)-b(-c))/2}});q("Math.tanh",function(a){return a?a:function(b){b=Number(b);if(0===b)return b;var c=Math.exp(-2*Math.abs(b));c=(1-c)/(1+c);return 0>b?-c:c}});q("Math.trunc",function(a){return a?a:function(b){b=Number(b);if(isNaN(b)||Infinity===b||-Infinity===b||0===b)return b;var c=Math.floor(Math.abs(b));return 0>b?-c:c}});q("Number.EPSILON",function(){return Math.pow(2,-52)});
|
||||
q("Number.MAX_SAFE_INTEGER",function(){return 9007199254740991});q("Number.MIN_SAFE_INTEGER",function(){return-9007199254740991});q("Number.isFinite",function(a){return a?a:function(b){return"number"!==typeof b?!1:!isNaN(b)&&Infinity!==b&&-Infinity!==b}});q("Number.isInteger",function(a){return a?a:function(b){return Number.isFinite(b)?b===Math.floor(b):!1}});q("Number.isNaN",function(a){return a?a:function(b){return"number"===typeof b&&isNaN(b)}});
|
||||
q("Number.isSafeInteger",function(a){return a?a:function(b){return Number.isInteger(b)&&Math.abs(b)<=Number.MAX_SAFE_INTEGER}});q("Number.parseFloat",function(a){return a||parseFloat});q("Number.parseInt",function(a){return a||parseInt});var oa="function"==typeof Object.assign?Object.assign:function(a,b){for(var c=1;c<arguments.length;c++){var d=arguments[c];if(d)for(var e in d)t(d,e)&&(a[e]=d[e])}return a};q("Object.assign",function(a){return a||oa});
|
||||
q("Object.entries",function(a){return a?a:function(b){var c=[],d;for(d in b)t(b,d)&&c.push([d,b[d]]);return c}});q("Object.fromEntries",function(a){return a?a:function(b){var c={};if(!(Symbol.iterator in b))throw new TypeError(""+b+" is not iterable");b=b[Symbol.iterator].call(b);for(var d=b.next();!d.done;d=b.next()){d=d.value;if(Object(d)!==d)throw new TypeError("iterable for fromEntries should yield objects");c[d[0]]=d[1]}return c}});q("Reflect",function(a){return a?a:{}});
|
||||
q("Object.getOwnPropertySymbols",function(a){return a?a:function(){return[]}});q("Reflect.ownKeys",function(a){return a?a:function(b){var c=[],d=Object.getOwnPropertyNames(b);b=Object.getOwnPropertySymbols(b);for(var e=0;e<d.length;e++)("jscomp_symbol_"==d[e].substring(0,14)?b:c).push(d[e]);return c.concat(b)}});q("Object.getOwnPropertyDescriptors",function(a){return a?a:function(b){for(var c={},d=Reflect.ownKeys(b),e=0;e<d.length;e++)c[d[e]]=Object.getOwnPropertyDescriptor(b,d[e]);return c}});
|
||||
q("Object.setPrototypeOf",function(a){return a||na});q("Object.values",function(a){return a?a:function(b){var c=[],d;for(d in b)t(b,d)&&c.push(b[d]);return c}});q("Promise.allSettled",function(a){function b(d){return{status:"fulfilled",value:d}}function c(d){return{status:"rejected",reason:d}}return a?a:function(d){var e=this;d=Array.from(d,function(f){return e.resolve(f).then(b,c)});return e.all(d)}});
|
||||
q("Promise.prototype.finally",function(a){return a?a:function(b){return this.then(function(c){return Promise.resolve(b()).then(function(){return c})},function(c){return Promise.resolve(b()).then(function(){throw c;})})}});q("Reflect.apply",function(a){if(a)return a;var b=Function.prototype.apply;return function(c,d,e){return b.call(c,d,e)}});
|
||||
var pa="function"==typeof Object.create?Object.create:function(a){function b(){}b.prototype=a;return new b},qa=function(){function a(){function c(){}new c;Reflect.construct(c,[],function(){});return new c instanceof c}if("undefined"!=typeof Reflect&&Reflect.construct){if(a())return Reflect.construct;var b=Reflect.construct;return function(c,d,e){c=b(c,d);e&&Reflect.setPrototypeOf(c,e.prototype);return c}}return function(c,d,e){void 0===e&&(e=c);e=pa(e.prototype||Object.prototype);return Function.prototype.apply.call(c,
|
||||
e,d)||e}}();q("Reflect.construct",function(){return qa});q("Reflect.defineProperty",function(a){return a?a:function(b,c,d){try{Object.defineProperty(b,c,d);var e=Object.getOwnPropertyDescriptor(b,c);return e?e.configurable===(d.configurable||!1)&&e.enumerable===(d.enumerable||!1)&&("value"in e?e.value===d.value&&e.writable===(d.writable||!1):e.get===d.get&&e.set===d.set):!1}catch(f){return!1}}});q("Reflect.deleteProperty",function(a){return a?a:function(b,c){if(!t(b,c))return!0;try{return delete b[c]}catch(d){return!1}}});
|
||||
q("Reflect.getOwnPropertyDescriptor",function(a){return a||Object.getOwnPropertyDescriptor});q("Reflect.getPrototypeOf",function(a){return a||Object.getPrototypeOf});function ra(a,b){for(;a;){var c=Reflect.getOwnPropertyDescriptor(a,b);if(c)return c;a=Reflect.getPrototypeOf(a)}}q("Reflect.get",function(a){return a?a:function(b,c,d){if(2>=arguments.length)return b[c];var e=ra(b,c);if(e)return e.get?e.get.call(d):e.value}});q("Reflect.has",function(a){return a?a:function(b,c){return c in b}});
|
||||
q("Reflect.isExtensible",function(a){return a?a:"function"==typeof Object.isExtensible?Object.isExtensible:function(){return!0}});q("Reflect.preventExtensions",function(a){return a?a:"function"!=typeof Object.preventExtensions?function(){return!1}:function(b){Object.preventExtensions(b);return!Object.isExtensible(b)}});
|
||||
q("Reflect.set",function(a){return a?a:function(b,c,d,e){var f=ra(b,c);return f?f.set?(f.set.call(3<arguments.length?e:b,d),!0):f.writable&&!Object.isFrozen(b)?(b[c]=d,!0):!1:Reflect.isExtensible(b)?(b[c]=d,!0):!1}});q("Reflect.setPrototypeOf",function(a){return a?a:na?function(b,c){try{return na(b,c),!0}catch(d){return!1}}:null});
|
||||
q("Set",function(a){function b(c){this.a=new Map;if(c){c=n(c);for(var d;!(d=c.next()).done;)this.add(d.value)}this.size=this.a.size}if(function(){if(!a||"function"!=typeof a||!a.prototype.entries||"function"!=typeof Object.seal)return!1;try{var c=Object.seal({x:4}),d=new a(n([c]));if(!d.has(c)||1!=d.size||d.add(c)!=d||1!=d.size||d.add({x:4})!=d||2!=d.size)return!1;var e=d.entries(),f=e.next();if(f.done||f.value[0]!=c||f.value[1]!=c)return!1;f=e.next();return f.done||f.value[0]==c||4!=f.value[0].x||
|
||||
f.value[1]!=f.value[0]?!1:e.next().done}catch(g){return!1}}())return a;b.prototype.add=function(c){c=0===c?0:c;this.a.set(c,c);this.size=this.a.size;return this};b.prototype.delete=function(c){c=this.a.delete(c);this.size=this.a.size;return c};b.prototype.clear=function(){this.a.clear();this.size=0};b.prototype.has=function(c){return this.a.has(c)};b.prototype.entries=function(){return this.a.entries()};b.prototype.values=function(){return this.a.values()};b.prototype.keys=b.prototype.values;b.prototype[Symbol.iterator]=
|
||||
b.prototype.values;b.prototype.forEach=function(c,d){var e=this;this.a.forEach(function(f){return c.call(d,f,f,e)})};return b});q("String.prototype.codePointAt",function(a){return a?a:function(b){var c=r(this,null,"codePointAt"),d=c.length;b=Number(b)||0;if(0<=b&&b<d){b|=0;var e=c.charCodeAt(b);if(55296>e||56319<e||b+1===d)return e;b=c.charCodeAt(b+1);return 56320>b||57343<b?e:1024*(e-55296)+b+9216}}});
|
||||
q("String.fromCodePoint",function(a){return a?a:function(b){for(var c="",d=0;d<arguments.length;d++){var e=Number(arguments[d]);if(0>e||1114111<e||e!==Math.floor(e))throw new RangeError("invalid_code_point "+e);65535>=e?c+=String.fromCharCode(e):(e-=65536,c+=String.fromCharCode(e>>>10&1023|55296),c+=String.fromCharCode(e&1023|56320))}return c}});
|
||||
q("String.prototype.matchAll",function(a){return a?a:function(b){if(b instanceof RegExp&&!b.global)throw new TypeError("RegExp passed into String.prototype.matchAll() must have global tag.");var c=new RegExp(b,b instanceof RegExp?void 0:"g"),d=this,e=!1,f={next:function(){var g={},h=c.lastIndex;if(e)return{value:void 0,done:!0};var k=c.exec(d);if(!k)return e=!0,{value:void 0,done:!0};c.lastIndex===h&&(c.lastIndex+=1);g.value=k;g.done=!1;return g}};f[Symbol.iterator]=function(){return f};return f}});
|
||||
function sa(a,b){a=void 0!==a?String(a):" ";return 0<b&&a?a.repeat(Math.ceil(b/a.length)).substring(0,b):""}q("String.prototype.padEnd",function(a){return a?a:function(b,c){var d=r(this,null,"padStart");return d+sa(c,b-d.length)}});q("String.prototype.padStart",function(a){return a?a:function(b,c){var d=r(this,null,"padStart");return sa(c,b-d.length)+d}});q("String.prototype.trimRight",function(a){function b(){return this.replace(/[\s\xa0]+$/,"")}return a||b});
|
||||
q("String.prototype.trimEnd",function(a){return a||String.prototype.trimRight});function u(a){return a?a:Array.prototype.copyWithin}q("Int8Array.prototype.copyWithin",u);q("Uint8Array.prototype.copyWithin",u);q("Uint8ClampedArray.prototype.copyWithin",u);q("Int16Array.prototype.copyWithin",u);q("Uint16Array.prototype.copyWithin",u);q("Int32Array.prototype.copyWithin",u);q("Uint32Array.prototype.copyWithin",u);q("Float32Array.prototype.copyWithin",u);q("Float64Array.prototype.copyWithin",u);
|
||||
function v(a){return a?a:Array.prototype.fill}q("Int8Array.prototype.fill",v);q("Uint8Array.prototype.fill",v);q("Uint8ClampedArray.prototype.fill",v);q("Int16Array.prototype.fill",v);q("Uint16Array.prototype.fill",v);q("Int32Array.prototype.fill",v);q("Uint32Array.prototype.fill",v);q("Float32Array.prototype.fill",v);q("Float64Array.prototype.fill",v);
|
||||
q("WeakSet",function(a){function b(c){this.a=new WeakMap;if(c){c=n(c);for(var d;!(d=c.next()).done;)this.add(d.value)}}if(function(){if(!a||!Object.seal)return!1;try{var c=Object.seal({}),d=Object.seal({}),e=new a([c]);if(!e.has(c)||e.has(d))return!1;e.delete(c);e.add(d);return!e.has(c)&&e.has(d)}catch(f){return!1}}())return a;b.prototype.add=function(c){this.a.set(c,!0);return this};b.prototype.has=function(c){return this.a.has(c)};b.prototype.delete=function(c){return this.a.delete(c)};return b});
|
||||
var w=this||self,ta=/^[\w+/_-]+[=]{0,2}$/,ua=null;function va(a){return(a=a.querySelector&&a.querySelector("script[nonce]"))&&(a=a.nonce||a.getAttribute("nonce"))&&ta.test(a)?a:""}function y(a){a=a.split(".");for(var b=w,c=0;c<a.length;c++)if(b=b[a[c]],null==b)return null;return b}function z(){}function ya(a){var b=typeof a;return"object"!=b?b:a?Array.isArray(a)?"array":b:"null"}function B(a){return"function"==ya(a)}function za(a){var b=typeof a;return"object"==b&&null!=a||"function"==b}
|
||||
function Aa(a,b,c){return a.call.apply(a.bind,arguments)}function Ba(a,b,c){if(!a)throw Error();if(2<arguments.length){var d=Array.prototype.slice.call(arguments,2);return function(){var e=Array.prototype.slice.call(arguments);Array.prototype.unshift.apply(e,d);return a.apply(b,e)}}return function(){return a.apply(b,arguments)}}function C(a,b,c){Function.prototype.bind&&-1!=Function.prototype.bind.toString().indexOf("native code")?C=Aa:C=Ba;return C.apply(null,arguments)}
|
||||
function D(a,b){a=a.split(".");var c=w;a[0]in c||"undefined"==typeof c.execScript||c.execScript("var "+a[0]);for(var d;a.length&&(d=a.shift());)a.length||void 0===b?c[d]&&c[d]!==Object.prototype[d]?c=c[d]:c=c[d]={}:c[d]=b}function E(a,b){function c(){}c.prototype=b.prototype;a.prototype=new c;a.prototype.constructor=a}function Ca(a){return a};function F(a){if(Error.captureStackTrace)Error.captureStackTrace(this,F);else{var b=Error().stack;b&&(this.stack=b)}a&&(this.message=String(a))}E(F,Error);F.prototype.name="CustomError";function G(a,b){this.a=a===Da&&b||"";this.b=Ea}G.prototype.O=!0;G.prototype.M=function(){return this.a};function Fa(a){return a instanceof G&&a.constructor===G&&a.b===Ea?a.a:"type_error:Const"}function H(a){return new G(Da,a)}var Ea={},Da={};var J={f:{}};
|
||||
J.f.I={ha:{"gstatic.com":{loader:H("https://www.gstatic.com/charts/%{version}/loader.js"),debug:H("https://www.gstatic.com/charts/debug/%{version}/js/jsapi_debug_%{package}_module.js"),debug_i18n:H("https://www.gstatic.com/charts/debug/%{version}/i18n/jsapi_debug_i18n_%{package}_module__%{language}.js"),compiled:H("https://www.gstatic.com/charts/%{version}/js/jsapi_compiled_%{package}_module.js"),compiled_i18n:H("https://www.gstatic.com/charts/%{version}/i18n/jsapi_compiled_i18n_%{package}_module__%{language}.js"),css:H("https://www.gstatic.com/charts/%{version}/css/%{subdir}/%{filename}"),
|
||||
css2:H("https://www.gstatic.com/charts/%{version}/css/%{subdir1}/%{subdir2}/%{filename}"),third_party:H("https://www.gstatic.com/charts/%{version}/third_party/%{subdir}/%{filename}"),third_party2:H("https://www.gstatic.com/charts/%{version}/third_party/%{subdir1}/%{subdir2}/%{filename}"),third_party_gen:H("https://www.gstatic.com/charts/%{version}/third_party/%{subdir}/%{filename}")},"gstatic.cn":{loader:H("https://www.gstatic.cn/charts/%{version}/loader.js"),debug:H("https://www.gstatic.cn/charts/debug/%{version}/js/jsapi_debug_%{package}_module.js"),
|
||||
debug_i18n:H("https://www.gstatic.cn/charts/debug/%{version}/i18n/jsapi_debug_i18n_%{package}_module__%{language}.js"),compiled:H("https://www.gstatic.cn/charts/%{version}/js/jsapi_compiled_%{package}_module.js"),compiled_i18n:H("https://www.gstatic.cn/charts/%{version}/i18n/jsapi_compiled_i18n_%{package}_module__%{language}.js"),css:H("https://www.gstatic.cn/charts/%{version}/css/%{subdir}/%{filename}"),css2:H("https://www.gstatic.cn/charts/%{version}/css/%{subdir1}/%{subdir2}/%{filename}"),third_party:H("https://www.gstatic.cn/charts/%{version}/third_party/%{subdir}/%{filename}"),
|
||||
third_party2:H("https://www.gstatic.cn/charts/%{version}/third_party/%{subdir1}/%{subdir2}/%{filename}"),third_party_gen:H("https://www.gstatic.cn/charts/%{version}/third_party/%{subdir}/%{filename}")}},Y:["default"],na:{"default":[],graphics:["default"],ui:["graphics"],ui_base:["graphics"],flashui:["ui"],fw:["ui"],geo:["ui"],annotatedtimeline:["annotationchart"],annotationchart:["ui","controls","corechart","table"],areachart:"browserchart",bar:["fw","dygraph","webfontloader"],barchart:"browserchart",
|
||||
browserchart:["ui"],bubbles:["fw","d3"],calendar:["fw"],charteditor:"ui corechart imagechart annotatedtimeline gauge geochart motionchart orgchart table".split(" "),charteditor_base:"ui_base corechart imagechart annotatedtimeline gauge geochart motionchart orgchart table_base".split(" "),circles:["fw","d3"],clusterchart:["corechart","d3"],columnchart:"browserchart",controls:["ui"],controls_base:["ui_base"],corechart:["ui"],gantt:["fw","dygraph"],gauge:["ui"],geochart:["geo"],geomap:["flashui","geo"],
|
||||
geomap_base:["ui_base"],heatmap:["vegachart"],helloworld:["fw"],imagechart:["ui"],imageareachart:"imagechart",imagebarchart:"imagechart",imagelinechart:"imagechart",imagepiechart:"imagechart",imagesparkline:"imagechart",line:["fw","dygraph","webfontloader"],linechart:"browserchart",map:["geo"],motionchart:["flashui"],orgchart:["ui"],overtimecharts:["ui","corechart"],piechart:"browserchart",sankey:["fw","d3","d3.sankey"],scatter:["fw","dygraph","webfontloader"],scatterchart:"browserchart",sunburst:["fw",
|
||||
"d3"],streamgraph:["fw","d3"],table:["ui"],table_base:["ui_base"],timeline:["fw","ui","dygraph"],treemap:["ui"],vegachart:["graphics"],wordtree:["ui"]},Ha:{d3:{subdir1:"d3",subdir2:"v5",filename:"d3.js"},"d3.sankey":{subdir1:"d3_sankey",subdir2:"v4",filename:"d3.sankey.js"},webfontloader:{subdir:"webfontloader",filename:"webfont.js"}},Ga:{dygraph:{subdir:"dygraphs",filename:"dygraph-tickers-combined.js"}},ma:{"default":[{subdir:"core",filename:"tooltip.css"}],annotationchart:[{subdir:"annotationchart",
|
||||
filename:"annotationchart.css"}],charteditor:[{subdir:"charteditor",filename:"charteditor.css"}],charteditor_base:[{subdir:"charteditor_base",filename:"charteditor_base.css"}],controls:[{subdir:"controls",filename:"controls.css"}],imagesparkline:[{subdir:"imagechart",filename:"imagesparkline.css"}],orgchart:[{subdir:"orgchart",filename:"orgchart.css"}],table:[{subdir:"table",filename:"table.css"},{subdir:"util",filename:"format.css"}],table_base:[{subdir:"util",filename:"format.css"},{subdir:"table",
|
||||
filename:"table_base.css"}],ui:[{subdir:"util",filename:"util.css"}],ui_base:[{subdir:"util",filename:"util_base.css"}]}};J.f.V={$:{"chrome-frame":{versions:{"1.0.0":{uncompressed:"CFInstall.js",compressed:"CFInstall.min.js"},"1.0.1":{uncompressed:"CFInstall.js",compressed:"CFInstall.min.js"},"1.0.2":{uncompressed:"CFInstall.js",compressed:"CFInstall.min.js"}},aliases:{1:"1.0.2","1.0":"1.0.2"}},swfobject:{versions:{"2.1":{uncompressed:"swfobject_src.js",compressed:"swfobject.js"},"2.2":{uncompressed:"swfobject_src.js",compressed:"swfobject.js"}},aliases:{2:"2.2"}},"ext-core":{versions:{"3.1.0":{uncompressed:"ext-core-debug.js",
|
||||
compressed:"ext-core.js"},"3.0.0":{uncompressed:"ext-core-debug.js",compressed:"ext-core.js"}},aliases:{3:"3.1.0","3.0":"3.0.0","3.1":"3.1.0"}},scriptaculous:{versions:{"1.8.3":{uncompressed:"scriptaculous.js",compressed:"scriptaculous.js"},"1.9.0":{uncompressed:"scriptaculous.js",compressed:"scriptaculous.js"},"1.8.1":{uncompressed:"scriptaculous.js",compressed:"scriptaculous.js"},"1.8.2":{uncompressed:"scriptaculous.js",compressed:"scriptaculous.js"}},aliases:{1:"1.9.0","1.8":"1.8.3","1.9":"1.9.0"}},
|
||||
webfont:{versions:{"1.0.12":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.13":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.14":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.15":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.10":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.11":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.27":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.28":{uncompressed:"webfont_debug.js",
|
||||
compressed:"webfont.js"},"1.0.29":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.23":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.24":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.25":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.26":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.21":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.22":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.3":{uncompressed:"webfont_debug.js",
|
||||
compressed:"webfont.js"},"1.0.4":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.5":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.6":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.9":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.16":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.17":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.0":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.18":{uncompressed:"webfont_debug.js",
|
||||
compressed:"webfont.js"},"1.0.1":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.19":{uncompressed:"webfont_debug.js",compressed:"webfont.js"},"1.0.2":{uncompressed:"webfont_debug.js",compressed:"webfont.js"}},aliases:{1:"1.0.29","1.0":"1.0.29"}},jqueryui:{versions:{"1.8.17":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.16":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.15":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.14":{uncompressed:"jquery-ui.js",
|
||||
compressed:"jquery-ui.min.js"},"1.8.4":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.13":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.5":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.12":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.6":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.11":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.7":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},
|
||||
"1.8.10":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.8":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.9":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.6.0":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.7.0":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.5.2":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.0":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.7.1":{uncompressed:"jquery-ui.js",
|
||||
compressed:"jquery-ui.min.js"},"1.5.3":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.1":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.7.2":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.8.2":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"},"1.7.3":{uncompressed:"jquery-ui.js",compressed:"jquery-ui.min.js"}},aliases:{1:"1.8.17","1.5":"1.5.3","1.6":"1.6.0","1.7":"1.7.3","1.8":"1.8.17","1.8.3":"1.8.4"}},mootools:{versions:{"1.3.0":{uncompressed:"mootools.js",
|
||||
compressed:"mootools-yui-compressed.js"},"1.2.1":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.1.2":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.4.0":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.3.1":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.2.2":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.4.1":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},
|
||||
"1.3.2":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.2.3":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.4.2":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.2.4":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.2.5":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"},"1.1.1":{uncompressed:"mootools.js",compressed:"mootools-yui-compressed.js"}},aliases:{1:"1.1.2","1.1":"1.1.2","1.2":"1.2.5",
|
||||
"1.3":"1.3.2","1.4":"1.4.2","1.11":"1.1.1"}},yui:{versions:{"2.8.0r4":{uncompressed:"build/yuiloader/yuiloader.js",compressed:"build/yuiloader/yuiloader-min.js"},"2.9.0":{uncompressed:"build/yuiloader/yuiloader.js",compressed:"build/yuiloader/yuiloader-min.js"},"2.8.1":{uncompressed:"build/yuiloader/yuiloader.js",compressed:"build/yuiloader/yuiloader-min.js"},"2.6.0":{uncompressed:"build/yuiloader/yuiloader.js",compressed:"build/yuiloader/yuiloader-min.js"},"2.7.0":{uncompressed:"build/yuiloader/yuiloader.js",
|
||||
compressed:"build/yuiloader/yuiloader-min.js"},"3.3.0":{uncompressed:"build/yui/yui.js",compressed:"build/yui/yui-min.js"},"2.8.2r1":{uncompressed:"build/yuiloader/yuiloader.js",compressed:"build/yuiloader/yuiloader-min.js"}},aliases:{2:"2.9.0","2.6":"2.6.0","2.7":"2.7.0","2.8":"2.8.2r1","2.8.0":"2.8.0r4","2.8.2":"2.8.2r1","2.9":"2.9.0",3:"3.3.0","3.3":"3.3.0"}},prototype:{versions:{"1.6.1.0":{uncompressed:"prototype.js",compressed:"prototype.js"},"1.6.0.2":{uncompressed:"prototype.js",compressed:"prototype.js"},
|
||||
"1.7.0.0":{uncompressed:"prototype.js",compressed:"prototype.js"},"1.6.0.3":{uncompressed:"prototype.js",compressed:"prototype.js"}},aliases:{1:"1.7.0.0","1.6":"1.6.1.0","1.6.0":"1.6.0.3","1.6.1":"1.6.1.0","1.7":"1.7.0.0","1.7.0":"1.7.0.0"}},jquery:{versions:{"1.2.3":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.2.6":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.3.0":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.3.1":{uncompressed:"jquery.js",compressed:"jquery.min.js"},
|
||||
"1.3.2":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.4.0":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.4.1":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.4.2":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.4.3":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.4.4":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.5.0":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.5.1":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.5.2":{uncompressed:"jquery.js",
|
||||
compressed:"jquery.min.js"},"1.6.0":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.6.1":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.6.2":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.6.3":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.6.4":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.7.0":{uncompressed:"jquery.js",compressed:"jquery.min.js"},"1.7.1":{uncompressed:"jquery.js",compressed:"jquery.min.js"}},aliases:{1:"1.7.1","1.2":"1.2.6","1.3":"1.3.2",
|
||||
"1.4":"1.4.4","1.5":"1.5.2","1.6":"1.6.4","1.7":"1.7.1"}},dojo:{versions:{"1.3.0":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.4.0":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.3.1":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.5.0":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.4.1":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},
|
||||
"1.3.2":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.2.3":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.6.0":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.5.1":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.7.0":{uncompressed:"dojo/dojo.js.uncompressed.js",compressed:"dojo/dojo.js"},"1.6.1":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},
|
||||
"1.4.3":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.7.1":{uncompressed:"dojo/dojo.js.uncompressed.js",compressed:"dojo/dojo.js"},"1.7.2":{uncompressed:"dojo/dojo.js.uncompressed.js",compressed:"dojo/dojo.js"},"1.2.0":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"},"1.1.1":{uncompressed:"dojo/dojo.xd.js.uncompressed.js",compressed:"dojo/dojo.xd.js"}},aliases:{1:"1.6.1","1.1":"1.1.1","1.2":"1.2.3","1.3":"1.3.2","1.4":"1.4.3","1.5":"1.5.1",
|
||||
"1.6":"1.6.1","1.7":"1.7.2"}}}};J.f.W={af:!0,am:!0,az:!0,ar:!0,arb:"ar",bg:!0,bn:!0,ca:!0,cs:!0,cmn:"zh",da:!0,de:!0,el:!0,en:!0,en_gb:!0,es:!0,es_419:!0,et:!0,eu:!0,fa:!0,fi:!0,fil:!0,fr:!0,fr_ca:!0,gl:!0,ka:!0,gu:!0,he:"iw",hi:!0,hr:!0,hu:!0,hy:!0,id:!0,"in":"id",is:!0,it:!0,iw:!0,ja:!0,ji:"yi",jv:!1,jw:"jv",km:!0,kn:!0,ko:!0,lo:!0,lt:!0,lv:!0,ml:!0,mn:!0,mo:"ro",mr:!0,ms:!0,nb:"no",ne:!0,nl:!0,no:!0,pl:!0,pt:"pt_br",pt_br:!0,pt_pt:!0,ro:!0,ru:!0,si:!0,sk:!0,sl:!0,sr:!0,sv:!0,sw:!0,swh:"sw",ta:!0,te:!0,th:!0,tl:"fil",tr:!0,uk:!0,
|
||||
ur:!0,vi:!0,yi:!1,zh:"zh_cn",zh_cn:!0,zh_hk:!0,zh_tw:!0,zsm:"ms",zu:!0};var Ga=Array.prototype.forEach?function(a,b,c){Array.prototype.forEach.call(a,b,c)}:function(a,b,c){for(var d=a.length,e="string"===typeof a?a.split(""):a,f=0;f<d;f++)f in e&&b.call(c,e[f],f,a)},Ha=Array.prototype.map?function(a,b){return Array.prototype.map.call(a,b,void 0)}:function(a,b){for(var c=a.length,d=Array(c),e="string"===typeof a?a.split(""):a,f=0;f<c;f++)f in e&&(d[f]=b.call(void 0,e[f],f,a));return d},Ia=Array.prototype.some?function(a,b){return Array.prototype.some.call(a,b,void 0)}:
|
||||
function(a,b){for(var c=a.length,d="string"===typeof a?a.split(""):a,e=0;e<c;e++)if(e in d&&b.call(void 0,d[e],e,a))return!0;return!1};function Ja(a){return Array.prototype.concat.apply([],arguments)}function Ka(a){var b=a.length;if(0<b){for(var c=Array(b),d=0;d<b;d++)c[d]=a[d];return c}return[]}
|
||||
function La(a,b){for(var c=1;c<arguments.length;c++){var d=arguments[c],e=ya(d);if("array"==e||"object"==e&&"number"==typeof d.length){e=a.length||0;var f=d.length||0;a.length=e+f;for(var g=0;g<f;g++)a[e+g]=d[g]}else a.push(d)}};var Ma;function K(a,b){this.a=a===Na&&b||"";this.b=Oa}K.prototype.O=!0;K.prototype.M=function(){return this.a.toString()};function Pa(a){return a instanceof K&&a.constructor===K&&a.b===Oa?a.a:"type_error:TrustedResourceUrl"}
|
||||
function Qa(a,b){var c=Fa(a);if(!Ra.test(c))throw Error("Invalid TrustedResourceUrl format: "+c);a=c.replace(Sa,function(d,e){if(!Object.prototype.hasOwnProperty.call(b,e))throw Error('Found marker, "'+e+'", in format string, "'+c+'", but no valid label mapping found in args: '+JSON.stringify(b));d=b[e];return d instanceof G?Fa(d):encodeURIComponent(String(d))});return Ta(a)}var Sa=/%{(\w+)}/g,Ra=/^((https:)?\/\/[0-9a-z.:[\]-]+\/|\/[^/\\]|[^:/\\%]+\/|[^:/\\%]*[?#]|about:blank#)/i,Ua=/^([^?#]*)(\?[^#]*)?(#[\s\S]*)?/;
|
||||
function Va(a,b,c){a=Qa(a,b);a=Ua.exec(Pa(a).toString());b=a[3]||"";return Ta(a[1]+Wa("?",a[2]||"",c)+Wa("#",b,void 0))}var Oa={};function Ta(a){if(void 0===Ma){var b=null;var c=w.trustedTypes;if(c&&c.createPolicy){try{b=c.createPolicy("goog#html",{createHTML:Ca,createScript:Ca,createScriptURL:Ca})}catch(d){w.console&&w.console.error(d.message)}Ma=b}else Ma=b}a=(b=Ma)?b.createScriptURL(a):a;return new K(Na,a)}
|
||||
function Wa(a,b,c){if(null==c)return b;if("string"===typeof c)return c?a+encodeURIComponent(c):"";for(var d in c)if(Object.prototype.hasOwnProperty.call(c,d)){var e=c[d];e=Array.isArray(e)?e:[e];for(var f=0;f<e.length;f++){var g=e[f];null!=g&&(b||(b=a),b+=(b.length>a.length?"&":"")+encodeURIComponent(d)+"="+encodeURIComponent(String(g)))}}return b}var Na={};var Xa=String.prototype.trim?function(a){return a.trim()}:function(a){return/^[\s\xa0]*([\s\S]*?)[\s\xa0]*$/.exec(a)[1]};function Ya(a,b){return a<b?-1:a>b?1:0};var L;a:{var Za=w.navigator;if(Za){var $a=Za.userAgent;if($a){L=$a;break a}}L=""}function M(a){return-1!=L.indexOf(a)};function ab(a,b){for(var c in a)b.call(void 0,a[c],c,a)}var bb="constructor hasOwnProperty isPrototypeOf propertyIsEnumerable toLocaleString toString valueOf".split(" ");function cb(a,b){for(var c,d,e=1;e<arguments.length;e++){d=arguments[e];for(c in d)a[c]=d[c];for(var f=0;f<bb.length;f++)c=bb[f],Object.prototype.hasOwnProperty.call(d,c)&&(a[c]=d[c])}};function db(a,b){a.src=Pa(b);(b=a.ownerDocument&&a.ownerDocument.defaultView)&&b!=w?b=va(b.document):(null===ua&&(ua=va(w.document)),b=ua);b&&a.setAttribute("nonce",b)};function eb(a){var b=fb;return Object.prototype.hasOwnProperty.call(b,11)?b[11]:b[11]=a(11)};var gb=M("Opera"),hb=M("Trident")||M("MSIE"),ib=M("Edge"),jb=M("Gecko")&&!(-1!=L.toLowerCase().indexOf("webkit")&&!M("Edge"))&&!(M("Trident")||M("MSIE"))&&!M("Edge"),kb=-1!=L.toLowerCase().indexOf("webkit")&&!M("Edge"),lb;
|
||||
a:{var mb="",nb=function(){var a=L;if(jb)return/rv:([^\);]+)(\)|;)/.exec(a);if(ib)return/Edge\/([\d\.]+)/.exec(a);if(hb)return/\b(?:MSIE|rv)[: ]([^\);]+)(\)|;)/.exec(a);if(kb)return/WebKit\/(\S+)/.exec(a);if(gb)return/(?:Version)[ \/]?(\S+)/.exec(a)}();nb&&(mb=nb?nb[1]:"");if(hb){var ob,pb=w.document;ob=pb?pb.documentMode:void 0;if(null!=ob&&ob>parseFloat(mb)){lb=String(ob);break a}}lb=mb}var qb=lb,fb={};
|
||||
function rb(){return eb(function(){for(var a=0,b=Xa(String(qb)).split("."),c=Xa("11").split("."),d=Math.max(b.length,c.length),e=0;0==a&&e<d;e++){var f=b[e]||"",g=c[e]||"";do{f=/(\d*)(\D*)(.*)/.exec(f)||["","","",""];g=/(\d*)(\D*)(.*)/.exec(g)||["","","",""];if(0==f[0].length&&0==g[0].length)break;a=Ya(0==f[1].length?0:parseInt(f[1],10),0==g[1].length?0:parseInt(g[1],10))||Ya(0==f[2].length,0==g[2].length)||Ya(f[2],g[2]);f=f[3];g=g[3]}while(0==a)}return 0<=a})};function sb(a,b){ab(b,function(c,d){c&&"object"==typeof c&&c.O&&(c=c.M());"style"==d?a.style.cssText=c:"class"==d?a.className=c:"for"==d?a.htmlFor=c:tb.hasOwnProperty(d)?a.setAttribute(tb[d],c):0==d.lastIndexOf("aria-",0)||0==d.lastIndexOf("data-",0)?a.setAttribute(d,c):a[d]=c})}
|
||||
var tb={cellpadding:"cellPadding",cellspacing:"cellSpacing",colspan:"colSpan",frameborder:"frameBorder",height:"height",maxlength:"maxLength",nonce:"nonce",role:"role",rowspan:"rowSpan",type:"type",usemap:"useMap",valign:"vAlign",width:"width"};function ub(a){var b=document;a=String(a);"application/xhtml+xml"===b.contentType&&(a=a.toLowerCase());return b.createElement(a)};function vb(a,b){this.c=a;this.g=b;this.b=0;this.a=null}vb.prototype.get=function(){if(0<this.b){this.b--;var a=this.a;this.a=a.next;a.next=null}else a=this.c();return a};function wb(a,b){a.g(b);100>a.b&&(a.b++,b.next=a.a,a.a=b)};function xb(a){w.setTimeout(function(){throw a;},0)}var yb;
|
||||
function zb(){var a=w.MessageChannel;"undefined"===typeof a&&"undefined"!==typeof window&&window.postMessage&&window.addEventListener&&!M("Presto")&&(a=function(){var e=ub("IFRAME");e.style.display="none";document.documentElement.appendChild(e);var f=e.contentWindow;e=f.document;e.open();e.close();var g="callImmediate"+Math.random(),h="file:"==f.location.protocol?"*":f.location.protocol+"//"+f.location.host;e=C(function(k){if(("*"==h||k.origin==h)&&k.data==g)this.port1.onmessage()},this);f.addEventListener("message",
|
||||
e,!1);this.port1={};this.port2={postMessage:function(){f.postMessage(g,h)}}});if("undefined"!==typeof a&&!M("Trident")&&!M("MSIE")){var b=new a,c={},d=c;b.port1.onmessage=function(){if(void 0!==c.next){c=c.next;var e=c.L;c.L=null;e()}};return function(e){d.next={L:e};d=d.next;b.port2.postMessage(0)}}return function(e){w.setTimeout(e,0)}};function Ab(){this.b=this.a=null}var Cb=new vb(function(){return new Bb},function(a){a.reset()});Ab.prototype.add=function(a,b){var c=Cb.get();c.set(a,b);this.b?this.b.next=c:this.a=c;this.b=c};function Db(){var a=Eb,b=null;a.a&&(b=a.a,a.a=a.a.next,a.a||(a.b=null),b.next=null);return b}function Bb(){this.next=this.b=this.a=null}Bb.prototype.set=function(a,b){this.a=a;this.b=b;this.next=null};Bb.prototype.reset=function(){this.next=this.b=this.a=null};function Fb(a,b){Gb||Hb();Ib||(Gb(),Ib=!0);Eb.add(a,b)}var Gb;function Hb(){if(w.Promise&&w.Promise.resolve){var a=w.Promise.resolve(void 0);Gb=function(){a.then(Jb)}}else Gb=function(){var b=Jb;!B(w.setImmediate)||w.Window&&w.Window.prototype&&!M("Edge")&&w.Window.prototype.setImmediate==w.setImmediate?(yb||(yb=zb()),yb(b)):w.setImmediate(b)}}var Ib=!1,Eb=new Ab;function Jb(){for(var a;a=Db();){try{a.a.call(a.b)}catch(b){xb(b)}wb(Cb,a)}Ib=!1};function Kb(a){if(!a)return!1;try{return!!a.$goog_Thenable}catch(b){return!1}};function N(a){this.a=0;this.j=void 0;this.g=this.b=this.c=null;this.h=this.i=!1;if(a!=z)try{var b=this;a.call(void 0,function(c){O(b,2,c)},function(c){O(b,3,c)})}catch(c){O(this,3,c)}}function Lb(){this.next=this.c=this.b=this.g=this.a=null;this.h=!1}Lb.prototype.reset=function(){this.c=this.b=this.g=this.a=null;this.h=!1};var Mb=new vb(function(){return new Lb},function(a){a.reset()});function Nb(a,b,c){var d=Mb.get();d.g=a;d.b=b;d.c=c;return d}
|
||||
N.prototype.then=function(a,b,c){return Ob(this,B(a)?a:null,B(b)?b:null,c)};N.prototype.$goog_Thenable=!0;N.prototype.cancel=function(a){if(0==this.a){var b=new P(a);Fb(function(){Pb(this,b)},this)}};function Pb(a,b){if(0==a.a)if(a.c){var c=a.c;if(c.b){for(var d=0,e=null,f=null,g=c.b;g&&(g.h||(d++,g.a==a&&(e=g),!(e&&1<d)));g=g.next)e||(f=g);e&&(0==c.a&&1==d?Pb(c,b):(f?(d=f,d.next==c.g&&(c.g=d),d.next=d.next.next):Qb(c),Rb(c,e,3,b)))}a.c=null}else O(a,3,b)}
|
||||
function Sb(a,b){a.b||2!=a.a&&3!=a.a||Tb(a);a.g?a.g.next=b:a.b=b;a.g=b}function Ob(a,b,c,d){var e=Nb(null,null,null);e.a=new N(function(f,g){e.g=b?function(h){try{var k=b.call(d,h);f(k)}catch(m){g(m)}}:f;e.b=c?function(h){try{var k=c.call(d,h);void 0===k&&h instanceof P?g(h):f(k)}catch(m){g(m)}}:g});e.a.c=a;Sb(a,e);return e.a}N.prototype.A=function(a){this.a=0;O(this,2,a)};N.prototype.B=function(a){this.a=0;O(this,3,a)};
|
||||
function O(a,b,c){if(0==a.a){a===c&&(b=3,c=new TypeError("Promise cannot resolve to itself"));a.a=1;a:{var d=c,e=a.A,f=a.B;if(d instanceof N){Sb(d,Nb(e||z,f||null,a));var g=!0}else if(Kb(d))d.then(e,f,a),g=!0;else{if(za(d))try{var h=d.then;if(B(h)){Ub(d,h,e,f,a);g=!0;break a}}catch(k){f.call(a,k);g=!0;break a}g=!1}}g||(a.j=c,a.a=b,a.c=null,Tb(a),3!=b||c instanceof P||Vb(a,c))}}
|
||||
function Ub(a,b,c,d,e){function f(k){h||(h=!0,d.call(e,k))}function g(k){h||(h=!0,c.call(e,k))}var h=!1;try{b.call(a,g,f)}catch(k){f(k)}}function Tb(a){a.i||(a.i=!0,Fb(a.m,a))}function Qb(a){var b=null;a.b&&(b=a.b,a.b=b.next,b.next=null);a.b||(a.g=null);return b}N.prototype.m=function(){for(var a;a=Qb(this);)Rb(this,a,this.a,this.j);this.i=!1};
|
||||
function Rb(a,b,c,d){if(3==c&&b.b&&!b.h)for(;a&&a.h;a=a.c)a.h=!1;if(b.a)b.a.c=null,Wb(b,c,d);else try{b.h?b.g.call(b.c):Wb(b,c,d)}catch(e){Xb.call(null,e)}wb(Mb,b)}function Wb(a,b,c){2==b?a.g.call(a.c,c):a.b&&a.b.call(a.c,c)}function Vb(a,b){a.h=!0;Fb(function(){a.h&&Xb.call(null,b)})}var Xb=xb;function P(a){F.call(this,a)}E(P,F);P.prototype.name="cancel";/*
|
||||
Portions of this code are from MochiKit, received by
|
||||
The Closure Authors under the MIT license. All other code is Copyright
|
||||
2005-2009 The Closure Authors. All Rights Reserved.
|
||||
*/
|
||||
function Q(a,b){this.h=[];this.F=a;this.H=b||null;this.g=this.a=!1;this.c=void 0;this.A=this.U=this.j=!1;this.i=0;this.b=null;this.m=0}Q.prototype.cancel=function(a){if(this.a)this.c instanceof Q&&this.c.cancel();else{if(this.b){var b=this.b;delete this.b;a?b.cancel(a):(b.m--,0>=b.m&&b.cancel())}this.F?this.F.call(this.H,this):this.A=!0;this.a||(a=new Yb(this),Zb(this),R(this,!1,a))}};Q.prototype.B=function(a,b){this.j=!1;R(this,a,b)};function R(a,b,c){a.a=!0;a.c=c;a.g=!b;$b(a)}
|
||||
function Zb(a){if(a.a){if(!a.A)throw new ac(a);a.A=!1}}function bc(a,b,c,d){a.h.push([b,c,d]);a.a&&$b(a);return a}Q.prototype.then=function(a,b,c){var d,e,f=new N(function(g,h){d=g;e=h});bc(this,d,function(g){g instanceof Yb?f.cancel():e(g)});return f.then(a,b,c)};Q.prototype.$goog_Thenable=!0;function cc(a){return Ia(a.h,function(b){return B(b[1])})}
|
||||
function $b(a){if(a.i&&a.a&&cc(a)){var b=a.i,c=dc[b];c&&(w.clearTimeout(c.a),delete dc[b]);a.i=0}a.b&&(a.b.m--,delete a.b);b=a.c;for(var d=c=!1;a.h.length&&!a.j;){var e=a.h.shift(),f=e[0],g=e[1];e=e[2];if(f=a.g?g:f)try{var h=f.call(e||a.H,b);void 0!==h&&(a.g=a.g&&(h==b||h instanceof Error),a.c=b=h);if(Kb(b)||"function"===typeof w.Promise&&b instanceof w.Promise)d=!0,a.j=!0}catch(k){b=k,a.g=!0,cc(a)||(c=!0)}}a.c=b;d&&(h=C(a.B,a,!0),d=C(a.B,a,!1),b instanceof Q?(bc(b,h,d),b.U=!0):b.then(h,d));c&&(b=
|
||||
new ec(b),dc[b.a]=b,a.i=b.a)}function fc(){var a=new Q;Zb(a);R(a,!0,null);return a}function ac(){F.call(this)}E(ac,F);ac.prototype.message="Deferred has already fired";ac.prototype.name="AlreadyCalledError";function Yb(){F.call(this)}E(Yb,F);Yb.prototype.message="Deferred was canceled";Yb.prototype.name="CanceledError";function ec(a){this.a=w.setTimeout(C(this.c,this),0);this.b=a}ec.prototype.c=function(){delete dc[this.a];throw this.b;};var dc={};var gc,hc=[];function ic(a,b){function c(){var e=a.shift();e=jc(e,b);a.length&&bc(e,c,c,void 0);return e}if(!a.length)return fc();var d=hc.length;La(hc,a);if(d)return gc;a=hc;return gc=c()}
|
||||
function jc(a,b){var c=b||{};b=c.document||document;var d=Pa(a).toString(),e=ub("SCRIPT"),f={P:e,S:void 0},g=new Q(kc,f),h=null,k=null!=c.timeout?c.timeout:5E3;0<k&&(h=window.setTimeout(function(){lc(e,!0);var m=new mc(1,"Timeout reached for loading script "+d);Zb(g);R(g,!1,m)},k),f.S=h);e.onload=e.onreadystatechange=function(){e.readyState&&"loaded"!=e.readyState&&"complete"!=e.readyState||(lc(e,c.la||!1,h),Zb(g),R(g,!0,null))};e.onerror=function(){lc(e,!0,h);var m=new mc(0,"Error while loading script "+
|
||||
d);Zb(g);R(g,!1,m)};f=c.attributes||{};cb(f,{type:"text/javascript",charset:"UTF-8"});sb(e,f);db(e,a);nc(b).appendChild(e);return g}function nc(a){var b;return(b=(a||document).getElementsByTagName("HEAD"))&&0!=b.length?b[0]:a.documentElement}function kc(){if(this&&this.P){var a=this.P;a&&"SCRIPT"==a.tagName&&lc(a,!0,this.S)}}function lc(a,b,c){null!=c&&w.clearTimeout(c);a.onload=z;a.onerror=z;a.onreadystatechange=z;b&&window.setTimeout(function(){a&&a.parentNode&&a.parentNode.removeChild(a)},0)}
|
||||
function mc(a,b){var c="Jsloader error (code #"+a+")";b&&(c+=": "+b);F.call(this,c);this.code=a}E(mc,F);J.f.o={};var oc=jc,qc=pc;function rc(a){return Va(a.format,a.K,a.ea||{})}function pc(a,b,c){c=c||{};a=Va(a,b,c);var d=oc(a,{timeout:3E4,attributes:{async:!1,defer:!1}});return new Promise(function(e){bc(d,e,null,void 0)})}J.f.o.Ca=function(a){pc=a};J.f.o.Fa=function(a){oc=a};J.f.o.Z=rc;J.f.o.load=qc;
|
||||
J.f.o.ua=function(a){a=Ha(a,rc);if(0==a.length)return Promise.resolve();var b={timeout:3E4,attributes:{async:!1,defer:!1}},c=[];!hb||rb()?Ga(a,function(d){c.push(oc(d,b))}):c.push(ic(a,b));return Promise.all(Ha(c,function(d){return new Promise(function(e){return bc(d,e,null,void 0)})}))};J.f.o.wa=function(a,b,c){return{format:a,K:b,ea:c}};J.f.v={};var S={};J.f.v.oa=function(a){return S[a]&&S[a].loaded};J.f.v.pa=function(a){return S[a]&&S[a].ga};J.f.v.aa=function(){return new Promise(function(a){"undefined"==typeof window||"complete"===document.readyState?a():window.addEventListener?(document.addEventListener("DOMContentLoaded",a,!0),window.addEventListener("load",a,!0)):window.attachEvent?window.attachEvent("onload",a):"function"!==typeof window.onload?window.onload=a:window.onload=function(b){window.onload(b);a()}})};J.f.v.va=S;
|
||||
J.f.v.Ba=function(){S={}};J.f.v.Da=function(a){S[a]||(S[a]={loaded:!1});S[a].loaded=!0};J.f.v.Ea=function(a,b){S[a]={ga:b,loaded:!1}};J.f.J={1:"1.0","1.0":"current","1.1":"upcoming","1.2":"testing",41:"pre-45",42:"pre-45",43:"pre-45",44:"pre-45",46:"46.1","46.1":"46.2",48:"48.1",current:"49",upcoming:"49",testing:"49"};function sc(a,b){this.b={};this.a=[];this.c=0;var c=arguments.length;if(1<c){if(c%2)throw Error("Uneven number of arguments");for(var d=0;d<c;d+=2)this.set(arguments[d],arguments[d+1])}else if(a)if(a instanceof sc)for(c=a.C(),d=0;d<c.length;d++)this.set(c[d],a.get(c[d]));else for(d in a)this.set(d,a[d])}l=sc.prototype;l.D=function(){tc(this);for(var a=[],b=0;b<this.a.length;b++)a.push(this.b[this.a[b]]);return a};l.C=function(){tc(this);return this.a.concat()};
|
||||
function tc(a){if(a.c!=a.a.length){for(var b=0,c=0;b<a.a.length;){var d=a.a[b];T(a.b,d)&&(a.a[c++]=d);b++}a.a.length=c}if(a.c!=a.a.length){var e={};for(c=b=0;b<a.a.length;)d=a.a[b],T(e,d)||(a.a[c++]=d,e[d]=1),b++;a.a.length=c}}l.get=function(a,b){return T(this.b,a)?this.b[a]:b};l.set=function(a,b){T(this.b,a)||(this.c++,this.a.push(a));this.b[a]=b};l.forEach=function(a,b){for(var c=this.C(),d=0;d<c.length;d++){var e=c[d],f=this.get(e);a.call(b,f,e,this)}};
|
||||
function T(a,b){return Object.prototype.hasOwnProperty.call(a,b)};var uc=/^(?:([^:/?#.]+):)?(?:\/\/(?:([^\\/?#]*)@)?([^\\/?#]*?)(?::([0-9]+))?(?=[\\/?#]|$))?([^?#]+)?(?:\?([^#]*))?(?:#([\s\S]*))?$/;function vc(a,b){if(a){a=a.split("&");for(var c=0;c<a.length;c++){var d=a[c].indexOf("="),e=null;if(0<=d){var f=a[c].substring(0,d);e=a[c].substring(d+1)}else f=a[c];b(f,e?decodeURIComponent(e.replace(/\+/g," ")):"")}}};function wc(a){this.a=this.j=this.g="";this.m=null;this.h=this.b="";this.i=!1;var b;a instanceof wc?(this.i=a.i,xc(this,a.g),this.j=a.j,this.a=a.a,yc(this,a.m),this.b=a.b,zc(this,Ac(a.c)),this.h=a.h):a&&(b=String(a).match(uc))?(this.i=!1,xc(this,b[1]||"",!0),this.j=Bc(b[2]||""),this.a=Bc(b[3]||"",!0),yc(this,b[4]),this.b=Bc(b[5]||"",!0),zc(this,b[6]||"",!0),this.h=Bc(b[7]||"")):(this.i=!1,this.c=new U(null,this.i))}
|
||||
wc.prototype.toString=function(){var a=[],b=this.g;b&&a.push(Cc(b,Dc,!0),":");var c=this.a;if(c||"file"==b)a.push("//"),(b=this.j)&&a.push(Cc(b,Dc,!0),"@"),a.push(encodeURIComponent(String(c)).replace(/%25([0-9a-fA-F]{2})/g,"%$1")),c=this.m,null!=c&&a.push(":",String(c));if(c=this.b)this.a&&"/"!=c.charAt(0)&&a.push("/"),a.push(Cc(c,"/"==c.charAt(0)?Ec:Fc,!0));(c=this.c.toString())&&a.push("?",c);(c=this.h)&&a.push("#",Cc(c,Gc));return a.join("")};
|
||||
wc.prototype.resolve=function(a){var b=new wc(this),c=!!a.g;c?xc(b,a.g):c=!!a.j;c?b.j=a.j:c=!!a.a;c?b.a=a.a:c=null!=a.m;var d=a.b;if(c)yc(b,a.m);else if(c=!!a.b){if("/"!=d.charAt(0))if(this.a&&!this.b)d="/"+d;else{var e=b.b.lastIndexOf("/");-1!=e&&(d=b.b.substr(0,e+1)+d)}e=d;if(".."==e||"."==e)d="";else if(-1!=e.indexOf("./")||-1!=e.indexOf("/.")){d=0==e.lastIndexOf("/",0);e=e.split("/");for(var f=[],g=0;g<e.length;){var h=e[g++];"."==h?d&&g==e.length&&f.push(""):".."==h?((1<f.length||1==f.length&&
|
||||
""!=f[0])&&f.pop(),d&&g==e.length&&f.push("")):(f.push(h),d=!0)}d=f.join("/")}else d=e}c?b.b=d:c=""!==a.c.toString();c?zc(b,Ac(a.c)):c=!!a.h;c&&(b.h=a.h);return b};function xc(a,b,c){a.g=c?Bc(b,!0):b;a.g&&(a.g=a.g.replace(/:$/,""))}function yc(a,b){if(b){b=Number(b);if(isNaN(b)||0>b)throw Error("Bad port number "+b);a.m=b}else a.m=null}function zc(a,b,c){b instanceof U?(a.c=b,Hc(a.c,a.i)):(c||(b=Cc(b,Ic)),a.c=new U(b,a.i))}
|
||||
function Bc(a,b){return a?b?decodeURI(a.replace(/%25/g,"%2525")):decodeURIComponent(a):""}function Cc(a,b,c){return"string"===typeof a?(a=encodeURI(a).replace(b,Jc),c&&(a=a.replace(/%25([0-9a-fA-F]{2})/g,"%$1")),a):null}function Jc(a){a=a.charCodeAt(0);return"%"+(a>>4&15).toString(16)+(a&15).toString(16)}var Dc=/[#\/\?@]/g,Fc=/[#\?:]/g,Ec=/[#\?]/g,Ic=/[#\?@]/g,Gc=/#/g;function U(a,b){this.b=this.a=null;this.c=a||null;this.g=!!b}
|
||||
function V(a){a.a||(a.a=new sc,a.b=0,a.c&&vc(a.c,function(b,c){a.add(decodeURIComponent(b.replace(/\+/g," ")),c)}))}l=U.prototype;l.add=function(a,b){V(this);this.c=null;a=W(this,a);var c=this.a.get(a);c||this.a.set(a,c=[]);c.push(b);this.b+=1;return this};function Kc(a,b){V(a);b=W(a,b);T(a.a.b,b)&&(a.c=null,a.b-=a.a.get(b).length,a=a.a,T(a.b,b)&&(delete a.b[b],a.c--,a.a.length>2*a.c&&tc(a)))}function Lc(a,b){V(a);b=W(a,b);return T(a.a.b,b)}
|
||||
l.forEach=function(a,b){V(this);this.a.forEach(function(c,d){Ga(c,function(e){a.call(b,e,d,this)},this)},this)};l.C=function(){V(this);for(var a=this.a.D(),b=this.a.C(),c=[],d=0;d<b.length;d++)for(var e=a[d],f=0;f<e.length;f++)c.push(b[d]);return c};l.D=function(a){V(this);var b=[];if("string"===typeof a)Lc(this,a)&&(b=Ja(b,this.a.get(W(this,a))));else{a=this.a.D();for(var c=0;c<a.length;c++)b=Ja(b,a[c])}return b};
|
||||
l.set=function(a,b){V(this);this.c=null;a=W(this,a);Lc(this,a)&&(this.b-=this.a.get(a).length);this.a.set(a,[b]);this.b+=1;return this};l.get=function(a,b){if(!a)return b;a=this.D(a);return 0<a.length?String(a[0]):b};l.toString=function(){if(this.c)return this.c;if(!this.a)return"";for(var a=[],b=this.a.C(),c=0;c<b.length;c++){var d=b[c],e=encodeURIComponent(String(d));d=this.D(d);for(var f=0;f<d.length;f++){var g=e;""!==d[f]&&(g+="="+encodeURIComponent(String(d[f])));a.push(g)}}return this.c=a.join("&")};
|
||||
function Ac(a){var b=new U;b.c=a.c;a.a&&(b.a=new sc(a.a),b.b=a.b);return b}function W(a,b){b=String(b);a.g&&(b=b.toLowerCase());return b}function Hc(a,b){b&&!a.g&&(V(a),a.c=null,a.a.forEach(function(c,d){var e=d.toLowerCase();d!=e&&(Kc(this,d),Kc(this,e),0<c.length&&(this.c=null,this.a.set(W(this,e),Ka(c)),this.b+=c.length))},a));a.g=b};J.f.u={};var X="",Y="",Mc,Z,Nc=null,Oc;function Pc(){Y=X="";Nc=Z=Mc=null;y("google.load")||(D("google.load",Qc),D("google.setOnLoadCallback",J.R));var a=document.getElementsByTagName("script");a=(document.currentScript||a[a.length-1]).getAttribute("src");a=new wc(a);var b=a.a;Oc=b=b.match(/^www\.gstatic\.cn/)?"gstatic.cn":"gstatic.com";Rc(a)}
|
||||
function Rc(a){a=new U(a.c.toString());var b=a.get("callback");"string"===typeof b&&(b=Sc(b),J.f.v.aa().then(b));a=a.get("autoload");if("string"===typeof a)try{if(""!==a){var c=JSON.parse(a).modules;for(a=0;a<c.length;a++){var d=c[a];Qc(d.name,d.version,d)}}}catch(e){throw Error("Autoload failed with: "+e);}}
|
||||
function Tc(a){var b=a,c,d=a.match(/^testing-/);d&&(b=b.replace(/^testing-/,""));a=b;do{if(b===J.f.J[b])throw Error("Infinite loop in version mapping: "+b);(c=J.f.J[b])&&(b=c)}while(c);c=(d?"testing-":"")+b;return{version:"pre-45"==b?a:c,ba:c}}
|
||||
function Uc(a){var b=J.f.I.ha[Oc].loader,c=Tc(a);return J.f.o.load(b,{version:c.ba}).then(function(){var d=y("google.charts.loader.VersionSpecific.load")||y("google.charts.loader.publicLoad")||y("google.charts.versionSpecific.load");if(!d)throw Error("Bad version: "+a);Nc=function(e){e=d(c.version,e);if(null==e||null==e.then){var f=y("google.charts.loader.publicSetOnLoadCallback")||y("google.charts.versionSpecific.setOnLoadCallback");e=new Promise(function(g){f(g)});e.then=f}return e}})}
|
||||
function Vc(a){"string"===typeof a&&(a=[a]);Array.isArray(a)&&0!==a.length||(a=J.f.I.Y);var b=[];a.forEach(function(c){c=c.toLowerCase();b=b.concat(c.split(/[\s,]+\s*/))});return b}function Wc(a){a=a||"";for(var b=a.replace(/-/g,"_").toLowerCase();"string"===typeof b;)a=b,b=J.f.W[b],b===a&&(b=!1);b||(a.match(/_[^_]+$/)?(a=a.replace(/_[^_]+$/,""),a=Wc(a)):a="en");return a}
|
||||
function Xc(a){a=a||"";""!==X&&X!==a&&(console.warn(" Attempting to load version '"+a+"' of Google Charts, but the previously loaded '"+(X+"' will be used instead.")),a=X);return X=a||""}function Yc(a){a=a||"";""!==Y&&Y!==a&&(console.warn(" Attempting to load Google Charts for language '"+a+"', but the previously loaded '"+(Y+"' will be used instead.")),a=Y);"en"===a&&(a="");return Y=a||""}function Zc(a){var b={},c;for(c in a)b[c]=a[c];return b}
|
||||
function $c(a,b){b=Zc(b);b.domain=Oc;b.callback=Sc(b.callback);a=Xc(a);var c=b.language;c=Yc(Wc(c));b.language=c;if(!Mc){if(b.enableUrlSettings&&window.URLSearchParams)try{a=(new URLSearchParams(top.location.search)).get("charts-version")||a}catch(d){console.info("Failed to get charts-version from top URL",d)}Mc=Uc(a)}b.packages=Vc(b.packages);return Z=Mc.then(function(){return Nc(b)})}J.ia=function(a){return J.load(Object.assign({},a,{safeMode:!0}))};D("google.charts.safeLoad",J.ia);
|
||||
J.load=function(a){for(var b=[],c=0;c<arguments.length;++c)b[c]=arguments[c];c=0;"visualization"===b[c]&&c++;var d="current";if("string"===typeof b[c]||"number"===typeof b[c])d=String(b[c]),c++;var e={};za(b[c])&&(e=b[c]);return $c(d,e)};D("google.charts.load",J.load);J.R=function(a){if(!Z)throw Error("Must call google.charts.load before google.charts.setOnLoadCallback");return a?Z.then(a):Z};D("google.charts.setOnLoadCallback",J.R);
|
||||
var ad=H("https://maps.googleapis.com/maps/api/js?jsapiRedirect=true"),bd=H("https://maps-api-ssl.google.com/maps?jsapiRedirect=true&file=googleapi");
|
||||
function cd(a,b,c){console.warn("Loading Maps API with the jsapi loader is deprecated.");c=c||{};a=c.key||c.client;var d=c.libraries,e=function(h){for(var k={},m=0;m<h.length;m++){var p=h[m];k[p[0]]=p[1]}return k}(c.other_params?c.other_params.split("&").map(function(h){return h.split("=")}):[]),f=Object.assign({},{key:a,sa:d},e),g="2"===b?bd:ad;Z=new Promise(function(h){var k=Sc(c&&c.callback);J.f.o.load(g,{},f).then(k).then(h)})}var dd=H("https://www.gstatic.com/inputtools/js/ita/inputtools_3.js");
|
||||
function ed(a,b,c){za(c)&&c.packages?(Array.isArray(c.packages)?c.packages:[c.packages]).includes("inputtools")?(console.warn('Loading "elements" with the jsapi loader is deprecated.\nPlease load '+(dd+" directly.")),Z=new Promise(function(d){var e=Sc(c&&c.callback);J.f.o.load(dd,{},{}).then(e).then(d)})):console.error('Loading "elements" other than "inputtools" is unsupported.'):console.error("google.load of elements was invoked without specifying packages")}var fd=H("https://ajax.googleapis.com/ajax/libs/%{module}/%{version}/%{file}");
|
||||
function gd(a,b){var c;do{if(a===b[a])throw Error("Infinite loop in version mapping for version "+a);(c=b[a])&&(a=c)}while(c);return a}
|
||||
function hd(a,b,c){var d=J.f.V.$[a];if(d){b=gd(b,d.aliases);d=d.versions[b];if(!d)throw Error("Unknown version, "+b+", of "+a+".");var e={module:a,version:b||"",file:d.compressed};b=Pa(J.f.o.Z({format:fd,K:e})).toString();console.warn("Loading modules with the jsapi loader is deprecated.\nPlease load "+(a+" directly from "+b+"."));Z=new Promise(function(f){var g=Sc(c&&c.callback);J.f.o.load(fd,e).then(g).then(f)})}else setTimeout(function(){throw Error('Module "'+a+'" is not supported.');},0)}
|
||||
function Sc(a){return function(){if("function"===typeof a)a();else if("string"===typeof a&&""!==a)try{var b=y(a);if("function"!==typeof b)throw Error("Type of '"+a+"' is "+typeof b+".");b()}catch(c){throw Error("Callback of "+a+" failed with: "+c);}}}function Qc(a){for(var b=[],c=0;c<arguments.length;++c)b[c]=arguments[c];switch(b[0]){case "maps":cd.apply(null,ba(b));break;case "elements":ed.apply(null,ba(b));break;case "visualization":J.load.apply(J,ba(b));break;default:hd.apply(null,ba(b))}}
|
||||
D("google.loader.LoadFailure",!1);Oc?console.warn("Google Charts loader.js should only be loaded once."):Pc();J.f.u.ra=Pc;J.f.u.xa=Tc;J.f.u.ya=Wc;J.f.u.za=Vc;J.f.u.Ja=Xc;J.f.u.Ia=Yc;J.f.u.Aa=Rc;J.f.u.qa=function(){return Nc};}).call(this);
|
|
@ -0,0 +1,77 @@
|
|||
|
||||
<link id="theme-style" type="text/css" href="https://dayz.echosystem.fr/main.css" rel="stylesheet">
|
||||
<header>
|
||||
<!-- Nav bar -->
|
||||
<nav class="navbar navbar-inverse navbar-fixed-top">
|
||||
<div class="container">
|
||||
<div class="navbar-header">
|
||||
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
|
||||
<span class="sr-only">Toggle navigation</span>
|
||||
<span class="icon-bar"></span>
|
||||
<span class="icon-bar"></span>
|
||||
<span class="icon-bar"></span>
|
||||
<span class="icon-bar"></span>
|
||||
</button>
|
||||
<!-- <div id="navbar" class="collapse navbar-right navbar-collapse"> <a href="/"><img src="https://dayz.echosystem.fr/DayZs.png" height="50" weight="200"></a></div>-->
|
||||
<a class="navbar-brand" href="https://dayz.echosystem.fr/"><img src="https://dayz.echosystem.fr/images/DayZS.png" height="40" weight="100"><!--<img src="./images/dayz.png" height="30" weight="30">--> ☠ (-ToX-) </a>
|
||||
</div>
|
||||
|
||||
<div id="navbar" class="collapse navbar-right navbar-collapse">
|
||||
<ul class="nav navbar-nav">
|
||||
<li ><a href="https://dayz.echosystem.fr/">⛄ Home</a></li>
|
||||
|
||||
<li class=" dropdown">
|
||||
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">☠ Servers <span class="caret"></span></a>
|
||||
<ul class="dropdown-menu">
|
||||
<li ><a href="https://dayz.echosystem.fr/server/Expansion">☠ Expansion</a></li>
|
||||
<li ><a href="https://dayz.echosystem.fr/server/Namalsk">☠ Namalsk</a></li>
|
||||
<li ><a href="https://dayz.echosystem.fr/server/Livonia">☠ Livonia</a></li>
|
||||
<li ><a href="https://dayz.echosystem.fr/info.php">📢 Règles</a></li>
|
||||
</ul>
|
||||
</li>
|
||||
|
||||
|
||||
|
||||
<li ><a href="https://discord.gg/MrtVHcE6mg">🟢 Discord</a></li>
|
||||
|
||||
<li class=" dropdown">
|
||||
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">
|
||||
☠ (-TOX-) <span class=" caret"></span></a>
|
||||
<ul class="dropdown-menu">
|
||||
<li ><a href="https://dayz.echosystem.fr/Contact">👤 Contact</a></li>
|
||||
<li ><a href="https://gam3r.echosystem.fr/?Dayz">📷 Gallery</a></li>
|
||||
</ul>
|
||||
</li>
|
||||
|
||||
<li class=" dropdown">
|
||||
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false"> Tools <span class="caret"></span></a>
|
||||
<ul class="dropdown-menu">
|
||||
<li ><a href="https://steamdb.info/app/221100/graphs">Stat DAYZ Steam</a></li>
|
||||
<li ><a href="https://steamcharts.com/app/221100">DAYZ Steamcharts</a></li>
|
||||
<li ><a href="https://downdetector.com/status/steam/map">Steam Down ?</a></li>
|
||||
<li ><a href="https://steamstat.us">Steam Status</a></li>
|
||||
<li ><a href="https://dayz.gamepedia.com/DayZ_Standalone_Wiki">WIKI DAYZ</a></li>
|
||||
</ul>
|
||||
</li>
|
||||
|
||||
<li >
|
||||
|
||||
<div class="dark_" style="text-align: right;padsding-right: 10px;padding-top: 15px;color: grey;" title="Dark Mode">
|
||||
<form action="#">
|
||||
<div class="switch">
|
||||
<input id="darkTrigger" type="checkbox" class="switch-input" />
|
||||
<label for="darkTrigger" class="switch-label" ></label>
|
||||
</div>
|
||||
</form>
|
||||
</div>
|
||||
|
||||
</li>
|
||||
</ul>
|
||||
</div>
|
||||
</div>
|
||||
</nav>
|
||||
|
||||
|
||||
|
||||
|
||||
</header>
|
Binary file not shown.
After Width: | Height: | Size: 97 KiB |
|
@ -0,0 +1,849 @@
|
|||
<?php
|
||||
$page = $_SERVER['PHP_SELF'];
|
||||
$sec = "300";
|
||||
?>
|
||||
<!DOCTYPE html>
|
||||
<html lang="en">
|
||||
|
||||
<head>
|
||||
<meta http-equiv="refresh" content="<?php echo $sec?>;URL='<?php echo $page?>'">
|
||||
|
||||
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
|
||||
|
||||
<meta http-equiv="X-UA-Compatible" content="IE=edge">
|
||||
<meta name="viewport" content="width=device-width, initial-scale=1.0, shrink-to-fit=no">
|
||||
<meta name="description" content="(-ToX-) server Namalsk Dayz standalone Fr, namalsk island, Discord https://discord.gg/MrtVHcE6mg" />
|
||||
<title>(-ToX-) DAYZ Server EXPANSION Chernarus FR/EN</title>
|
||||
<meta name="author" content="Erreur32">
|
||||
<link rel="shortcut icon" type="image/x-icon" href="https://dayz.echosystem.fr/favicon.ico">
|
||||
<meta name="keyword" content="tox,server,dayz,map,namalsk,namalsk island">
|
||||
|
||||
|
||||
<link id="theme-style" href="style_dark.css" rel="stylesheet">
|
||||
|
||||
<style>
|
||||
.tab-content {
|
||||
margin-top: -1px;
|
||||
background: #3a4149;
|
||||
border: none;
|
||||
border-radius: 0 0 .25rem .25rem;
|
||||
}
|
||||
|
||||
a,
|
||||
a:visited {
|
||||
color: #20A8D8;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
a:hover {
|
||||
color: orange;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
.description-header {
|
||||
color: #26A65B;
|
||||
}
|
||||
|
||||
.label-danger {
|
||||
background-color: #EF4836;
|
||||
}
|
||||
|
||||
.label-warning {
|
||||
background-color: #F89406;
|
||||
color: white;
|
||||
}
|
||||
|
||||
.label-success {
|
||||
background-color: #26A65B;
|
||||
}
|
||||
|
||||
.label-primary {
|
||||
background-color: #4183D7;
|
||||
color: green;
|
||||
}
|
||||
|
||||
.label-info {
|
||||
background-color: #5bc0de;
|
||||
}
|
||||
|
||||
.label {
|
||||
display: inline;
|
||||
padding: .2em .6em .3em;
|
||||
font-size: 75%;
|
||||
font-weight: 700;
|
||||
line-height: 1;
|
||||
color: #fff;
|
||||
text-align: center;
|
||||
white-space: nowrap;
|
||||
vertical-align: baseline;
|
||||
border-radius: .25em;
|
||||
}
|
||||
|
||||
.selection {
|
||||
color: black !important;
|
||||
}
|
||||
|
||||
.select2-results {
|
||||
color: black !important;
|
||||
}
|
||||
|
||||
.select2-results__option--highlighted {
|
||||
background-color: #3875d7 !important;
|
||||
}
|
||||
|
||||
.pull-left {
|
||||
float: left !important;
|
||||
}
|
||||
|
||||
.pull-right {
|
||||
float: right !important;
|
||||
}
|
||||
|
||||
.navbar-brand-logo {
|
||||
margin-left: 2rem;
|
||||
}
|
||||
</style>
|
||||
|
||||
<script>
|
||||
window.dataLayer = window.dataLayer || [];
|
||||
window.mobileAndTabletcheck = function() {
|
||||
var check = false;
|
||||
(function(a){if(/(android|bb\d+|meego).+mobile|avantgo|bada\/|blackberry|blazer|compal|elaine|fennec|hiptop|iemobile|ip(hone|od)|iris|kindle|lge |maemo|midp|mmp|mobile.+firefox|netfront|opera m(ob|in)i|palm( os)?|phone|p(ixi|re)\/|plucker|pocket|psp|series(4|6)0|symbian|treo|up\.(browser|link)|vodafone|wap|windows ce|xda|xiino|android|ipad|playbook|silk/i.test(a)||/1207|6310|6590|3gso|4thp|50[1-6]i|770s|802s|a wa|abac|ac(er|oo|s\-)|ai(ko|rn)|al(av|ca|co)|amoi|an(ex|ny|yw)|aptu|ar(ch|go)|as(te|us)|attw|au(di|\-m|r |s )|avan|be(ck|ll|nq)|bi(lb|rd)|bl(ac|az)|br(e|v)w|bumb|bw\-(n|u)|c55\/|capi|ccwa|cdm\-|cell|chtm|cldc|cmd\-|co(mp|nd)|craw|da(it|ll|ng)|dbte|dc\-s|devi|dica|dmob|do(c|p)o|ds(12|\-d)|el(49|ai)|em(l2|ul)|er(ic|k0)|esl8|ez([4-7]0|os|wa|ze)|fetc|fly(\-|_)|g1 u|g560|gene|gf\-5|g\-mo|go(\.w|od)|gr(ad|un)|haie|hcit|hd\-(m|p|t)|hei\-|hi(pt|ta)|hp( i|ip)|hs\-c|ht(c(\-| |_|a|g|p|s|t)|tp)|hu(aw|tc)|i\-(20|go|ma)|i230|iac( |\-|\/)|ibro|idea|ig01|ikom|im1k|inno|ipaq|iris|ja(t|v)a|jbro|jemu|jigs|kddi|keji|kgt( |\/)|klon|kpt |kwc\-|kyo(c|k)|le(no|xi)|lg( g|\/(k|l|u)|50|54|\-[a-w])|libw|lynx|m1\-w|m3ga|m50\/|ma(te|ui|xo)|mc(01|21|ca)|m\-cr|me(rc|ri)|mi(o8|oa|ts)|mmef|mo(01|02|bi|de|do|t(\-| |o|v)|zz)|mt(50|p1|v )|mwbp|mywa|n10[0-2]|n20[2-3]|n30(0|2)|n50(0|2|5)|n7(0(0|1)|10)|ne((c|m)\-|on|tf|wf|wg|wt)|nok(6|i)|nzph|o2im|op(ti|wv)|oran|owg1|p800|pan(a|d|t)|pdxg|pg(13|\-([1-8]|c))|phil|pire|pl(ay|uc)|pn\-2|po(ck|rt|se)|prox|psio|pt\-g|qa\-a|qc(07|12|21|32|60|\-[2-7]|i\-)|qtek|r380|r600|raks|rim9|ro(ve|zo)|s55\/|sa(ge|ma|mm|ms|ny|va)|sc(01|h\-|oo|p\-)|sdk\/|se(c(\-|0|1)|47|mc|nd|ri)|sgh\-|shar|sie(\-|m)|sk\-0|sl(45|id)|sm(al|ar|b3|it|t5)|so(ft|ny)|sp(01|h\-|v\-|v )|sy(01|mb)|t2(18|50)|t6(00|10|18)|ta(gt|lk)|tcl\-|tdg\-|tel(i|m)|tim\-|t\-mo|to(pl|sh)|ts(70|m\-|m3|m5)|tx\-9|up(\.b|g1|si)|utst|v400|v750|veri|vi(rg|te)|vk(40|5[0-3]|\-v)|vm40|voda|vulc|vx(52|53|60|61|70|80|81|83|85|98)|w3c(\-| )|webc|whit|wi(g |nc|nw)|wmlb|wonu|x700|yas\-|your|zeto|zte\-/i.test(a.substr(0,4))) check = true;})(navigator.userAgent||navigator.vendor||window.opera);
|
||||
return check;
|
||||
};
|
||||
window.mobilecheck = function() {
|
||||
var check = false;
|
||||
(function(a){if(/(android|bb\d+|meego).+mobile|avantgo|bada\/|blackberry|blazer|compal|elaine|fennec|hiptop|iemobile|ip(hone|od)|iris|kindle|lge |maemo|midp|mmp|mobile.+firefox|netfront|opera m(ob|in)i|palm( os)?|phone|p(ixi|re)\/|plucker|pocket|psp|series(4|6)0|symbian|treo|up\.(browser|link)|vodafone|wap|windows ce|xda|xiino/i.test(a)||/1207|6310|6590|3gso|4thp|50[1-6]i|770s|802s|a wa|abac|ac(er|oo|s\-)|ai(ko|rn)|al(av|ca|co)|amoi|an(ex|ny|yw)|aptu|ar(ch|go)|as(te|us)|attw|au(di|\-m|r |s )|avan|be(ck|ll|nq)|bi(lb|rd)|bl(ac|az)|br(e|v)w|bumb|bw\-(n|u)|c55\/|capi|ccwa|cdm\-|cell|chtm|cldc|cmd\-|co(mp|nd)|craw|da(it|ll|ng)|dbte|dc\-s|devi|dica|dmob|do(c|p)o|ds(12|\-d)|el(49|ai)|em(l2|ul)|er(ic|k0)|esl8|ez([4-7]0|os|wa|ze)|fetc|fly(\-|_)|g1 u|g560|gene|gf\-5|g\-mo|go(\.w|od)|gr(ad|un)|haie|hcit|hd\-(m|p|t)|hei\-|hi(pt|ta)|hp( i|ip)|hs\-c|ht(c(\-| |_|a|g|p|s|t)|tp)|hu(aw|tc)|i\-(20|go|ma)|i230|iac( |\-|\/)|ibro|idea|ig01|ikom|im1k|inno|ipaq|iris|ja(t|v)a|jbro|jemu|jigs|kddi|keji|kgt( |\/)|klon|kpt |kwc\-|kyo(c|k)|le(no|xi)|lg( g|\/(k|l|u)|50|54|\-[a-w])|libw|lynx|m1\-w|m3ga|m50\/|ma(te|ui|xo)|mc(01|21|ca)|m\-cr|me(rc|ri)|mi(o8|oa|ts)|mmef|mo(01|02|bi|de|do|t(\-| |o|v)|zz)|mt(50|p1|v )|mwbp|mywa|n10[0-2]|n20[2-3]|n30(0|2)|n50(0|2|5)|n7(0(0|1)|10)|ne((c|m)\-|on|tf|wf|wg|wt)|nok(6|i)|nzph|o2im|op(ti|wv)|oran|owg1|p800|pan(a|d|t)|pdxg|pg(13|\-([1-8]|c))|phil|pire|pl(ay|uc)|pn\-2|po(ck|rt|se)|prox|psio|pt\-g|qa\-a|qc(07|12|21|32|60|\-[2-7]|i\-)|qtek|r380|r600|raks|rim9|ro(ve|zo)|s55\/|sa(ge|ma|mm|ms|ny|va)|sc(01|h\-|oo|p\-)|sdk\/|se(c(\-|0|1)|47|mc|nd|ri)|sgh\-|shar|sie(\-|m)|sk\-0|sl(45|id)|sm(al|ar|b3|it|t5)|so(ft|ny)|sp(01|h\-|v\-|v )|sy(01|mb)|t2(18|50)|t6(00|10|18)|ta(gt|lk)|tcl\-|tdg\-|tel(i|m)|tim\-|t\-mo|to(pl|sh)|ts(70|m\-|m3|m5)|tx\-9|up(\.b|g1|si)|utst|v400|v750|veri|vi(rg|te)|vk(40|5[0-3]|\-v)|vm40|voda|vulc|vx(52|53|60|61|70|80|81|83|85|98)|w3c(\-| )|webc|whit|wi(g |nc|nw)|wmlb|wonu|x700|yas\-|your|zeto|zte\-/i.test(a.substr(0,4))) check = true;})(navigator.userAgent||navigator.vendor||window.opera);
|
||||
return check;
|
||||
};
|
||||
</script>
|
||||
|
||||
|
||||
|
||||
<?php
|
||||
$time = microtime();
|
||||
$time = explode(' ', $time);
|
||||
$time = $time[1] + $time[0];
|
||||
$start = $time;
|
||||
|
||||
include_once('./consql.php');
|
||||
include_once('./config.php');
|
||||
|
||||
if (empty($Info['HostName'])) {
|
||||
$Info['HostName'] = "<h1>OFF LINE</h1>" ;
|
||||
}
|
||||
|
||||
?>
|
||||
<main class="main" style="margin-top: 10px">
|
||||
|
||||
<!-- Breadcrumb-->
|
||||
<ol class=""></ol>
|
||||
|
||||
<div class="container-fluid">
|
||||
<div id="ui-view">
|
||||
|
||||
<div class="animated fadeIn">
|
||||
<div class="row">
|
||||
|
||||
<div class="col-lg-12 col-xs-12">
|
||||
|
||||
<div class="card">
|
||||
<div class="card-body" style="text-align:center;">
|
||||
<h3> <img height="24px" width="24px"
|
||||
src="https://steamcdn-a.akamaihd.net/steamcommunity/public/images/apps/221100/f8c16699ed5ce1cc8aa9b15ed3fdd66553fce2bf.ico">
|
||||
|
||||
<?php echo $Info['HostName'] ; ?>
|
||||
<!--<i class="flag-icon h5 flag-icon-fr"></i>-->
|
||||
|
||||
</h3>
|
||||
<h5><span style="color:grey;"><?php echo " $namemap" ?> </span></h5>
|
||||
<span class="label label-<?php echo $Timer > 1.0 ? 'danger' : 'success'; ?>"><?php echo $Timer; ?>s</span>
|
||||
<br><small>(-ToX-) <?php //echo $urlserv; ?> <?php //echo count($objlower); ?></small>
|
||||
|
||||
</div>
|
||||
|
||||
<?php
|
||||
if (empty($Info['Map'])) {
|
||||
echo "</main>";
|
||||
setlocale(LC_ALL,'french');
|
||||
echo "<center><small class='text-muted'>Last time refresh</small> <br> <strong class='h4'>".date('m/d/y H:i:s')."</strong></center>";
|
||||
echo "<div style=\"padding-bottom: 10%;padding-left: 20%;padding-right: 20%\"> <center><img src=\"https://steamuserimages-a.akamaihd.net/ugc/1649972925761363590/C3DC3BB6880B659518D19ECDA9610C424794EBB8/\" class=\"arrondie2\" width=\"100%\" max-height=\"20%\" height=\"auto\"></center></div></div>";
|
||||
include('footer.php');
|
||||
exit;
|
||||
|
||||
}
|
||||
|
||||
?>
|
||||
<div class="card-footer">
|
||||
<div class="row" style="text-align:center;">
|
||||
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header">
|
||||
<?php if (empty($Info['Players'])) {
|
||||
echo "<span style='color:grey;'>"; } ?>
|
||||
<?php echo $Info['Players'] ?> <span style='color:grey;'>/<?php echo $Info['MaxPlayers'] ; ?></span></span>
|
||||
</h5>
|
||||
<span class="description-text">PLAYERS</span>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header"><?php echo $ipserv.":".$Info['GamePort']; ?>
|
||||
</h5>
|
||||
<span class="description-text">IP Server</span>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header"><?php echo $Info['Map']; ?></h5>
|
||||
<span class="description-text">MAP</span>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="">
|
||||
<h5 class="description-header">
|
||||
<?php echo $InfoGT[67].$InfoGT[68].$InfoGT[69].$InfoGT[70].$InfoGT[71]; ?> <small style="color: grey;"> <i
|
||||
class="fas fa-sun"></i><span style="color: white;">x<?php echo $InfoGT[41]; ?></span>
|
||||
- <i class="fas fa-moon"></i><span style="color: white;">x<?php echo $InfoGT[54]; ?></span>
|
||||
</small></h5>
|
||||
<span class="description-text">GAME TIME</span>
|
||||
</div>
|
||||
</div>
|
||||
<!--
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header">Yes</h5> <span
|
||||
class="description-text">3 PP</span>
|
||||
</div>
|
||||
</div>
|
||||
-->
|
||||
|
||||
|
||||
<div class="col-md-2 col-xs-12 border-right">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header"> <?php echo $Info['Version'] ; ?></h5>
|
||||
<span class="description-text">SERVER VERSION</span>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-md-2 col-xs-12">
|
||||
<div class="description-block">
|
||||
<h5 class="description-header"><?php echo $InfoGT[18].$InfoGT[19].$InfoGT[20].$InfoGT[21].$InfoGT[22].$InfoGT[23].$InfoGT[24].$InfoGT[25]; ?>
|
||||
</h5>
|
||||
<span class="description-text">HIVE</span>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
</div>
|
||||
</div><!-- class="card-footer" -->
|
||||
|
||||
</div> <!-- end card -->
|
||||
</div>
|
||||
</div>
|
||||
<!--end row -->
|
||||
|
||||
<div class="row">
|
||||
<div class="col-lg-12">
|
||||
<div class="card">
|
||||
<div class="card-body menutab">
|
||||
<ul class="nav nav-tabs" id="tabs" role="tablist">
|
||||
|
||||
<li class="nav-item">
|
||||
<a class="nav-link active show" id="overview-tab" data-toggle="tab" href="#overview" role="tab" aria-controls="overview" aria-selected="true">
|
||||
<!--<i class="fas fa-home">-->
|
||||
<i class="fas fa-chart-area"></i> Server Info</a>
|
||||
</li>
|
||||
|
||||
<li class="nav-item">
|
||||
<a class="nav-link" id="modlist-tab" data-toggle="tab" href="#modlist" role="tab" aria-controls="modlist" aria-selected="false">
|
||||
<i class="fas fa-server"></i> MOD List</a>
|
||||
</li>
|
||||
|
||||
<!--<li class="nav-item">
|
||||
<a class="nav-link" id="metrics-tab" data-toggle="tab" href="#metrics" role="tab" aria-controls="metrics" aria-selected="false">
|
||||
<i class="fas fa-chart-area"></i> Server Graph</a>
|
||||
</li>-->
|
||||
|
||||
<li class="nav-item">
|
||||
<a class="nav-link" id="userdata-tab" data-toggle="tab" href="#userdata" role="tab" aria-controls="userdata" aria-selected="false">
|
||||
<i class="fas fa-user-check"></i> User Database</a>
|
||||
</li>
|
||||
|
||||
<li class="nav-item"> <a class="nav-link" id="history-tab" data-toggle="tab" href="#historytab" role="tab" aria-controls="historytab" aria-selected="false">
|
||||
<i class="fas fa-info"></i> 🅷 🅸 🆂 🆃 🅾 🆁 🆈 ️ </a>
|
||||
</li>
|
||||
|
||||
<li class="nav-item"> <a class="nav-link" id="map-tab" data-toggle="tab" href="#maptab" role="tab" aria-controls="maptab" aria-selected="false">
|
||||
<i class="fas fa-map"></i> MAP</a>
|
||||
|
||||
</li>
|
||||
|
||||
</ul>
|
||||
|
||||
<div class="tab-content content_wrapper" id="tabs">
|
||||
|
||||
<!-- cadre stat Gameserver -->
|
||||
<div class="tab-pane fade tab_content active show" id="overview" role="tabpanel" aria-labelledby="overview-tab">
|
||||
|
||||
<div class="row" style="margin-left: 30px;">
|
||||
|
||||
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-dark" style="padding:0">
|
||||
<img src="image_ico.png" class="arrondie2" height="50px" width="150px">
|
||||
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-light">
|
||||
<small class="text-muted">Last time refresh</small> <br>
|
||||
<strong class="h4"> <?php setlocale(LC_ALL,'french'); echo date("m/d/y H:i:s"); ?></span> </strong>
|
||||
<div class="chart-wrapper">
|
||||
<canvas id="sparkline-chart-1" width="100" height="30"></canvas>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-primary">
|
||||
<small class="text-muted">Last Player actif</small> <br>
|
||||
<strong class="h4"><span style="color: #20A8D8;"><?php echo $Info['Players'] ; ?></span>
|
||||
<small> /<span style="color: grey;"><?php echo $Info['MaxPlayers'] ; ?></span></small>
|
||||
</strong>
|
||||
<div class="chart-wrapper">
|
||||
<canvas id="sparkline-chart-1" width="100" height="30"></canvas>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-warning">
|
||||
<small class="text-muted">MOD actif</small>
|
||||
<br>
|
||||
<strong class="h4"><span style="color: #FFC107;"><?php echo count($objlower); ?></span></strong>
|
||||
<div class="chart-wrapper">
|
||||
<canvas id="sparkline-chart-1" width="100" height="30"></canvas>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-success">
|
||||
<small class="text-muted">Whitelist entries</small>
|
||||
<br> <strong class="h4" style="color: #4DBD74">0</strong>
|
||||
<div class="chart-wrapper">
|
||||
<canvas id="sparkline-chart-1" width="100" height="30"></canvas>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="col-lg-2 col-sm-5">
|
||||
<div class="callout callout-danger">
|
||||
<small class="text-muted">Bans</small>
|
||||
<br> <strong class="h4" style="color: #F86C6B">0</strong>
|
||||
<div class="chart-wrapper">
|
||||
<canvas id="sparkline-chart-1" width="100" height="30"></canvas>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
</div>
|
||||
|
||||
|
||||
<div class="row" style="margin-top: 30px">
|
||||
|
||||
<div class="col-lg-10 col-sm-16">
|
||||
<div class="card">
|
||||
|
||||
<div class="card-header"><i class="fas fa-server"></i>Stat Gameserver</div>
|
||||
|
||||
<div class="card-body">
|
||||
<div class="row">
|
||||
|
||||
<div class="col-lg-14">
|
||||
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline" style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Server Name</strong>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-10" style="color:grey;">
|
||||
<?php echo $Info['HostName'] ; ?>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline"
|
||||
style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Server IP</strong>
|
||||
<br><i></i><small> Server port</small></i>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7">
|
||||
<a href="#"><?php echo $ipserv; ?></a><br>
|
||||
<a href="#"><?php echo $portserv; ?></a>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline"
|
||||
style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Total User</strong>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7">
|
||||
<i class="fa fa-user"></i>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline"
|
||||
style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Gameserver protection</strong>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7">
|
||||
<span class="badge badge-light">Anti-Cheat ON</span>
|
||||
<span class="badge badge-light">BattlEye enabled</span>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline"
|
||||
style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Best Distance Kill</strong>
|
||||
<!--br>
|
||||
<small> From User</small-->
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7"><i class="fas fa-dot-circle" style="color:#20a8d8"></i>
|
||||
800m <small> by </small> <span class="badge badge-light"> Terror_east</span>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline"
|
||||
style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Mod information</strong>
|
||||
<br><i><small> MOD Actif </small></i>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7">
|
||||
<a href="https://dayz.echosystem.fr/server/mod_namalsk2.php"><span class="badge badge-light">
|
||||
<b><?php echo count($objlower); ?></b>
|
||||
<span class="text-muted"> Mods </span></a>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="row">
|
||||
<div class="form-check form-check-inline" style="width: 100% !important;">
|
||||
<div class="col-sm-5">
|
||||
<label class="col-form-label" for="date-input">
|
||||
<strong>Direct connect</strong><br>
|
||||
<em><i> (clic to join) </i> </em>
|
||||
</label>
|
||||
</div>
|
||||
<div class="col-sm-7">
|
||||
<a style="text-decoration:none;" href='steam://connect/82.64.214.194:3201/'>
|
||||
<span class='label label-success'> GO PLAY </span>
|
||||
</a>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
</div>
|
||||
|
||||
|
||||
</div>
|
||||
</div>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
<!-- graph home page -->
|
||||
|
||||
<div class="col-lg-10 col-sm-16">
|
||||
<div class="card">
|
||||
|
||||
<?php if( !empty( $Players ) ): ?>
|
||||
<div class="card-header">List players online: <?php echo $Info['Players'] ; ?></div>
|
||||
<div class="card-body">
|
||||
|
||||
<?php foreach( $Players as $Player ): ?>
|
||||
<table>
|
||||
<tr>
|
||||
<td style="width:200px"> <span class="btn btn-large btn-primary">"Survivor" </span> </td>
|
||||
<td>  <span style='color:grey'>Playtime </span>> <?php echo $Player[ 'TimeF' ]; ?> Minutes </td>
|
||||
</tr>
|
||||
</table>
|
||||
|
||||
|
||||
|
||||
<?php endforeach; ?>
|
||||
|
||||
|
||||
</div>
|
||||
<?php else: ?>
|
||||
|
||||
<div class="card-header">List players online: <?php echo $Info['Players'] ; ?></div>
|
||||
<div class="card-body">
|
||||
<tr>
|
||||
<td colspan="2"><center><span class="btn btn-large btn-primary">( No players in game )</span></center></td> </td>
|
||||
</div>
|
||||
|
||||
|
||||
|
||||
<?php endif; ?>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<!-- graph home page -->
|
||||
|
||||
<div class="col-lg-12 col-sm-16">
|
||||
<div class="card">
|
||||
<div class="card-header">Player last 24 hours</div>
|
||||
<div class="card-body">
|
||||
<?php include('./GraphPlayer.php'); ?>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<!--
|
||||
<div class="col-lg-4 col-sm-4">
|
||||
<div class="card_">
|
||||
|
||||
</div>
|
||||
</div>
|
||||
-->
|
||||
</div>
|
||||
|
||||
</div>
|
||||
|
||||
|
||||
|
||||
<!-- TAB MOD list -->
|
||||
|
||||
<div class="tab-pane tab_content fade" id="modlist" role="tabpanel" aria-labelledby="modlist-tab">
|
||||
|
||||
<div class="row">
|
||||
<div class="col-lg-5 col-sm-12">
|
||||
|
||||
<div class="card">
|
||||
<div class="card-header"><i class="fas fa-users"></i>
|
||||
MOD list on <span style="color:grey;"><?php echo $Info['HostName'] ; ?></span>
|
||||
</div>
|
||||
|
||||
<div class="card-body">
|
||||
|
||||
<p class="before-list">
|
||||
<center>
|
||||
<span style="padding: 5px 0px 2px 20px;"><?php echo count($objlower); ?> MODS </span>
|
||||
<span style="padding: 5px 0px 2px 20px;">IP: <span style="color: orange;"><?php echo $urlserv; ?> </span></span>
|
||||
</center>
|
||||
</p>
|
||||
|
||||
<table class="table_ table-bordered_ table-striped_">
|
||||
<thead>
|
||||
<tr>
|
||||
<th style="text-align:left;"><span class='label label-info'>MOD Name</span>
|
||||
</th>
|
||||
<!-- <th><span class='label label-info'>steamWorkshopId</span></th>-->
|
||||
</tr>
|
||||
|
||||
</thead>
|
||||
|
||||
<tbody>
|
||||
<tr><td><br></td><td></td><br></tr>
|
||||
<?php $objlower=json_decode($json); //converts to an array of objects
|
||||
foreach( $objlower as $item ) { ?>
|
||||
<tr>
|
||||
<td><a href="http://steamcommunity.com/sharedfiles/filedetails/?id=<?php echo $item->steamWorkshopId?>" data-type="Link"><?php echo $item->name; ?></a></td>
|
||||
</tr>
|
||||
<?php } ?>
|
||||
|
||||
</tbody>
|
||||
|
||||
</table>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
|
||||
<!-- tab Metrics Graph -->
|
||||
|
||||
<div class="tab-pane tab_content fade" id="metrics" role="tabpanel" aria-labelledby="metrics-tab">
|
||||
|
||||
<div class="row">
|
||||
<div class="col-lg-6 col-sm-8">
|
||||
<div class="card">
|
||||
<div class="card-header">Player population 24 hours</div>
|
||||
<div class="card-body"><br>
|
||||
<!-- <iframe src="graph.php" frameborder="0" allowfullscreen scrolling="no"
|
||||
style="margin:auto;width:100%;height:395px;" security="restricted"></iframe> -->
|
||||
<?php // include('graph.php'); ?>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-lg-6 col-sm-12">
|
||||
<div class="card" style="margin: 0 auto; height: 500px; width: 100%;">
|
||||
<div class="card-header"> line-chart </div>
|
||||
<div class="card-body"><center>
|
||||
<!-- <iframe src="line-chart1.php" frameborder="0"
|
||||
allowfullscreen scrolling="no" style="overflow:hidden;width:100%;height:450px;" security="restricted"></iframe> -->
|
||||
</center>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<div class="col-lg-8 col-sm-8">
|
||||
<div class="card">
|
||||
<div class="card-header"> 12 h </div>
|
||||
<div class="card-body" style="margin: 0 auto; height: 500px; width: 100%; padding-bottom: 0;">
|
||||
<center> <script src="morris/morris.js"></script>
|
||||
</center>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
<!-- HISTORY List -->
|
||||
|
||||
<div class="tab-pane tab_content fade" id="historytab" role="tabpanel" aria-labelledby="history-tab">
|
||||
<div class="col-lg-12 col-sm-12">
|
||||
<div class="card">
|
||||
<div class="card-header" style="padding: 15px;"> <i class="fas fa-search"></i> Namalsk Island </div>
|
||||
<div style="padding:15px;">
|
||||
<img src="https://dayz.echosystem.fr/server/Namalsk/image_ico.png" alt="Namalsk island" style="width:100%;max-width:800px;max-height:450px;height:auto;" class="arrondie2 ban">
|
||||
<?php include('info.php'); ?>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
<!-- MAP tab -->
|
||||
|
||||
<div class="tab-pane tab_content fade" id="maptab" role="tabpanel" aria-labelledby="map-tab">
|
||||
<div class="col-lg-12 col-sm-12">
|
||||
<div class="card">
|
||||
<div class="card-header" style="padding: 15px;"> <i class="fas fa-map"></i> Namalsk Island </div>
|
||||
<a href="https://dayz.echosystem.fr/server/Namalsk/namalsk_map2.png">
|
||||
<!-- <img src="https://dayz.echosystem.fr/server/Namalsk/namalsk_map2.png" style="width:100%;max-width:1200px;height:auto;padding:5px;" class="arrondie2"></a>-->
|
||||
<img src="https://dayz.echosystem.fr/server/Namalsk/namalsk_map_hd.png" style="width:100%;max-width:1200px;height:auto;padding:5px;" class="arrondie2"></a>
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
<!-- tab User List -->
|
||||
<div class="tab-pane tab_content fade" id="userdata" role="tabpanel" aria-labelledby="userdata-tab">
|
||||
|
||||
<div class="col-lg-12 col-sm-12">
|
||||
<div class="card">
|
||||
<div class="card-header"> <i class="fas fa-user-check"></i> User registered </div>
|
||||
|
||||
<div style="position:relative;padding-top:56.25%;">
|
||||
<iframe src="Players" frameborder="0" allowfullscreen style="position:absolute;top:0;left:0;width:100%;height:100%;" security="restricted"></iframe>
|
||||
</div>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
<!-- end tabs -->
|
||||
|
||||
</div>
|
||||
</div>
|
||||
</div>
|
||||
</div><!-- end row -->
|
||||
</div>
|
||||
</div>
|
||||
|
||||
</div>
|
||||
</div>
|
||||
</main>
|
||||
|
||||
|
||||
|
||||
<!-- menu droite -->
|
||||
|
||||
|
||||
|
||||
<!--/div-->
|
||||
|
||||
<footer class="app-footer">
|
||||
|
||||
<div style="text-align:center;"> <span style="text-align:center;"> © 2020 (-ToX-) - <a href="https://git.echosystem.fr/Erreur32/DayZ-Stat-Server">Dayz-server-stats</a> by <a href="">Erreur32</a> </span>
|
||||
<!-- <div class="dark_" style="text-align: right;padsding-right: 10px;padding-top: 15px;color: grey;" title="Dark Mode">
|
||||
<form action="#">
|
||||
<div class="switch">
|
||||
<input id="darkTrigger" type="checkbox" class="switch-input" />
|
||||
<label for="darkTrigger" class="switch-label" ></label>
|
||||
</div>
|
||||
</form>
|
||||
</div>-->
|
||||
</div>
|
||||
|
||||
<div class="ml-auto">
|
||||
<?php
|
||||
$time = microtime();
|
||||
$time = explode(' ', $time);
|
||||
$time = $time[1] + $time[0];
|
||||
$finish = $time;
|
||||
$total_time = round(($finish - $start), 3);
|
||||
echo 'Generated in <a style="font-size: 12px" href="#" target="_blank">'.$total_time.'</a> sec.';
|
||||
?>
|
||||
</div>
|
||||
</footer>
|
||||
|
||||
<script src="./bootstrap.min.js"></script>
|
||||
<script src="./graph_loader.js"></script>
|
||||
|
||||
<!-- menu deroulant
|
||||
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script> -->
|
||||
|
||||
<script src="https://kit.fontawesome.com/9ba5e91dd6.js" crossorigin="anonymous"></script>
|
||||
|
||||
<style>
|
||||
.tab-content {
|
||||
margin-top: -1px;
|
||||
background: #3a4149;
|
||||
border: none;
|
||||
border-radius: 0 0 .25rem .25rem;
|
||||
}
|
||||
|
||||
a,
|
||||
a:visited {
|
||||
color: #20A8D8;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
a:hover {
|
||||
color: orange;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
.description-header {
|
||||
color: #26A65B;
|
||||
}
|
||||
|
||||
.arrondie2 {
|
||||
border:2px solid grey;
|
||||
-moz-border-radius:7px;
|
||||
-webkit-border-radius:7px;
|
||||
border-radius:7px;
|
||||
}
|
||||
|
||||
.switch {
|
||||
position: relative;
|
||||
display: inline-block;
|
||||
}
|
||||
.switch-input {
|
||||
display: none;
|
||||
}
|
||||
.switch-label {
|
||||
display: block;
|
||||
width: 36px;
|
||||
height: 14px;
|
||||
text-indent: -100%;
|
||||
clip: rect(0 0 0 0);
|
||||
color: transparent;
|
||||
user-select: none;
|
||||
}
|
||||
.switch-label::before,
|
||||
.switch-label::after {
|
||||
content: "";
|
||||
display: block;
|
||||
position: absolute;
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
.switch-label::before {
|
||||
width: 100%;
|
||||
height: 100%;
|
||||
background-color: #535658;
|
||||
border-radius: 9999em;
|
||||
-webkit-transition: background-color 0.25s ease;
|
||||
transition: background-color 0.25s ease;
|
||||
}
|
||||
.switch-label::after {
|
||||
top: 0;
|
||||
left: 0;
|
||||
width: 18px;
|
||||
height: 18px;
|
||||
border-radius: 50%;
|
||||
background-color: #fff;
|
||||
box-shadow: 0 0 2px rgba(0, 0, 0, 0.45);
|
||||
-webkit-transition: left 0.25s ease;
|
||||
transition: left 0.25s ease;
|
||||
}
|
||||
.switch-input:checked + .switch-label::before {
|
||||
background-color: #89c12d;
|
||||
}
|
||||
.switch-input:checked + .switch-label::after {
|
||||
left: 18px;
|
||||
}
|
||||
|
||||
</style>
|
||||
|
||||
<link rel="stylesheet" type="text/css" href="dark.css" />
|
||||
<!-- Dark Theme -->
|
||||
<script>
|
||||
$("#darkTrigger").click(function(){
|
||||
if ($("body").hasClass("dark")){
|
||||
$("body").removeClass("dark");
|
||||
}
|
||||
else{
|
||||
$("body").addClass("dark");
|
||||
}
|
||||
});
|
||||
</script>
|
||||
|
||||
|
||||
|
||||
|
||||
<!-- Global site tag (gtag.js) - Google Analytics -->
|
||||
<script async src="https://www.googletagmanager.com/gtag/js?id=G-KZ70E6176C"></script>
|
||||
<script>
|
||||
window.dataLayer = window.dataLayer || [];
|
||||
function gtag(){dataLayer.push(arguments);}
|
||||
gtag('js', new Date());
|
||||
|
||||
gtag('config', 'G-KZ70E6176C');
|
||||
</script>
|
||||
|
|
@ -0,0 +1,90 @@
|
|||
|
||||
<meta name="description" content="(-ToX-) server Namalskk Dayz standalone Fr, namalsk island , Discord https://discord.gg/MrtVHcE6mg" />
|
||||
|
||||
<div class="contents-2mQqc9" role="document" style="padding: 15px;">
|
||||
<!--
|
||||
<img src="https://dayz.echosystem.fr/server/Namalsk/image_ico.png" alt="Namalsk island" width="100%" max-height="20%" height="auto" class="arrondie2 ban">
|
||||
-->
|
||||
<br>
|
||||
<h1><span class="username-1A8OIy clickable-1bVtEA" aria-controls="popout_55" aria-expanded="false" style="color: rgb(43 173 222);" role="button" tabindex="0">
|
||||
Namalsk</span></h1>
|
||||
|
||||
<span> 🅷 🅸 🆂 🆃 🅾 🆁 🆈 ️ ❗</span>
|
||||
|
||||
<br><br>
|
||||
<strong>Namalsk</strong> est une terre gelée et sauvage.<br><br>
|
||||
🗻 elle propulse le joueur dans sur une nouvelle île dans des zones <strong>mystérieuses</strong> et<strong> sauvages</strong>, à <strong>l'ambiance oppressante</strong>.<br>
|
||||
🗺️ <em> La carte permet d'offrir une nouvelle expérience, où le joueur sentira très vite la solitude et l'inquiétude l'envahir. <br>
|
||||
🌡️ ️ Elle ajoute également la gestion de la température corporelle en permanence.</em>
|
||||
<br><br>
|
||||
❄️ <strong>Namalsk</strong> étant une région glaciale, le joueur devra trouver de quoi se réchauffer sous peine de tomber malade et d'être parcouru de tremblements de plus en plus violents compliquant singulièrement la visée.
|
||||
<br><br>
|
||||
|
||||
<h3> Pour ce faire</h3>
|
||||
✅ il faudra trouver de quoi faire <strong>un feu</strong> 🔥 , ou posséder des <strong>heat packs</strong> 🌡️ (chaufferettes).<br>
|
||||
✅ Pensez aussi à s'abriter... et 🔪 Mangez le plus possible les animaux. 🦌 <br>
|
||||
✅ De nouveaux objets et nouvelles armes 🔫 sont également de la partie.<br><br>
|
||||
|
||||
Si le joueur n'aura pas de difficultés à trouver du matériel militaire pour s'armer , <br>
|
||||
<strong> il est par contre plus difficile pour trouver de quoi se nourrir, s'abreuver 💧 et se soigner. 💉</strong><br>
|
||||
<strong> 💦 Le froid se fera de plus en plus mordant au fur-et-à-mesure que le joueur grimpera dans les hauteurs. 🌀 </strong>
|
||||
<!--
|
||||
🔥 🦌 🎄🍴🎿 ⛄ ✅ ❗🧤
|
||||
🏔️ ❄️ 🗺️ 〽️ 👹 💦 💧 🌡️ Thermometer 🧊 Ice
|
||||
-->
|
||||
<br><br><hr>
|
||||
<h2 style="color: orange;"> 💡 INFORMATION 🚨</h2>
|
||||
<br>
|
||||
<h3> 💥 Blowout</h3>
|
||||
Des sortes d'éruptions d'energie sur toute la carte qui irradient les joueurs.
|
||||
A chacun de ces orages, dont le nombre varie en fonction de la configuration des serveurs (allant de une fois par heure à une fois par jour, en passant par jamais), le joueur devra s'abriter en vitesse s'il ne tient pas à mourir.
|
||||
- En effet, cet éruption fait à la fois perdre connaissance et retire 6 000 unités de sang (sur 12 000 pour un joueur en parfaite santé).<br>
|
||||
<br>
|
||||
<h3> 👹 Les Bloodsuckers </h3>
|
||||
Des créatures avides de sang humain, rapides, et qui ont de plus la capacité de se rendre invisibles (sauf les yeux).
|
||||
La difficulté réside, outre le fait qu'il faille un grand nombre de balles pour en venir à bout (une grande quantité d'unités de sang), dans le fait que le bloodsucker ne redevient visible que lorsqu'il attaque...Si jamais cette créature vous blesse, pas de saignements ou d'infection, mais une vision troublée, des mouvements ralentis et une incapacité à tirer.<br>
|
||||
Elle court à la même vitesse que les zombies mais est en revanche capable de continuer à courir à l'intérieur des bâtiments. <br><br>
|
||||
<span style="color: red;">
|
||||
A noter qu'il est impossible d'échapper à un bloodsucker en fuyant en ligne droite ou en se réfugiant à l'intérieur d'un bâtiment, ou d'une forêt. Ils traqueront le joueur, même s'ils ne le voient plus.</span><br>
|
||||
<br>
|
||||
|
||||
<h3> 🔫 ER7 RFW</h3>
|
||||
Derrière ce nom se cache un fusil sniper electromagnétique, de science-fiction, infligeant des dégâts monstrueux qui tue instantanément la cible dans un rayon de 2 km.<br>
|
||||
Le projectile tiré n'est aucunement affecté par la gravité et touche sa cible sans lui laisser le temps de s'évader. <br>
|
||||
✅ Le ER7 RFW n'apparait que dans les bunkers de Namalsk, avec une infime chance d'effectivement de s'y trouver lors de votre visite. <br>
|
||||
les munitions sont rares et apparaissent à un endroit différent. Il ne peut y avoir qu'UN SEUL ER7 RFW à la fois sur le même serveur.<br>
|
||||
|
||||
<br><br><hr>
|
||||
<h2 style="color: red;" > 💊 Conseils de survie ! </h2>
|
||||
<br>
|
||||
- Gardez un oeil sur votre température corporelle. Elle descend plus vite qu'elle ne remonte. <br>
|
||||
- Evitez de vous engager dans les hauteurs si vous n'avez pas de quoi vous réchauffer. <br>
|
||||
- Evitez également de rester immobile, n'hésitez pas à aller vous réfugier dans un bâtiment ou un véhicule afin d'éviter d'avoir trop froid.<br>
|
||||
|
||||
<br><br>
|
||||
💥 En cas de blowout, ne trainez vraiment pas pour vous mettre à l'abri. <br>
|
||||
<u>S'évanouir au milieu d'une horde de zombies affamés n'est clairement pas la meilleure idée que vous ayez eu. <br></u>
|
||||
✅ Il est possible de se prémunir contre les blowout grâce à un appareil : l'APSI, ou Anti PSI. <br>
|
||||
Cet appareil vous avertit entre 10 à 15 secondes avant le début de l'éruption (pratique pour prévenir vos alliés qui n'en ont pas), et vous protége contre l'évanouissement (éventuellement) et la perte de sang. <br>
|
||||
|
||||
<br><br>
|
||||
👹 Les Bloodsuckers s'entendent avant de se voir. Si vous ne possédez pas de bonnes armes, ou si vous jouez seul et que votre meilleur équipement se résume à une hache, fuyez !<br>
|
||||
✅ Une fois qu'ils vous ont senti, vous n'avez que très peu de chances de vous en débarasser.<br><br>
|
||||
De même, lorsqu'ils sont invisibles, leurs yeux eux, ne le sont pas. Et bien qu'il soit très difficile de les voir de journée, gardez un oeil ouvert. A noter que ces créatures vivent dans les hauteurs.
|
||||
<br><br>
|
||||
<br> 〽️ Bonne chance et à Bientôt sur Namalsk ❄️
|
||||
|
||||
<br><br><h1>(-ToX-)</h1>
|
||||
|
||||
</div>
|
||||
|
||||
<style>
|
||||
hr {
|
||||
border: 5px solid green;
|
||||
border-radius: 5px;
|
||||
width: 40%;
|
||||
}
|
||||
|
||||
</style>
|
||||
|
||||
|
File diff suppressed because one or more lines are too long
|
@ -0,0 +1,569 @@
|
|||
/* * Dark CSS * */
|
||||
|
||||
.dark {
|
||||
background-color: #112F41;
|
||||
color: #CCCCCC;
|
||||
}
|
||||
|
||||
.dark button {
|
||||
background: #144098;
|
||||
color: #CCCCCC;
|
||||
}
|
||||
|
||||
.btn {
|
||||
color: black;
|
||||
}
|
||||
|
||||
.dark {
|
||||
/* background-color: #1B2838;
|
||||
color: #ffffff;
|
||||
*/
|
||||
background-color: #e6e6e6;
|
||||
color: #000000;
|
||||
}
|
||||
|
||||
.dark button {
|
||||
background: #144098;
|
||||
color: #e6e6e6;
|
||||
}
|
||||
|
||||
.dark button:link {
|
||||
background: #000;
|
||||
color: #e6e6e6;
|
||||
}
|
||||
|
||||
.dark button:hover{
|
||||
color:green;
|
||||
}
|
||||
|
||||
|
||||
a {
|
||||
color: green;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
a,a:visited {
|
||||
color: green;
|
||||
text-decoration: none;
|
||||
}
|
||||
a:hover {
|
||||
color: orange;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
/*CSS Table
|
||||
.div-table {
|
||||
display: table;
|
||||
border: 1px;
|
||||
}
|
||||
.div-table-row {
|
||||
display: table-row;
|
||||
height: auto;
|
||||
}
|
||||
.div-table-col {
|
||||
display: table-cell;
|
||||
padding: 5px;
|
||||
border: 1px;
|
||||
height: auto;
|
||||
}
|
||||
|
||||
.div-left {
|
||||
width: 30%;
|
||||
}
|
||||
.div-right {
|
||||
width: 70%;
|
||||
}
|
||||
|
||||
.error {
|
||||
color: red;
|
||||
text-decoration: none;
|
||||
font-weight: bold;
|
||||
}
|
||||
|
||||
.underline {
|
||||
text-decoration: underline;
|
||||
}
|
||||
*/
|
||||
/* main */
|
||||
|
||||
body{
|
||||
background-color: #112F41;
|
||||
color: #CCCCCC;
|
||||
-webkit-font-smoothing: subpixel-antialiased;
|
||||
-webkit-text-stroke:1px transparent;
|
||||
padding: 1rem;
|
||||
}
|
||||
|
||||
/*
|
||||
.main {
|
||||
background-color: #112F41;
|
||||
color: #CCCCCC;
|
||||
|
||||
}
|
||||
|
||||
*/
|
||||
|
||||
.label-danger {
|
||||
background-color: #EF4836;
|
||||
}
|
||||
.label-warning {
|
||||
background-color: #F89406;
|
||||
color: white;
|
||||
}
|
||||
.label-success {
|
||||
background-color: #26A65B;
|
||||
}
|
||||
.label-primary {
|
||||
background-color: #4183D7;
|
||||
color: green;
|
||||
}
|
||||
|
||||
|
||||
.alert-success {
|
||||
color: #26A65B;
|
||||
background-color: rgba(38, 166, 91, 0.1);
|
||||
border-color: #26A65B;
|
||||
}
|
||||
.alert-info {
|
||||
color: #4183D7;
|
||||
background-color: rgba(65, 131, 215, 0.1);
|
||||
border-color: #4183D7;
|
||||
}
|
||||
.alert-warning {
|
||||
color: #F89406;
|
||||
background-color: rgba(248, 148, 6, 0.1);
|
||||
border-color: #F89406;
|
||||
}
|
||||
.alert-danger {
|
||||
color: #EF4836;
|
||||
background-color: rgba(239, 72, 54, 0.1);
|
||||
border-color: #EF4836;
|
||||
}
|
||||
|
||||
|
||||
|
||||
.btn{
|
||||
border-radius: 2px;
|
||||
}
|
||||
|
||||
.btn-default {
|
||||
color: #333;
|
||||
background-color: rgba(51, 51, 51, 0.1);
|
||||
border-color: #333;
|
||||
-webkit-transition: background-color .1s, color .1s;
|
||||
transition: background-color .1s, color .1s;
|
||||
outline: 0 !important;
|
||||
}
|
||||
.btn-default:hover,
|
||||
.btn-default:focus {
|
||||
color: #333;
|
||||
background-color: rgba(51, 51, 51, 0.2) !important;
|
||||
border-color: #333;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-default:active {
|
||||
color: white !important;
|
||||
background-color: rgb(51, 51, 51) !important;
|
||||
border-color: #333 !important;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-primary {
|
||||
color: #4183D7;
|
||||
background-color: rgba(65, 131, 215, 0.1);
|
||||
border-color: #4183D7;
|
||||
-webkit-transition: background-color .1s, color .1s;
|
||||
transition: background-color .1s, color .1s;
|
||||
outline: 0 !important;
|
||||
}
|
||||
.btn-primary:hover,
|
||||
.btn-primary:focus {
|
||||
color: #4183D7 !important;
|
||||
background-color: rgba(65, 131, 215, 0.2);
|
||||
border-color: #4183D7;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-primary:active {
|
||||
color: white !important;
|
||||
background-color: rgb(65, 131, 215) !important;
|
||||
border-color: #4183D7 !important;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-success {
|
||||
color: #26A65B;
|
||||
background-color: rgba(38, 166, 91, 0.1);
|
||||
border-color: #26A65B;
|
||||
-webkit-transition: background-color .1s, color .1s;
|
||||
transition: background-color .1s, color .1s;
|
||||
outline: 0 !important;
|
||||
}
|
||||
.btn-success:hover,
|
||||
.btn-success:focus {
|
||||
color: #26A65B !important;
|
||||
background-color: rgba(38, 166, 91, 0.2);
|
||||
border-color: #26A65B;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-success:active {
|
||||
color: white !important;
|
||||
background-color: rgb(38, 166, 91) !important;
|
||||
border-color: #26A65B !important;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-warning {
|
||||
color: #F89406;
|
||||
background-color: rgba(248, 148, 6, 0.1);
|
||||
border-color: #F89406;
|
||||
-webkit-transition: background-color .1s, color .1s;
|
||||
transition: background-color .1s, color .1s;
|
||||
outline: 0 !important;
|
||||
}
|
||||
.btn-warning:hover,
|
||||
.btn-warning:focus {
|
||||
color: #F89406 !important;
|
||||
background-color: rgba(248, 148, 6, 0.2);
|
||||
border-color: #F89406;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-warning:active {
|
||||
color: white !important;
|
||||
background-color: rgb(248, 148, 6) !important;
|
||||
border-color: #F89406 !important;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-danger {
|
||||
color: #EF4836;
|
||||
background-color: rgba(239, 72, 54, 0.1);
|
||||
border-color: #EF4836;
|
||||
-webkit-transition: background-color .1s, color .1s;
|
||||
transition: background-color .1s, color .1s;
|
||||
outline: 0 !important;
|
||||
}
|
||||
.btn-danger:hover,
|
||||
.btn-danger:focus {
|
||||
color: #EF4836 !important;
|
||||
background-color: rgba(239, 72, 54, 0.2);
|
||||
border-color: #EF4836;
|
||||
box-shadow: none;
|
||||
}
|
||||
.btn-danger:active {
|
||||
color: white !important;
|
||||
background-color: rgb(239, 72, 54) !important;
|
||||
border-color: #EF4836 !important;
|
||||
box-shadow: none;
|
||||
}
|
||||
|
||||
.btn-update > i.fa{
|
||||
width:14px;
|
||||
}
|
||||
.no_connection{
|
||||
display: none;
|
||||
}
|
||||
.hidden{
|
||||
display: none;
|
||||
}
|
||||
.info-ip{cursor:pointer;}
|
||||
|
||||
a.btn.pull-right{margin-top: -6px;}
|
||||
|
||||
|
||||
|
||||
/* preso */
|
||||
|
||||
.navbar-brand{
|
||||
padding-top: 8px;
|
||||
font-weight: 200;
|
||||
font-size: 25px;
|
||||
/* color: #F22613 !important;*/
|
||||
text-shadow: none;
|
||||
-webkit-transition: text-shadow .3s;
|
||||
transition: text-shadow .3s;
|
||||
}
|
||||
.navbar-brand:hover{
|
||||
text-shadow: 0px 0px 15px #F22613;
|
||||
-webkit-transition: text-shadow .3s;
|
||||
transition: text-shadow .3s;
|
||||
}
|
||||
|
||||
.dropdown-menu>li>a:focus, .dropdown-menu>li>a:hover {
|
||||
color: #b8b8b8;
|
||||
text-decoration: none;
|
||||
background-color: #333;
|
||||
}
|
||||
|
||||
.navbar-inverse{
|
||||
background-color: #333;
|
||||
height: 50px;
|
||||
}
|
||||
.navbar-inverse .navbar-nav>.active>a, .navbar-inverse .navbar-nav>.active>a:focus, .navbar-inverse .navbar-nav>.active>a:hover {
|
||||
background-color: transparent;
|
||||
}
|
||||
.navbar-inverse .navbar-nav>.open>a, .navbar-inverse .navbar-nav>.open>a:focus, .navbar-inverse .navbar-nav>.open>a:hover {
|
||||
background-color: transparent;
|
||||
}
|
||||
|
||||
.navbar-search{
|
||||
cursor: pointer;
|
||||
}
|
||||
.navbar-brand img {
|
||||
/* width: 33px;*/
|
||||
display: inline;
|
||||
/* margin-right: 10px;
|
||||
margin-top: 2px; */
|
||||
margin-left: auto;
|
||||
margin-right: auto;
|
||||
margin-top: -4px;
|
||||
margin-bottom: 0px;
|
||||
}
|
||||
.navbar-nav{
|
||||
background-color: #333;
|
||||
margin-top: -1px;
|
||||
}
|
||||
.dropdown-menu li.active>a{
|
||||
background-color: #333;
|
||||
}
|
||||
.dropdown-menu li.active>a:hover{
|
||||
background-color: #333;
|
||||
}
|
||||
.navbar-form input{
|
||||
background-color: #595959;
|
||||
color: #9d9d9d;
|
||||
-webkit-transition: background-color .2s;
|
||||
transition: background-color .2s;
|
||||
}
|
||||
.navbar-form input:focus{
|
||||
background-color: white;
|
||||
}
|
||||
|
||||
.container-wrapper_{
|
||||
width: 100%;
|
||||
padding-top: 30px;
|
||||
padding-bottom: 20px;
|
||||
/* background-color: #f0f0f0;*/
|
||||
}
|
||||
select.form-control{
|
||||
-webkit-appearance: none;
|
||||
-moz-appearance: none;
|
||||
background-position: right 50%;
|
||||
background-repeat: no-repeat;
|
||||
background-image: url(/image/arrow.svg);
|
||||
}
|
||||
.form-control {
|
||||
-webkit-box-shadow: none;
|
||||
box-shadow: none;
|
||||
|
||||
border-radius: 2px;
|
||||
color: #333;
|
||||
background-color: rgba(51, 51, 51, 0.05);
|
||||
border-color: #333;
|
||||
}
|
||||
.form-control:focus {
|
||||
color: #333;
|
||||
background-color: rgba(51, 51, 51, 0.1);
|
||||
border-color: #333;
|
||||
|
||||
outline: 0;
|
||||
-webkit-box-shadow: none;
|
||||
box-shadow: none;
|
||||
}
|
||||
|
||||
|
||||
|
||||
.switch {
|
||||
position: relative;
|
||||
display: inline-block;
|
||||
}
|
||||
.switch-input {
|
||||
display: none;
|
||||
}
|
||||
.switch-label {
|
||||
display: block;
|
||||
width: 36px;
|
||||
height: 14px;
|
||||
text-indent: -100%;
|
||||
clip: rect(0 0 0 0);
|
||||
color: transparent;
|
||||
user-select: none;
|
||||
}
|
||||
.switch-label::before,
|
||||
.switch-label::after {
|
||||
content: "";
|
||||
display: block;
|
||||
position: absolute;
|
||||
cursor: pointer;
|
||||
}
|
||||
|
||||
.switch-label::before {
|
||||
width: 100%;
|
||||
height: 100%;
|
||||
background-color: #535658;
|
||||
border-radius: 9999em;
|
||||
-webkit-transition: background-color 0.25s ease;
|
||||
transition: background-color 0.25s ease;
|
||||
}
|
||||
.switch-label::after {
|
||||
top: 0;
|
||||
left: 0;
|
||||
width: 18px;
|
||||
height: 18px;
|
||||
border-radius: 50%;
|
||||
background-color: #fff;
|
||||
box-shadow: 0 0 2px rgba(0, 0, 0, 0.45);
|
||||
-webkit-transition: left 0.25s ease;
|
||||
transition: left 0.25s ease;
|
||||
}
|
||||
.switch-input:checked + .switch-label::before {
|
||||
background-color: #89c12d;
|
||||
}
|
||||
.switch-input:checked + .switch-label::after {
|
||||
left: 18px;
|
||||
}
|
||||
|
||||
|
||||
|
||||
/* dark theme
|
||||
[data-theme="dark"] {
|
||||
background-color: #FFF !important;
|
||||
color: #eee;
|
||||
}
|
||||
[data-theme="dark"] .bg-light {
|
||||
background-color: #333 !important;
|
||||
}
|
||||
[data-theme="dark"] .bg-white {
|
||||
background-color: #000 !important;
|
||||
}
|
||||
[data-theme="dark"] .bg-black {
|
||||
background-color: #FFF !important;
|
||||
}
|
||||
*/
|
||||
img.bg {
|
||||
/* Set rules to fill background */
|
||||
min-height: 50px;
|
||||
min-width: 100%;
|
||||
|
||||
/* Set up proportionate scaling */
|
||||
width: 100%;
|
||||
height: auto;
|
||||
|
||||
/* Set up positioning */
|
||||
position: fixed;
|
||||
top: 0;
|
||||
left: 0;
|
||||
}
|
||||
|
||||
#nav_ img {
|
||||
position: left;
|
||||
top: 0px;
|
||||
left: 0px;
|
||||
}
|
||||
|
||||
hr {
|
||||
border: 5px solid green;
|
||||
border-radius: 5px;
|
||||
width: 40%;
|
||||
}
|
||||
|
||||
|
||||
.card {
|
||||
/* padding: 10rem;*/
|
||||
/*height: 16rem;*/
|
||||
border: #333;
|
||||
border-radius: 15%;
|
||||
}
|
||||
.cards {
|
||||
max-width: 1200px;
|
||||
margin: 0 auto;
|
||||
display: grid;
|
||||
grid-gap: 4rem;
|
||||
|
||||
}
|
||||
|
||||
|
||||
@media (min-width: 990px) {
|
||||
.navbar-form input{
|
||||
width: 188px !important;
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
/* Screen larger than 600px? 2 column */
|
||||
@media (min-width: 660px) {
|
||||
.cards { grid-template-columns: repeat(2, 1fr); }
|
||||
}
|
||||
|
||||
/* Screen larger than 900px? 3 columns */
|
||||
@media (min-width: 900px) {
|
||||
.cards { grid-template-columns: repeat(3, 1fr); }
|
||||
}
|
||||
|
||||
/*
|
||||
@media (min-width: 1200px) {
|
||||
.cards { grid-template-columns: repeat(4, 1fr); }
|
||||
}
|
||||
*/
|
||||
|
||||
h1 {
|
||||
padding: 20px 20px 0 20px;
|
||||
font-weight: 200;
|
||||
font-size: 3em;
|
||||
margin: 0;
|
||||
}
|
||||
|
||||
em {
|
||||
font-variant: italic;
|
||||
color:silver;
|
||||
}
|
||||
|
||||
.arrondie2 {
|
||||
border:2px solid grey;
|
||||
-moz-border-radius:7px;
|
||||
-webkit-border-radius:7px;
|
||||
border-radius:7px;
|
||||
}
|
||||
|
||||
.arrondie {
|
||||
-moz-border-radius: 7px;
|
||||
-webkit-border-radius: 7px;
|
||||
border-radius: 7px;
|
||||
}
|
||||
|
||||
|
||||
/* footer css */
|
||||
|
||||
.footer {
|
||||
background-color: #333;
|
||||
color: grey;
|
||||
/* position: relative;*/
|
||||
/*position: absolute;*/
|
||||
/* width: 100%; */
|
||||
}
|
||||
|
||||
footer a.footerd, footer a.footerd:visited {
|
||||
color: green;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
a.footerd:hover {
|
||||
color: rgb(190, 115, 17);
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
a.footerd, a.footerd:visited {
|
||||
color: green;
|
||||
text-decoration: none;
|
||||
}
|
||||
|
||||
footer {
|
||||
background-color: #333;
|
||||
color: grey;
|
||||
/* position: relative;*/
|
||||
/*position: absolute;
|
||||
position: -webkit-sticky;
|
||||
position: sticky;*/
|
||||
position: fixed;
|
||||
bottom: 0;
|
||||
left: 0px;
|
||||
right: 0px;
|
||||
text-align: center;
|
||||
padding-bottom: 5px;
|
||||
padding-top: 5px;
|
||||
}
|
|
@ -0,0 +1,2 @@
|
|||
.morris-hover{position:absolute;z-index:1000}.morris-hover.morris-default-style{border-radius:10px;padding:6px;color:#666;background:rgba(255,255,255,0.8);border:solid 2px rgba(230,230,230,0.8);font-family:sans-serif;font-size:12px;text-align:center}.morris-hover.morris-default-style .morris-hover-row-label{font-weight:bold;margin:0.25em 0}
|
||||
.morris-hover.morris-default-style .morris-hover-point{white-space:nowrap;margin:0.1em 0}
|
File diff suppressed because it is too large
Load Diff
Binary file not shown.
After Width: | Height: | Size: 376 KiB |
Binary file not shown.
After Width: | Height: | Size: 4.2 MiB |
File diff suppressed because one or more lines are too long
Loading…
Reference in New Issue